Suppose that I have a hash table data[CAPACITY]. The hash table uses open addressing with a quadratic probing. The table size is a global constant called CAPACITY. Locations of the table that have NEVER been used will contain the key -1. All valid keys will be non-negative, and the hash function is: int hash_function(int key) { return (key $ CAPACITY); Complete the implementation of the following functions. There is no need to check the precondition, but your code must be as efficient as possible. Implement the following functions. const int CAPACITY = 10; void initTable(int data[]);// data[CAPACITY] ={-1, -1, -, -1} void printTable(int data[]); int hash_function(int key); void hashInsert(int data[], int key); bool key_occurs(int data[], int search_key); Part 1: Insert keys {10, 22, 11, 31, 24, 88, 38, 21} into an empty hash table with CAPACITY = 10 using quadratic probing (c1=0 and c2=1) to resolve collision (You shouldn't use a for/while loop except to increment the value of i for quadratic probing). Part 2: Check whether following numbers (11, 31, and 23) are in the table. (You shouldn't use a for/while loop except to increment the value of i for quadratic probing).
Suppose that I have a hash table data[CAPACITY]. The hash table uses open addressing with a quadratic probing. The table size is a global constant called CAPACITY. Locations of the table that have NEVER been used will contain the key -1. All valid keys will be non-negative, and the hash function is: int hash_function(int key) { return (key $ CAPACITY); Complete the implementation of the following functions. There is no need to check the precondition, but your code must be as efficient as possible. Implement the following functions. const int CAPACITY = 10; void initTable(int data[]);// data[CAPACITY] ={-1, -1, -, -1} void printTable(int data[]); int hash_function(int key); void hashInsert(int data[], int key); bool key_occurs(int data[], int search_key); Part 1: Insert keys {10, 22, 11, 31, 24, 88, 38, 21} into an empty hash table with CAPACITY = 10 using quadratic probing (c1=0 and c2=1) to resolve collision (You shouldn't use a for/while loop except to increment the value of i for quadratic probing). Part 2: Check whether following numbers (11, 31, and 23) are in the table. (You shouldn't use a for/while loop except to increment the value of i for quadratic probing).
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Please help with C++ hashing question in C++ language. Sample output also in image. Thank you.
![Suppose that I have a hash table data[CAPACITY]. The hash table uses open addressing with a quadratic
probing. The table size is a global constant called CAPACITY. Locations of the table that have NEVER
been used will contain the key -1. All valid keys will be non-negative, and the hash function is:
int hash_function (int key)
{
return (key & CAPACITY);
}
Complete the implementation of the following functions. There is no need to check the
precondition, but your code must be as efficient as possible.
Implement the following functions.
const int CAPACITY = 10;
void initTable(int data[]);// data[CAPACITY] ={-1, -1, -, -1}
void printTable(int data[]);
int hash_function(int key);
void hashInsert(int data[], int key);
bool key_occurs(int data[], int search_key);
Part 1: Insert keys {10, 22, 11, 31, 24, 88, 38, 21} into an empty hash table with CAPACITY = 10 using
quadratic probing (c1=0 and c2=1) to resolve collision (You shouldn't use a for/while loop except to
increment the value of i for quadratic probing).
Part 2: Check whether following numbers (11, 31, and 23) are in the table. (You shouldn't use a for/while
loop except to increment the value of i for quadratic probing).](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F620c60a1-10fe-416e-8b85-1e75a34b227e%2Fe1b04271-6df5-4e79-a113-b8cfb7927e83%2Fgxnhamn_processed.png&w=3840&q=75)
Transcribed Image Text:Suppose that I have a hash table data[CAPACITY]. The hash table uses open addressing with a quadratic
probing. The table size is a global constant called CAPACITY. Locations of the table that have NEVER
been used will contain the key -1. All valid keys will be non-negative, and the hash function is:
int hash_function (int key)
{
return (key & CAPACITY);
}
Complete the implementation of the following functions. There is no need to check the
precondition, but your code must be as efficient as possible.
Implement the following functions.
const int CAPACITY = 10;
void initTable(int data[]);// data[CAPACITY] ={-1, -1, -, -1}
void printTable(int data[]);
int hash_function(int key);
void hashInsert(int data[], int key);
bool key_occurs(int data[], int search_key);
Part 1: Insert keys {10, 22, 11, 31, 24, 88, 38, 21} into an empty hash table with CAPACITY = 10 using
quadratic probing (c1=0 and c2=1) to resolve collision (You shouldn't use a for/while loop except to
increment the value of i for quadratic probing).
Part 2: Check whether following numbers (11, 31, and 23) are in the table. (You shouldn't use a for/while
loop except to increment the value of i for quadratic probing).
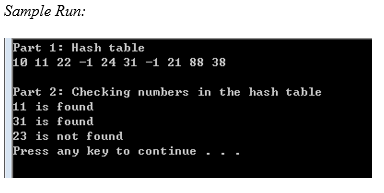
Transcribed Image Text:Sample Run:
Part 1: Hash table
10 11 22 -1 24 31 -1 21 88 38
Part 2: Checking numbers in the hash table
11 is found
31 is found
23 is not found
Press any key to continue . .
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Recommended textbooks for you
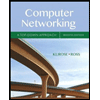
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
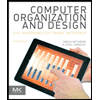
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
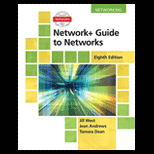
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
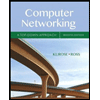
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
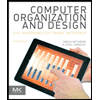
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
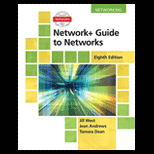
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
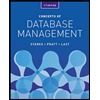
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
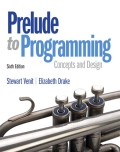
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
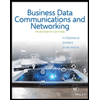
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY