This question concerns the use of a book cypher to encode a message. Write a program called ‘bookcypher.py’ that accepts: 1. The file name for a Project Gutenberg book text. 2. A message that is to be encoded. 3. A file name for output of the encoded version of the message. The program will create an encoded version of the message by replacing its words with the locations of words in the book. Say, for example, the message is ‘meet me at the usual time and place’, and the Gutenberg text is ‘The Importance of Being Earnest’ by Oscar Wilde. • There is an occurrence of ‘meet’ as the 5th word on the 493rd line of the book text. • There is an occurrence of ‘me’ as the 9th word on the 190th line of the text. • There is an occurrence of ‘at’ as the 6th word on the 8th line of the text. • And so on. Representing a word position as a conjunction of the line number and the index of the word within the line, we can encode the message as 493-5, 190-9, 8-6, etc. Sample I/O (user input in bold): Enter the book filename: ImportanceOfBeingEarnest.txt Enter the message: Meet me at the usual time and place Enter the output filename: secret.txt BEGIN 492-5 129-9 4-10 1-1 174-3 71-1 4-13 253-6 END The program will output results to the given file (‘secret.txt’ in the example) AND to the screen. • The first line of results will consist of the word ‘BEGIN’. • Subsequent lines will consist of word encodings, one per line. • A word encoding will consist of a line number, a hyphen, and a word index. • The last line of results will consist of the word ‘END’. NOTE: • You can assume the message does not contain punctuation. • If a word match cannot be found, the program should output the word itself instead of a line number and word index encoding. • You can use the string ‘split()’ method to split a sentence up into words e.g. >>> 'how now, brown cow.'.split() ['how', 'now,', 'brown', 'cow.'] >>> The result is a list of strings, one for each word. • To clean up the results of split(), i.e. to get rid of bits of punctuation stuck to words, you can use the string ‘strip()’ method e.g. >>> 'eh?'.strip('?!.,;:)([]{}\'" \n') 'eh' >>> "('ello!)".strip('?!.,;:)([]{}\'" \n') 'ello' >>> The parameter to the strip() method is a string containing instances of all the characters we want to remove.
This question concerns the use of a book cypher to encode a message.
Write a
1. The file name for a Project Gutenberg book text.
2. A message that is to be encoded.
3. A file name for output of the encoded version of the message.
The program will create an encoded version of the message by replacing its words with the locations
of words in the book.
Say, for example, the message is ‘meet me at the usual time and place’, and the Gutenberg text is
‘The Importance of Being Earnest’ by Oscar Wilde.
• There is an occurrence of ‘meet’ as the 5th word on the 493rd line of the book text.
• There is an occurrence of ‘me’ as the 9th word on the 190th line of the text.
• There is an occurrence of ‘at’ as the 6th word on the 8th line of the text.
• And so on.
Representing a word position as a conjunction of the line number and the index of the word within
the line, we can encode the message as 493-5, 190-9, 8-6, etc.
Sample I/O (user input in bold):
Enter the book filename:
ImportanceOfBeingEarnest.txt
Enter the message:
Meet me at the usual time and place
Enter the output filename:
secret.txt
BEGIN
492-5
129-9
4-10
1-1
174-3
71-1
4-13
253-6
END
The program will output results to the given file (‘secret.txt’ in the example) AND to the screen.
• The first line of results will consist of the word ‘BEGIN’.
• Subsequent lines will consist of word encodings, one per line.
• A word encoding will consist of a line number, a hyphen, and a word index.
• The last line of results will consist of the word ‘END’.
NOTE:
• You can assume the message does not contain punctuation.
• If a word match cannot be found, the program should output the word itself instead of a line
number and word index encoding.
• You can use the string ‘split()’ method to split a sentence up into words e.g.
>>> 'how now, brown cow.'.split()
['how', 'now,', 'brown', 'cow.']
>>>
The result is a list of strings, one for each word.
• To clean up the results of split(), i.e. to get rid of bits of punctuation stuck to words, you
can use the string ‘strip()’ method e.g.
>>> 'eh?'.strip('?!.,;:)([]{}\'" \n')
'eh'
>>> "('ello!)".strip('?!.,;:)([]{}\'" \n')
'ello'
>>>
The parameter to the strip() method is a string containing instances of all the characters
we want to remove.

Step by step
Solved in 3 steps with 3 images

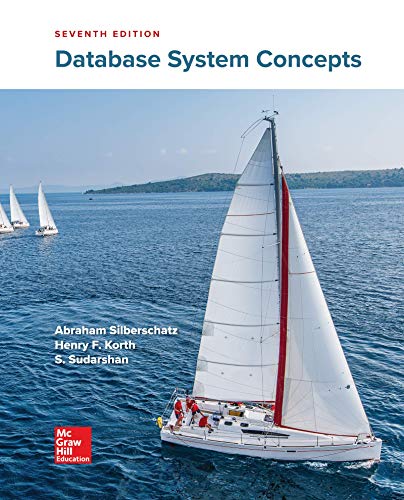
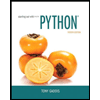
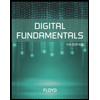
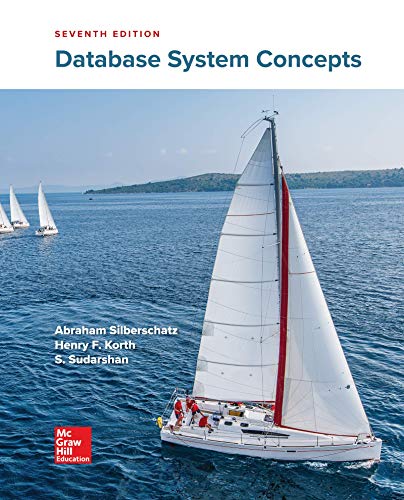
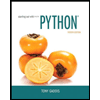
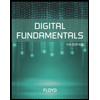
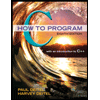
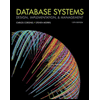
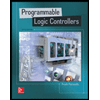