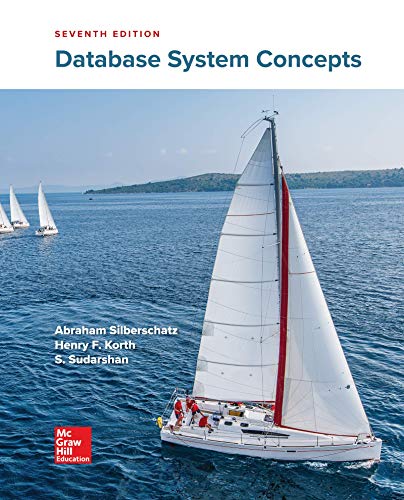
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
You need to implement a class for Dequeue i.e. for double ended queue. In this queue, elements can be inserted and deleted from both the ends. | |
You don't need to double the capacity. | |
You need to implement the following functions - | |
1. constructor | |
You need to create the appropriate constructor. Size for the queue passed is 10. | |
2. insertFront - | |
This function takes an element as input and insert the element at the front of queue. Insert the element only if queue is not full. And if queue is full, print -1 and return. | |
3. insertRear - | |
This function takes an element as input and insert the element at the end of queue. Insert the element only if queue is not full. And if queue is full, print -1 and return. | |
4. deleteFront - | |
This function removes an element from the front of queue. Print -1 if queue is empty. | |
5. deleteRear - | |
This function removes an element from the end of queue. Print -1 if queue is empty. | |
6. getFront - | |
Returns the element which is at front of the queue. Return -1 if queue is empty. | |
7. getRear - | |
Returns the element which is at end of the queue. Return -1 if queue is empty. | |
Input Format: | |
For C++ and Java, input is already managed for you. You just have to implement given functions. | |
For Python: | |
The choice codes and corresponding input for each choice are given in a new line. The input is terminated by integer -1. The following table elaborately describes the function, their choice codes and their corresponding input - | |
Alt Text | |
Output Format: | |
For C++ and Java, output is already managed for you. You just have to implement given functions. | |
For Python: | |
The output format for each function has been mentioned in the problem description itself. | |
Sample Input 1: | |
5 1 49 1 64 2 99 5 6 -1 | |
Sample Output 1: | |
-1 | |
64 | |
99 | |
Explanation: | |
The first choice code corresponds to getFront. Since the queue is empty, hence the output is -1. | |
The following input adds 49 at the top and the resultant queue becomes: 49. | |
The following input adds 64 at the top and the resultant queue becomes: 64 -> 49 | |
The following input add 99 at the end and the resultant queue becomes: 64 -> 49 -> 99 | |
The following input corresponds to getFront. Hence the output is 64. | |
The following input corresponds to getRear. Hence the output is 99..
|
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In C++, – Implement a Priority queue using a SORTED list. Use Quick sort after adding a new node. Create a class called Node: Have a Name and Priority.Data set - 1 is the highest priority, 10 is lowest priority.Enqueue and dequeue in the following order.Function Name, PriorityEnqueue Joe, 3Enqueue Fred, 1Enqueue Tuyet, 9Enqueue Jose, 6DequeueEnqueue Jing, 2Enqueue Xi, 5Enqueue Moe, 3DequeueEnqueue Miko, 7Enqueue Vlady, 8Enqueue Frank, 9Enqueue Anny, 3DequeueEnqueue Xi, 2Enqueue Wali, 2Enqueue Laschec, 6Enqueue Xerrax, 8DequeueDequeueDequeueDequeueDequeueDequeueDequeueDequeueDequeueDequeueDequeuearrow_forwardFor the Assignment 5 (Part 2), Routes v.2 at the end of this module you will create a Route class that has a member a "bag" of Leg objects. The bag is to use a vector of pointers, but since all the objects in the bag are to be Leg s, it's okay to use Leg* instead of void* in the bag's declaration. You wrote its first constructor in a previous exercise. Now write a second one. (You may refer to the Assignment page to refer to the completed class declarations of Leg and Route classes). Assume that class Leg has data member C strings const char* const beginCity; and const char* const endCity; that are available to Route by a friend relationship. Write a constructor function for a Route class that takes exactly two parameters: a constant Route object reference to an already-existing Route , and a constant Leg object reference to be appended to the end of that Route to form the new Route . Here's the algorithm: Copy the first parameter's bag into the host object's bag. If the endCity of…arrow_forwardAssume that, you have a Linked List class "LL" with a function named "fun1". You can assume that "value" is the value of the nodes and "Next" is the next pointer of the node. You created an instance of the LL class, named "L1", where "head" is the head node. And you inserted the following numbers: 31 -> 32 -> 43 -> 17 -> 34 -> 57 ->91. What will be the output of the linked list L1 after the "fun1" operation to it?* 31 -> 32 -> 43 -> 17 -> 34 -> 57 ->91 31 -> 32 -> 17 -> 34 -> 57 ->91 31 -> 32 -> 43 -> 17 -> 57 ->91 31 -> 32 -> 43 -> 17 -> 34 -> 57 31 -> 32 -> 43 -> 57 ->91 31 -> 32 -> 43 -> 34 -> 57 ->91arrow_forward
