Step 1. Call by Values - Swap Function 1. Declare a function named swap on the top of your main to accept two int variables and returns void. 2. Write your main function as follow: int main() ( int x = 2; int y = 14; printf("Before swap method: x=%d, y=%d.\n", x,y); swap(x,y); printf("After swap method: x=%d, y=%d.\n", x,y); return 0; } 3. Develop swap function to swap two variables. void swap (int a, int b) ( //write a code to swap a and b. printf("In swap function a=%d, b=%d.\n", a,b); return; Output sample: Before swap method: x=14, y=2. In swap function a=14, b=2. After swap method: x=2, y=14. Step 2. Call by Reference. As you saw in step 1, to effectively exchange the values of two variables we can't use a function as call by values. 1. Change the function declaration at the top of the main functon to accept two pointers to int. 2. Change the swap function header to accept two pointers to int as a parameter list. 3. Change the swap function's body to work on two pointers to exchange the values of two variables.
Step 1. Call by Values - Swap Function 1. Declare a function named swap on the top of your main to accept two int variables and returns void. 2. Write your main function as follow: int main() ( int x = 2; int y = 14; printf("Before swap method: x=%d, y=%d.\n", x,y); swap(x,y); printf("After swap method: x=%d, y=%d.\n", x,y); return 0; } 3. Develop swap function to swap two variables. void swap (int a, int b) ( //write a code to swap a and b. printf("In swap function a=%d, b=%d.\n", a,b); return; Output sample: Before swap method: x=14, y=2. In swap function a=14, b=2. After swap method: x=2, y=14. Step 2. Call by Reference. As you saw in step 1, to effectively exchange the values of two variables we can't use a function as call by values. 1. Change the function declaration at the top of the main functon to accept two pointers to int. 2. Change the swap function header to accept two pointers to int as a parameter list. 3. Change the swap function's body to work on two pointers to exchange the values of two variables.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter10: Pointers
Section: Chapter Questions
Problem 2PP
Related questions
Question
100%
Hello. Please answer the attached C
*If correctly fulfill and do all of the steps correctly, I will give you a thumbs up. Thanks.

Transcribed Image Text:Step 1. Call by Values - Swap Function
1. Declare a function named swap on the top of your main to accept two int
variables and returns void.
2. Write your main function as follow:
int main()
{
int x = 2;
int y = 14;
printf ("Before swap method: x=%d, y=%d.\n", x,y);
swap(x,y);
printf("After swap method: x=%d, y=%d.\n", x,y);
return 0;
}
3. Develop swap function to swap two variables.
}
void swap (int a, int b)
{
//write a code to swap a and b.
printf("In swap function a=%d, b=%d.\n", a,b);
return;
Output sample:
Before swap method: x=14, y=2.
In swap function a=14, b=2.
After swap method: x=2, y=14.
Step 2. Call by Reference.
As you saw in step 1, to effectively exchange the values of two variables we can't use a function
as call by values.
1. Change the function declaration at the top of the main functon to accept two pointers
to int.
2. Change the swap function header to accept two pointers to int as a parameter list.
3. Change the swap function's body to work on two pointers to exchange the values of two
variables.

Transcribed Image Text:4. Chane the function call in the main function to call the new swap.
void swap (- --------) ;
int main()
{
}
int x = 2;
int y = 14;
printf("Before swap method: x=%d, y=%d.\n", x,y);
//Call swap function.
printf("After swap method: x=%d, y=%d.\n", x,y);
return 0;
void swap
{
//Write the code exchange the values of the parameter
printf("In swap function a=%d, b=%d.\n", ---------);
return;
//values
}
Output sample:
Before swap method: x=2, y=14.
In swap function a=14, b=2.
After swap method: x=14, y=2.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
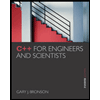
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
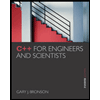
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr