START OF PLAY The player starts with 100 points. They have ten attempts (or rounds) to reach 500 points to win. Each round, the player "bets" some amount of points. See gameplay as follows: A HIGH/LOW ROUND (1) The player is shown an initial random card. Cards have a value from 2-14; however, these values are converted to a string as follows: 2-9 are converted to their string equivalent "2", "3", ... "9" 10 is "T", 11 is "J", 12 is "Q", 13 is "K" and 14 is "A" (2) After the play sees the first card, they should guess if the next card will be higher (i.e., greater than the current card) or lower (i.e., a lower value than the first card). They will do this by inputting "H" or "L" (lowercase "h" and "l" will also be accepted as valid input). (3) After they input their "high" or "low" guess, the player bets a number of points that their guess will be right. The bet must be between 1 and the total amount of points the player has.1 (4) After the bet amount is entered, a second card will be randomly generated. If the player was right about their guess (high or low) they win the bet amount; otherwise, they lose the amount the bet amount. The bet amount will be added or deducted from their overall points. Question: What if the second card is the same value as the first? You still lose because it isn't higher or lower. (5) The game keeps going until the player points go to 0 (i.e., they lose all their points) or the player wins 500 points or more. If the player cannot get to 500 points within ten (10) rounds, they also lose. STOPPING CRITERIA (1) [WIN] If the player reaches 500 or more points, stop and let them know how many rounds it took them. (2) [LOSE] If the player runs out of points (i.e., points is 0), stop the game and let them know what round they ran out of points. (3) [LOSE] If they have not reached 500 or more points after ten rounds, let them know the final number of points they have and that they made it to 10 rounds. 2. Implementation Details As mentioned in Section 1, you need to implement specified functions five (5) functions. Note – you can have more functions, but you should have these five (5) implemented as specified. getCardValue() This function will return a random number between 2-14. It takes no parameters. getCardStr(cardValue) Parameter cardValue is an integer between 2-14. This function will convert the integer to a string as follows. Integers 2 to 9 are converted to "2" .. "9" 10 to "T", 11 to "J", 12 to "Q", 13 to "K", and 14 to "A" Return: The function returns a string. getHLGuess() This function will repeatedly ask the player "High or Low (H/L)?:" The function should repeat get input until either a "H", "h", "L" or "l" is entered. Return: Depending on what is entered, return the string "HIGH" or the string "LOW". NOTE – you don't return "H" or "L", the function should return the string "HIGH" or "LOW" getBetAmount(maximum) Parameter maximum is an integer that represents the maximum a player can bet. This maximum should be the player's current number of points they have. This function will repeatedly ask the player "Input bet amount: " Check to make sure the amount is between 1 and maximum. If the amount is less than 0 or over the maximum, ask the player to enter again. Return: This function should return the amount the user entered. playerGuessCorrect(card1, card2, betType) Parameters card1 and card2 represent the integer values of the two cards. Parameter betType is a string that is either "HIGH" or "LOW". Depending on the betType, see if the player was correct. For example, if the betType was "HIGH" and Card1 > Card2, then the user was incorrect (False). Think carefully about all the cases for the player to be right or wrong. Return: return True or False (Boolean) depending on if the guess was right. Remember, if both cards are equal, the guess is wrong (False)
START OF PLAY
The player starts with 100 points. They have ten attempts (or rounds) to reach 500 points to win. Each round, the player "bets" some amount of points. See gameplay as follows:
A HIGH/LOW ROUND
(1) The player is shown an initial random card.
Cards have a value from 2-14; however, these values are converted to a string as follows: 2-9 are converted to their string equivalent "2", "3", ... "9"
10 is "T", 11 is "J", 12 is "Q", 13 is "K" and 14 is "A"
(2) After the play sees the first card, they should guess if the next card will be higher (i.e., greater than the current card) or lower (i.e., a lower value than the first card).
They will do this by inputting "H" or "L" (lowercase "h" and "l" will also be accepted as valid input).
(3) After they input their "high" or "low" guess, the player bets a number of points that their guess will be right. The bet must be between 1 and the total amount of points the player has.1
(4) After the bet amount is entered, a second card will be randomly generated. If the player was right about their guess (high or low) they win the bet amount; otherwise, they lose the amount the bet amount.
The bet amount will be added or deducted from their overall points.
Question: What if the second card is the same value as the first? You still lose because it isn't higher or lower.
(5) The game keeps going until the player points go to 0 (i.e., they lose all their points) or the player wins 500 points or more. If the player cannot get to 500 points within ten (10) rounds, they also lose.