- The play method in the Player class of the craps game plays an entire game without interaction with the user. Revise the Player class so that its user can make individual rolls of the dice and view the results after each roll. The Player class no longer accumulates a list of rolls, but saves the string representation of each roll after it is made. Add new methods rollDice, getNumberOfRolls, isWinner, and isLoser to the Player class. The last three methods allow the user to obtain the number of rolls and to determine whether there is a winner or a loser. The last two methods are associated with new Boolean instance variables (winner and loser respectively). Two other instance variables track the number of rolls and the string representation of the most recent roll (rollsCount and roll). Another instance variable (atStartup) tracks whether or not the first roll has occurred. At instantiation, the roll, rollsCount, atStartup, winner, and loser variables are set to their appropriate…arrow_forwardConsider the following class that inherits from the built-in list class: class BoundedList (list): def _init_(self, bound): list._init_(self) self.bound = bound Suppose that we want this class to act like a list, except that when append is called, if there are self.bound items in the list already, the first item in the list gets removed. Which of the following methods will properly overload append to work this way? def append(self, item): if len(self) >= self.bound: self.pop(0) list.append(self, item) def append (self, item): if len(self) >= self.bound: self.remove (0) list. append (self, item) def append(self, item): if len(self) >= self.bound: self.pop(0) self.append (item) def append(self, item): list. append (self,item) if len(self) > self.bound: self.pop(0)arrow_forwardPart 2: Sorting the WorkOrders via dates Another error that will still be showing is that there is not Comparable/compareTo() method setup on the WorkOrder class file. That is something you need to fix and code. Implement the use of the Comparable interface and add the compareTo() method to the WorkOrder class. The compareTo() method will take a little work here. We are going to compare via the date of the work order. The dates of the WorkOrder are saved in a MM-DD-YYYY format. There is a dash '-' in between each part of the date. You will need to split both the current object's date and the date sent through the compareTo() parameters. You will have three things to compare against. You first need to check the year. If the years are the same value then you need to go another step to check the months, otherwise you compare them with less than or greater than and return the corresponding value. If you have to check the months it would be the same for years. If the months are the same you…arrow_forward
- Complete the Kennel class by implementing the following methods: addDog(Dog dog) findYoungestDog() method, which returns the Dog object with the lowest age in the kennel. Assume that no two dogs have the same age. Given classes: Class LabProgram contains the main method for testing the program. Class Kennel represents a kennel, which contains an array of Dog objects as a dog list. (Type your code in here.) Class Dog represents a dog, which has three fields: name, breed, and age. (Hint: getAge() returns a dog's age.) For testing purposes, different dog values will be used. Ex. For the following dogs: Rex Labrador 3.5 Fido Healer 2.0 Snoopy Beagle 3.2 Benji Spaniel 3.9 the output is: Youngest Dog: Fido (Healer) (Age: 