Tape for a Turing Machine (Doubly-linked List)”.(I USE ECLIPSE & RUN CHECK ONLINE CONSOLE) A class named Tape to represent Turing machine tapes. The class should have an instance variable of type Cell that points to the current cell with the method: public Cell getCurrentCell() -- returns the pointer that points to the current cell. public char getContent() -- returns the char from the current cell. public void setContent(char ch) -- changes the char in the current cell to the specified value. Don't forget the Javadoc comments. IT WOULD HAVE A PUBLIC CLASS public void moveLeft() -- moves the current cell one position to the left along the tape. Note that if the current cell is the leftmost cell that exists, then a new cell must be created and added to the tape at the left of the current cell, and then the current cell pointer can be moved to point to the new cell. The content of the new cell should be a blank space. (Remember that the Turing machine's tape is conceptually infinite, so the linked list must be prepared to expand on-demand when the machine wants to move past the current end of the list.) public void moveRight() -- moves the current cell one position to the right along the tape. Note that if the current cell is the rightmost cell that exists, then a new cell must be created and added to the tape at the right of the current cell, and then the current cell pointer can be moved to point to the new cell. The content of the new cell should be a blank space. public String getTapeContents() -- returns a String consisting of the chars from all the cells on the tape, read from left to right, except that leading or trailing blank characters should be discarded. The current cell pointer should not be moved by this method; it should point to the same cell after the method is called as it did before. You can create a different pointer to move along the tape and get the full contents. (This method is the hardest one to implement.) It is also useful to have a constructor that creates a tape that initially consists of a single cell. The cell should contain a blank space, and the current cell pointer should point to it. (The alternative -- letting the current cell pointer be null to represent a completely blank tape To test your Tape class, you can run the programs that are defined by the files TestTape.java, TestTapeGUI.java, and TestTuringMachine.java. The first two programs just do things with a tape, to test whether it is functioning properly. TestTuringMachine actually creates and runs several Turing machines, using your Tape class to represent the machines' tapes.
Tape for a Turing Machine (Doubly-linked List)”.(I USE ECLIPSE & RUN CHECK ONLINE CONSOLE)
A class named Tape to represent Turing machine tapes. The class should have an instance variable of type Cell that points to the current cell with the method:
public Cell getCurrentCell() -- returns the pointer that points to the current cell.
public char getContent() -- returns the char from the current cell.
public void setContent(char ch) -- changes the char in the current cell to the specified value. Don't forget the Javadoc comments. IT WOULD HAVE A PUBLIC CLASS
public void moveLeft() -- moves the current cell one position to the left along the tape. Note that if the current cell is the leftmost cell that exists, then a new cell must be created and added to the tape at the left of the current cell, and then the current cell pointer can be moved to point to the new cell. The content of the new cell should be a blank space. (Remember that the Turing machine's tape is conceptually infinite, so the linked list must be prepared to expand on-demand when the machine wants to move past the current end of the list.)
public void moveRight() -- moves the current cell one position to the right along the tape. Note that if the current cell is the rightmost cell that exists, then a new cell must be created and added to the tape at the right of the current cell, and then the current cell pointer can be moved to point to the new cell. The content of the new cell should be a blank space.
public String getTapeContents() -- returns a String consisting of the chars from all the cells on the tape, read from left to right, except that leading or trailing blank characters should be discarded. The current cell pointer should not be moved by this method; it should point to the same cell after the method is called as it did before. You can create a different pointer to move along the tape and get the full contents. (This method is the hardest one to implement.)
It is also useful to have a constructor that creates a tape that initially consists of a single cell. The cell should contain a blank space, and the current cell pointer should point to it. (The alternative -- letting the current cell pointer be null to represent a completely blank tape
To test your Tape class, you can run the programs that are defined by the files TestTape.java, TestTapeGUI.java, and TestTuringMachine.java. The first two programs just do things with a tape, to test whether it is functioning properly. TestTuringMachine actually creates and runs several Turing machines, using your Tape class to represent the machines' tapes.
USE THIS CLASS
package turing;
/**
* Represents one cell on a Turing Machine tape.
*/
public class Cell {
public char content; // The character in this cell.
public Cell next; // Pointer to the cell to the right of this one.
public Cell prev; // Pointer to the cell to the left of this one.
}
TestTape.java
package turing;
// A test program for the Tape class that calls most of the methods in that class.
