Students are required to create a Python program that performs customized Caesar encryption/decryption, as follows: A- The program should have a main menu, through which the user can choose whether he wants to encrypt a text or decrypt it. B- If the user chose to encrypt plaintext, he will be asked to enter his ID, which is also the name of the input file (i.e. if student ID is 199999, then the input file name should be 199999.txt). The user should not enter the file extension (i.e. .txt), instead, the program should append the extension to the ID automatically. C- The input file must contain the following information: - Student full name - Student ID - Student Age D- After that, if the file does not exist, the program should warn the user about it. If the file exists, the program must import the data from the input file using a function. E- The user then will be asked to choose an operation: Encryption, Decryption, or Exit. 1. Encryption: the encryption process should be done through a group of steps (functions). First, calling a function to read the plaintext data (unencrypted data) from a file called: plain.txt. Then another function will be called, which is the function responsible for creating the key for encryption, the key creation process is explained below. After creating the key, a third function will be called to calculate the rounded average of the key, age, and the number of letters in the student’s name. The main encryption function will proceed with encrypting the data, using the created key. Finally, a function to save the ciphertext will be called, to save the result to a text file, named cipher.txt 2. Decryption: Similar to encryption, the decryption process will have similar functions for reading the ciphertext from cipher.txt, load the key from key.txt, decrypting the ciphertext, and finally saving the recovered plaintext to a file called original.txt. 3. Exit: Choosing this option will exit the program. F- Creating the key: Step 1: Based on the student ID (SID) and age, first calculate the sum of SID digits (i.e. SID: 190190 then sum = 20). Second, find the sum % age (i.e. 20 %18 = 2), and based on this number, you need to left-shift or right-shift the SID. If the value is 1, left-shift by 1 (i.e. the 190190 will become 901901), and so on for values from 1 to 9. If the value is 0, then you need to do a single right-shift (i.e. 190190 will become 019019). Finally, if the value above 9, then you need to subtract the 10 from the value (i.e. sum % age = 33 % 20 = 13, then 13 – 10 = 3 is the number of left-shifts) Step 2: After shifting the SID, the student must create the encryption key as follows: Shifted SID + age + length(name). Example, shifted SID = 901901, age =20, name = Ahmed Ali, then length of name = 9. The generated key = 901901209 Your program must save the key into a file called mykey.txt G- Using the key for encryption: Since the used algorithm is Caesar’s, it means you need to shift the plaintext letters by a certain number, you can obtain this number by rounding the average of your key digits, i.e. if your key is 190190, the total is 20, the average is 3.33, and after rounding it becomes 3. So it means your Caesar algorithm will perform shifting by three digits. In order to increase the strength of your encryption, you must use your key after applying Caesar, as in this example: If your text is “Hello”, and you applied Caesar cipher with a shift of 3, the result is “khoor”. If your key is 190190, your final ciphertext would be “k1h9o0o1r90”. In other words, your final ciphertext is composed of one character from the result of Caesar cipher, then the second character is from the key, and so on until you iterate over the whole plaintext (repeating the key if it is shorter than the plaintext) Your program must save the result in a file called cipher.txt H- Decrypting the ciphertext: In order to decrypt the ciphertext, you must load it from cipher.txt, remove the embedded key, apply Caesar cipher again to decrypt, and finally save the recovered plaintext into a file called original
Students are required to create a Python
A- The program should have a main menu, through which the user can choose whether he wants to encrypt a text or decrypt it.
B- If the user chose to encrypt plaintext, he will be asked to enter his ID, which is also the name of the input file (i.e. if student ID is 199999, then the input file name should be 199999.txt).
The user should not enter the file extension (i.e. .txt), instead, the program should append the extension to the ID automatically.
C- The input file must contain the following information:
- Student full name
- Student ID
- Student Age
D- After that, if the file does not exist, the program should warn the user about it. If the file exists, the program must import the data from the input file using a function.
E- The user then will be asked to choose an operation: Encryption, Decryption, or Exit.
1. Encryption: the encryption process should be done through a group of steps (functions).
First, calling a function to read the plaintext data (unencrypted data) from a file called: plain.txt.
Then another function will be called, which is the function responsible for creating the key for encryption, the key creation process is explained below.
After creating the key, a third function will be called to calculate the rounded average of the key, age, and the number of letters in the student’s name.