2.0)arrow_forwardJava programming language I have to create a remove method that removes the element at an index (ind) or space in an array and returns it. Thanks! I have to write the remove method in the code below. i attached the part where i need to write it. public class ourArrayList<T>{ private Node<T> Head = null; private Node<T> Tail = null; private int size = 0; //default constructor public ourArrayList() { Head = Tail = null; size = 0; } public int size() { return size; } public boolean isEmpty() { return (size == 0); } //implement the method add, that adds to the back of the list public void add(T e) { //HW TODO and TEST //create a node and assign e to the data of that node. Node<T> N = new Node<T>();//N.mData is null and N.next is null as well N.setsData(e); //chain the new node to the list //check if the list is empty, then deal with the special case if(this.isEmpty()) { //head and tail refer to N this.Head = this.Tail = N; size++; //return we are done.…arrow_forwardAdd the function min as an abstract function to the class arrayListType to return the smallest element of the list. Also, write the definition of the function min in the class unorderedArrayListType and write a program to test this function. I have 5 tabs: I have tried every solution I can think of with no luck. These are the guides: arrayListType.h arrayListTypeImp.cpp: main.cpp unorderedArraryListType.h unorderedArrayListTypeImp.cpp I am needing these in order to pass the assignment in Cengage Mindtap, please help with codes for each one if possible.arrow_forward
- You will create two programs. The first one will use the data structure Stack and the other program will use the data structure Queue. Keep in mind that you should already know from your video and free textbook that Java uses a LinkedList integration for Queue. Stack Program Create a deck of cards using an array (Array size 15). Each card is an object. So you will have to create a Card class that has a value (1 - 10, Jack, Queen, King, Ace) and suit (clubs, diamonds, heart, spade). You will create a stack and randomly pick a card from the deck to put be pushed onto the stack. You will repeat this 5 times. Then you will take cards off the top of the stack (pop) and reveal the values of the cards in the output. As a challenge, you may have the user guess the value and suit of the card at the bottom of the stack. Queue Program There is a new concert coming to town. This concert is popular and has a long line. The line uses the data structure Queue. The people in the line are objects…arrow_forwardGiven main(), complete the SongNode class to include the function PrintSongInfo(). Then write the PrintPlaylist() function in main.cpp to print all songs in the playlist. DO NOT print the dummy head node. Ex: If the input is: Stomp!380The Brothers JohnsonThe Dude337Quincy JonesYou Don't Own Me151Lesley Gore-1 the output is: LIST OF SONGS-------------Title: Stomp!Length: 380Artist: The Brothers Johnson Title: The DudeLength: 337Artist: Quincy Jones Title: You Don't Own MeLength: 151Artist: Lesley Gore #include "SongNode.h" // TODO: Write PrintPlaylist() function int main() {SongNode* headNode;SongNode* currNode;SongNode* lastNode; string songTitle;string songLength;string songArtist; // Front of nodes list headNode = new SongNode();lastNode = headNode; // Read user input until -1 enteredgetline(cin, songTitle);while (songTitle != "-1") {getline(cin, songLength);getline(cin, songArtist); currNode = new SongNode(songTitle, songLength,…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
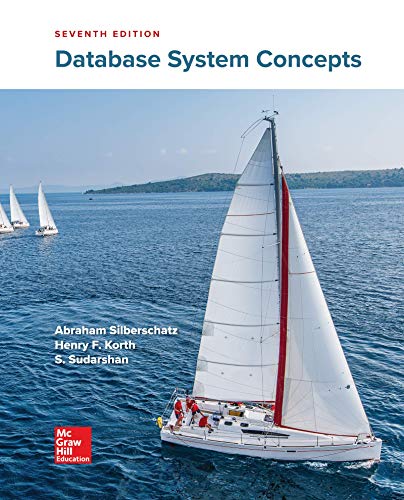
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
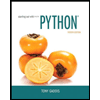
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
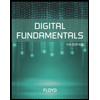
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
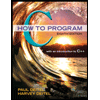
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
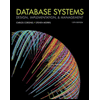
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
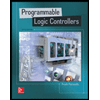
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education