STOPPING CRITERIA
(1) [WIN] If the player reaches 500 or more points, stop and let them know how many rounds it took them.
(2) [LOSE] If the player runs out of points (i.e., points is 0), stop the game and let them know what round they ran out of points.
(3) [LOSE] If they have not reached 500 or more points after ten rounds, let them know the final number of points they have and that they made it to 10 rounds.
2. Implementation Details
As mentioned in Section 1, you need to implement specified functions five (5) functions.
Note – you can have more functions, but you should have these five (5) implemented as specified.
getCardValue()
This function will return a random number between 2-14.
getCardStr(cardValue)
Parameter cardValue is an integer between 2-14.
This function will convert the integer to a string as follows. Integers 2 to 9 are converted to "2" .. "9"
10 to "T", 11 to "J", 12 to "Q", 13 to "K", and 14 to "A" Return: The function returns a string.
getHLGuess()
This function will repeatedly ask the player "High or Low (H/L)?:"
The function should repeat get input until either a "H", "h", "L" or "l" is entered. Return: Depending on what is entered, return the string "HIGH" or the string "LOW".
NOTE – you don't return "H" or "L", the function should return the string "HIGH" or "LOW"
getBetAmount(maximum)
Parameter maximum is an integer that represents the maximum a player can bet.
This maximum should be the player's current number of points they have.
This function will repeatedly ask the player "Input bet amount: "
Check to make sure the amount is between 1 and maximum. If the amount is less than 0 or over the maximum, ask the player to enter again.
Return: This function should return the amount the user entered.
playerGuessCorrect(card1, card2, betType)
Parameters card1 and card2 represent the integer values of the two cards.
Parameter betType is a string that is either "HIGH" or "LOW".
Depending on the betType, see if the player was correct.
For example, if the betType was "HIGH" and Card1 > Card2, then the user was incorrect (False). Think carefully about all the cases for the player to be right or wrong. Return: return True or False (Boolean) depending on if the guess was right.
Remember, if both cards are equal, the guess is wrong (False)
![EXAMPLE 1 - User input in red (WIN)
--- Welcome to High-Low ---
Start with 100 points. Each round a card will be drawn and shown.
Select whether you think the 2nd card will be Higher or Lower than the 1st card.
Then enter the amount you want to bet.
If you are right, you win the amount you bet, otherwise you lose.
Try to make it to 500 points within 10 tries.
First card is shown.
OVERALL POINTS: 100
ROUND 1/10
Player selects "high" or "low"
First card is a [T]
High or Low (H/L)?: 1
Input bet amount: 50
Second card is a [5]
Cardi [T] Card 2 [5] - You bet 'LOW' for 50
Player enters in an amount between 1
and their current overall points.
YOU WON
Second card is shown.
OVERALL POINTS: 150 ROUND 2/10
First card is a [Q]
High or Low (H/L)?: 1
Input bet amount: 140
Second card is a [6]
Card1 [Q] Card 2 [6] - You bet 'LOW' for 140 - YOU WON
If player was correct, they win the
amount they bet. The amount is
added to their overall points. (In this
round the play won 50 points. Notice
in round 2 the overall points is now
150).
Start each round showing current
overall points and what round it is.
OVERALL POINTS: 290
ROUND 3/10
First card is a [9]
High or Low (H/L)?: 1
Input bet amount: 90
Second card is a [4]
Card1 [9] Card 2 [4] - You bet 'LOW' for 90 - YOU WON
OVERALL POINTS: 380 ROUND 4/10
First card is a [4]
High or Low (H/L)?: h
Input bet amount: 200
Second card is a [7]
Card1 [4] Card 2 [7] - You bet 'HIGH' for 200
YOU WON
If 500 or more points are reached,
game stops and winning message is
displayed as shown.
------WIN--
YOU MADE IT TO *580* POINTS IN 4 ROUNDS!](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F842ddfb5-3cba-4856-a7b3-78a95dca4dd5%2Fbc5df005-6dfd-46f0-afa1-d233baf48c05%2Fhdbdwtb_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

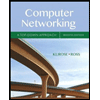
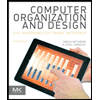
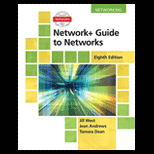
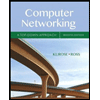
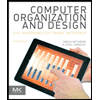
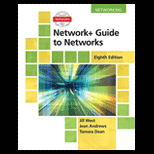
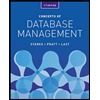
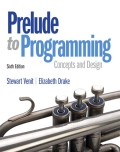
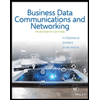