Task 1: • Declare the first function as follow: 1. Return type: int. 2. Function name: bintodec (Binary to Decimal). 3. Parameter List: a string. (char *str). • Declare the second function as follow: o Return type: void. o Function name: dectobin (Decimal to Binary). o Parameter List: an int(named x), a string. (char *bin) Task 2: Define bintodec(char *str): Task 3: Each element of str is a character ('0' or '1'). Declare an int named x and initialize it as zero. To convert str to a decimal number, you can start from left of str to right. For example, to convert "00101100" to a decimal number, start at "00101100". Decrement the ASCII code of '0' to find if the number is 0 or 1. Add it to twice of x. Hint: x= (str[i] - '0') + x * 2; Continue for the next character up to the end of str. Retune x Define dectobin (int x, char *bin). In a loop, assign 0 to all 7 elements of bin. Assign '\0' to bin[7]. Repeat the following statements as far as x is not 0. The remainder of any number by two is either 0 or one. Add the ASCII code of zero to convert the reminder to a character and assign the character to the appropriate element of bin. Divide x by 2 and continue the loop. • No need to return any value.
Task 1: • Declare the first function as follow: 1. Return type: int. 2. Function name: bintodec (Binary to Decimal). 3. Parameter List: a string. (char *str). • Declare the second function as follow: o Return type: void. o Function name: dectobin (Decimal to Binary). o Parameter List: an int(named x), a string. (char *bin) Task 2: Define bintodec(char *str): Task 3: Each element of str is a character ('0' or '1'). Declare an int named x and initialize it as zero. To convert str to a decimal number, you can start from left of str to right. For example, to convert "00101100" to a decimal number, start at "00101100". Decrement the ASCII code of '0' to find if the number is 0 or 1. Add it to twice of x. Hint: x= (str[i] - '0') + x * 2; Continue for the next character up to the end of str. Retune x Define dectobin (int x, char *bin). In a loop, assign 0 to all 7 elements of bin. Assign '\0' to bin[7]. Repeat the following statements as far as x is not 0. The remainder of any number by two is either 0 or one. Add the ASCII code of zero to convert the reminder to a character and assign the character to the appropriate element of bin. Divide x by 2 and continue the loop. • No need to return any value.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter7: User-defined Simple Data Types, Namespaces, And The String Type
Section: Chapter Questions
Problem 1TF
Related questions
Question
100%
Hello. Please answer the attached C
*If correctly fulfill and answer all of the tasks correctly, I will give you a thumbs up. Thanks.
![Task 1:
•
Declare the first function as follow:
1. Return type: int.
2. Function name: bintodec (Binary to Decimal).
3. Parameter List: a string. (char *str).
• Declare the second function as follow:
o Return type: void.
o
o
Function name: dectobin (Decimal to Binary).
Parameter List: an int(named x), a string. (char *bin)
Task 2:
Define bintodec(char *str):
•
Each element of str is a character ('0' or '1').
Declare an int named x and initialize it as zero.
•
To convert str to a decimal number, you can start from left of str to right. For
example, to convert "00101100" to a decimal number, start at "00101100".
Decrement the ASCII code of '0' to find if the number is 0 or 1.
Add it to twice of x. Hint: x= (str[i] - '0') + x * 2;
Continue for the next character up to the end of str.
Retune x
Task 3:
Define dectobin (int x, char *bin).
In a loop, assign 0 to all 7 elements of bin.
Assign '\0' to bin[7].
Repeat the following statements as far as x is not 0.
• The remainder of any number by two is either 0 or one. Add the ASCII code
of zero to convert the reminder to a character and assign the character to the
appropriate element of bin.
•
Divide x by 2 and continue the loop.
• No need to return any value.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1223c4c6-4ebb-4911-bc0d-edb37c26385f%2F7f7045b3-2bae-4094-8136-464f9018528d%2F5q5twho_processed.png&w=3840&q=75)
Transcribed Image Text:Task 1:
•
Declare the first function as follow:
1. Return type: int.
2. Function name: bintodec (Binary to Decimal).
3. Parameter List: a string. (char *str).
• Declare the second function as follow:
o Return type: void.
o
o
Function name: dectobin (Decimal to Binary).
Parameter List: an int(named x), a string. (char *bin)
Task 2:
Define bintodec(char *str):
•
Each element of str is a character ('0' or '1').
Declare an int named x and initialize it as zero.
•
To convert str to a decimal number, you can start from left of str to right. For
example, to convert "00101100" to a decimal number, start at "00101100".
Decrement the ASCII code of '0' to find if the number is 0 or 1.
Add it to twice of x. Hint: x= (str[i] - '0') + x * 2;
Continue for the next character up to the end of str.
Retune x
Task 3:
Define dectobin (int x, char *bin).
In a loop, assign 0 to all 7 elements of bin.
Assign '\0' to bin[7].
Repeat the following statements as far as x is not 0.
• The remainder of any number by two is either 0 or one. Add the ASCII code
of zero to convert the reminder to a character and assign the character to the
appropriate element of bin.
•
Divide x by 2 and continue the loop.
• No need to return any value.
![Task 4:
Check the functions by the following main() function. Change num and binaryNum to
check your code.
int main()
{
int num = 12;
char binaryNum[] = "00101100";
char binaryRep[8];
int decRep;
int i, j;
dectobin(num, binaryRep);
decRep = bintodec(binaryNum);
printf(" Binary representation of %d is %s.\n", num, binaryRep);
printf(" Decimal representation of %s is %d.\n", binaryNum, decRep);
return 0;
}
Test Your code:
1.
2.
3.
int num = 12; the output should be 0000 1100.
int num = 67; the output should be 0100 0011.
int num = 32; and the output should be 0100 0000.
1. char binaryNum[ ] = "00101100", the output is 44.
2. char binaryNum[ ] = "01000011"; the output should be 67.
3. char binaryNum[ ] = "01000000"; and the output should be 32.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1223c4c6-4ebb-4911-bc0d-edb37c26385f%2F7f7045b3-2bae-4094-8136-464f9018528d%2Ftam8r1c_processed.png&w=3840&q=75)
Transcribed Image Text:Task 4:
Check the functions by the following main() function. Change num and binaryNum to
check your code.
int main()
{
int num = 12;
char binaryNum[] = "00101100";
char binaryRep[8];
int decRep;
int i, j;
dectobin(num, binaryRep);
decRep = bintodec(binaryNum);
printf(" Binary representation of %d is %s.\n", num, binaryRep);
printf(" Decimal representation of %s is %d.\n", binaryNum, decRep);
return 0;
}
Test Your code:
1.
2.
3.
int num = 12; the output should be 0000 1100.
int num = 67; the output should be 0100 0011.
int num = 32; and the output should be 0100 0000.
1. char binaryNum[ ] = "00101100", the output is 44.
2. char binaryNum[ ] = "01000011"; the output should be 67.
3. char binaryNum[ ] = "01000000"; and the output should be 32.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
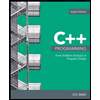
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
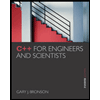
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
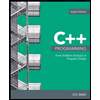
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
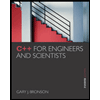
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr