Write a program that reads two positive integers D and i (in this order) from its input and adds the rest of the integers (all non-negative) in the input to a hash table of size D. Finally the program should output the content of the table. Use closed hashing with linear probing where the parameter is i and h(x)=x. The content of the table should be printed in the following format: -1 7 8 ..... where the output contains exactly D lines and an empty slot is printed as -1.
"please turn this python code to c++"
class hashTable:
def _init_(self):
self.size = int(input('Enter the size of hash table:'))
#initializwe the table with 0
self.table = list(None for i in range(self.size))
self.elementCount = 0
# function to get the position for element
def hashFunction(self,key):
return key%self.size
#function to check if hash table is full or not
def isFull(self):
if self.elementCount == self.size:
return True
else:
return False
#function to insert data into hash table
def insert(self,data):
#check if table is full
if self.isFull():
print("hash table is full")
return False
position = self.hashFunction(data)
#checking if positiohn is empty
if self.table[position] == None:
self.table[position] = data
self.elementCount+=1
#if collision occur linear probing is done
else:
while self.table[position] != None:
position+=1
if position >= self.size:
position = 0
self.table[position] = data
#function to display the hash table
def display(self):
for i in range( self.size):
print(self.table[i]+"\n")
ht = hashTable()
i = int(input("Enter interger to be inserted into hash table"))
ht.insert(i)
ht.display()
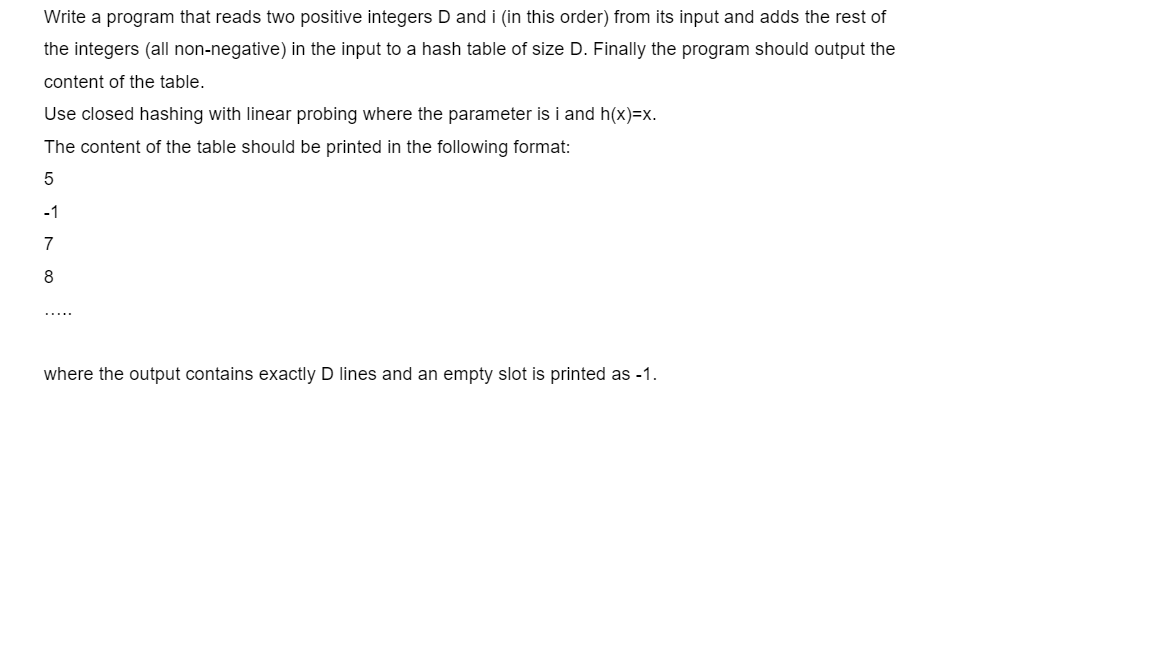

Step by step
Solved in 3 steps with 1 images

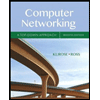
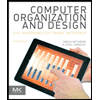
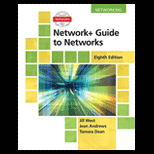
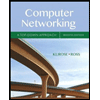
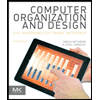
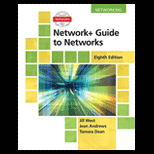
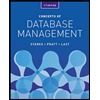
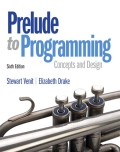
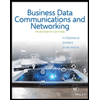