//
// The output from this program should be: Tape Conents: Hello World
// Final position at the W
public class TestTape {
public static void main(String[] args) {
Tape tape = new Tape();
for (int i = 0; i < "World".length(); i++) {
tape.setContent("World".charAt(i));
tape.moveRight();
}
for (int i = 0; i < "Hello World".length(); i++)
tape.moveLeft();
for (int i =0; i < "Hello".length(); i++) {
tape.setContent("Hello".charAt(i));
tape.moveRight();
}
System.out.println("Tape Conents: " + tape.getTapeContents());
tape.moveRight();
System.out.println("Final position at the " + tape.getContent());
}
}
TestTapeGUI.java
package turing;
// A test program for the Tape class that creates a window containing
// a graphical representation of the tape with an arrow pointing to
// the current cell. There are buttons for moving the current cell to
// the left and to the right. A text-input box shows the content of
// the current cell. This box can be edited, and there is a "Set" button
// that copies the contents of the cell (actually just the first character)
// to the current cell.
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class TestTapeGUI extends JPanel {
public static void main(String[] args) {
JFrame window = new JFrame("Test Tape");
window.setContentPane( new TestTapeGUI("Test") );
window.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
window.pack();
window.setLocation(100,100);
window.setVisible(true);
}
private Tape tape;
private TapePanel tapePanel;
private JButton moveLeftButton, moveRightButton, setContentButton;
private JTextField contentInput;
private class TapePanel extends JPanel {
public void paintComponent(Graphics g) {
super.paintComponent(g);
int mid = getWidth() / 2;
g.drawLine(0,30,getWidth(),30);
g.drawLine(0,60,getWidth(),60);
g.drawLine(mid,70,mid,110);
g.drawLine(mid,70,mid+15,85);
g.drawLine(mid,70,mid-15,85);
int ct = (mid / 30) + 1;
for (int i = 0; i <= ct; i++) {
g.drawLine(mid + 30*i - 15, 30, mid + 30*i - 15, 59);
g.drawString("" + tape.getContent(), mid + 30*i - 8, 53);
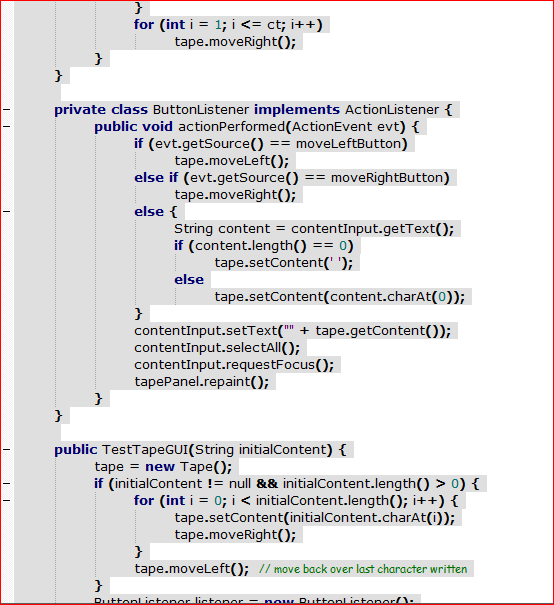
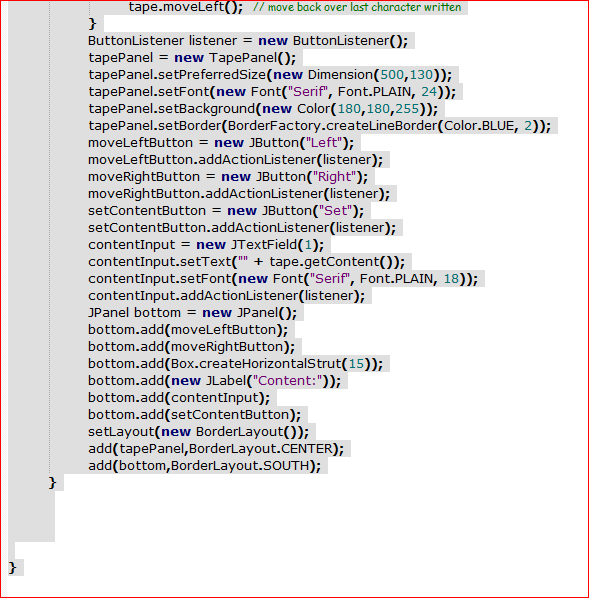

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

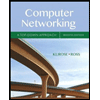
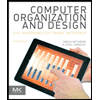
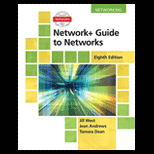
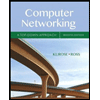
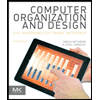
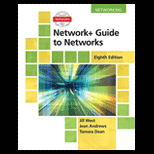
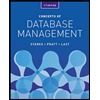
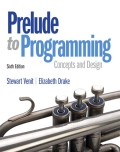
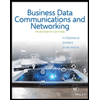