Suppose that there are N events to be held this year. The ith event is scheduled from day si to day ti, and free food is to be served for that event every day from day si to ti (inclusive). Your task is to find out how many days there are in which free food is served by at least one event. For example, let N = 3 events. The first event is held from day 10 to 14, the second event is held from day 13 to 17, and the third event is held from day 25 to 26. The days in which free food is served by at least one event are 10, 11, 12, 13, 14, 15, 16, 17, 25, 26, for a total of 10 days. Note that both events serve free food on days 13 and 14, and therefore they are only counted once. The first line the user will supply you is an integer, N, denoting the number of events. Each of the next N lines contains two integers si and ti denoting that the ith event will be held from si to ti (inclusive), and free food is served for all of those days. The output contains a single integer which denotes the number of days in which free food is served by at least one event.
Suppose that there are N events to be held this year. The ith event is scheduled from day si to day ti, and free food is to be served for that event every day from day si to ti (inclusive). Your task is to find out how many days there are in which free food is served by at least one event.
For example, let N = 3 events. The first event is held from day 10 to 14, the second event is held from day 13 to 17, and the third event is held from day 25 to 26. The days in which free food is served by at least one event are 10, 11, 12, 13, 14, 15, 16, 17, 25, 26, for a total of 10 days. Note that both events serve free food on days 13 and 14, and therefore they are only counted once.
The first line the user will supply you is an integer, N, denoting the number of events. Each of the next N lines contains two integers si and ti denoting that the ith event will be held from si to ti (inclusive), and free food is served for all of those days.
The output contains a single integer which denotes the number of days in which free food is served by at least one event.
Learning Objectives
- Be able to take a variable number of inputs based on a user's value.
- Be able to conditionally execute code.
- Be able to take a word problem and split it into the essential elements.
- Be able to write a program to solve the given problem.
Template
def get_input():
num_events = int(input())
events = []
# TODO: Collect the events (si and ti per line)
# TODO: After collecting events, return the list of events
# Each event is a range from si to ti (inclusively!!)
# Notice I already created an empty list called events above.
return events
def calculate(events):
# Events above comes from your get_input() function and must have
# the format of (si, ti) for each event element.
# TODO: Calculate **unique** event days.
unique_days = 0
# Return the number of **unique** event days.
return unique_days
if __name__ == "__main__": events = get_input() free_food = calculate(events) print(free_food)
Assignment
Step 1
Write a function called get_input():
def get_input():
- First input the number of events. This is the first line of input and is the integer value N from the problem.
- Then read N more lines. Each one of these lines has two values: si and ti, where i is the line number.
- Finally, return a list of ranges from the start to end times. Remember that these times are inclusive!
- A range can be created using the range(start, end) function which will produce a range from [start, end).
Step 2
Write a function called calculate():
def calculate(events):
- The events passed to this function is the same list of ranges returned by your get_input() function.
- Recall that the events are range() items from start to end (inclusively).
- Your job in this function is to count the number of unique days where free food is offered.
- Return the number of unique days where free food is offered given the events list.
Step 3
Write your testing framework. You are required to have if __name__ == "__main__". We will be testing your code as a module, and WE need to control when to run get_input versus calculate(). NOTE: If you do not do this, your code will be penalized for not being directly testable as a submission.
if __name__ == "__main__":
events = get_input()
free_food = calculate(events)
print(free_food)
Testing
Given the following input:
3
10 13
11 12
12 15
your code have the following output:
6
You should thoroughly test your code to ensure it works properly.
Hints and Tips
- The data type you select will be very important. You can use a list or a set. However, a set will automatically do what you want.
- Think about what the problem is asking you to do. The simplest form is enumerate all days from the start to the end of each day. The "harder" part is to remove duplicates. If you recall what a set does and its properties, you'd know that if you insert a value into a set twice, the set will only contain it once. This is exactly what you want to do for this lab.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

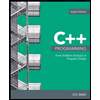
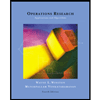
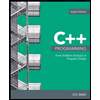
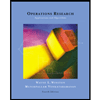