The main encryption function will proceed with encrypting the data, using the created key.
Finally, a function to save the ciphertext will be called, to save the result to a text file, named cipher.txt
2. Decryption: Similar to encryption, the decryption process will have similar functions for reading the ciphertext from cipher.txt, load the key from key.txt, decrypting the ciphertext, and finally saving the recovered plaintext to a file called original.txt.
3. Exit: Choosing this option will exit the program.
F- Creating the key:
Step 1: Based on the student ID (SID) and age, first calculate the sum of SID digits (i.e. SID: 190190 then sum = 20). Second, find the sum % age (i.e. 20 %18 = 2), and based on this number, you need to left-shift or right-shift the SID. If the value is 1, left-shift by 1 (i.e. the 190190 will become 901901), and so on for values from 1 to 9. If the value is 0, then you need to do a single right-shift (i.e. 190190 will become 019019). Finally, if the value above 9, then you need to subtract the 10 from the value (i.e. sum % age = 33 % 20 = 13, then 13 – 10 = 3 is the number of left-shifts)
Step 2: After shifting the SID, the student must create the encryption key as follows: Shifted SID + age + length(name). Example, shifted SID = 901901, age =20, name = Ahmed Ali, then length of name = 9. The generated key = 901901209
Your program must save the key into a file called mykey.txt
G- Using the key for encryption:
Since the used
In order to increase the strength of your encryption, you must use your key after applying Caesar, as in this example: If your text is “Hello”, and you applied Caesar cipher with a shift of 3, the result is “khoor”. If your key is 190190, your final ciphertext would be “k1h9o0o1r90”. In other words, your final ciphertext is composed of one character from the result of Caesar cipher, then the second character is from the key, and so on until you iterate over the whole plaintext (repeating the key if it is shorter than the plaintext)
Your program must save the result in a file called cipher.txt
H- Decrypting the ciphertext:
In order to decrypt the ciphertext, you must load it from cipher.txt, remove the embedded key, apply Caesar cipher again to decrypt, and finally save the recovered plaintext into a file called original.txt.
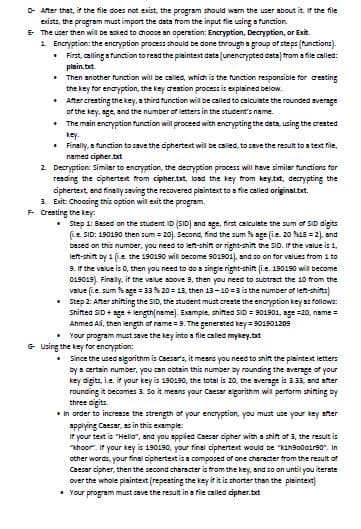
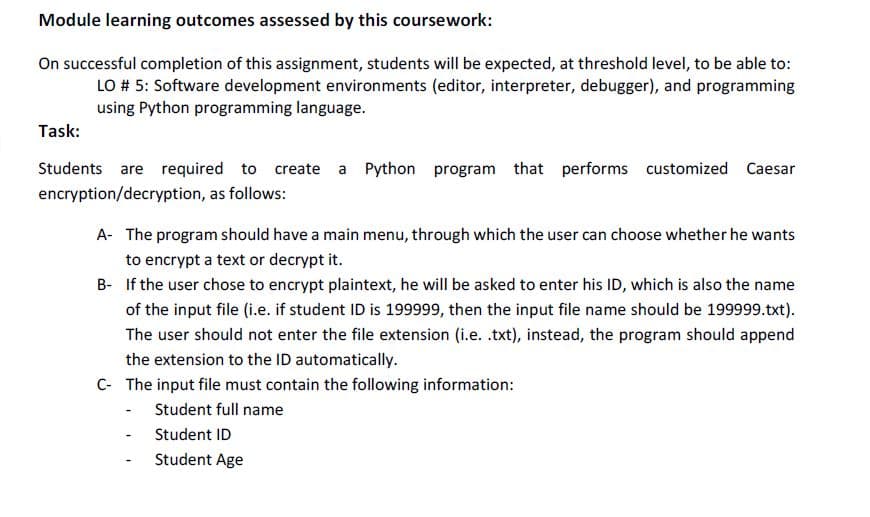

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images
