Implement and call the following C-style function in MIPS assembly code. You do not have to worry about invalid values of n. The min() function int min(int n, int x[]}{ int minval; int i; minval = x[0]; for (i=1; i
Implement and call the following C-style function in MIPS assembly code. You do not have to worry about invalid values of n. The min() function int min(int n, int x[]}{ int minval; int i; minval = x[0]; for (i=1; i
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter10: Pointers
Section10.1: Addresses And Pointers
Problem 7E
Related questions
Question
100%
Dont answer copied in previous answer
else report the answer suredont
dont answer without knowledge please I am posting this from so many times same answer
![Objectives
• Implement loop in MIPS assembly code
• Implement decision statement in MIPS assembly code
Implement array in MIPS assembly code
• Pass array as a function parameter and access it in a function in MIPS assembly code
• Process array data in MIPS assembly code
Task
Implement and call the following C-style function in MIPS assembly code. You do not have to worry about
invalid values of n.
The min() function
int min(int n, int x[]){
int minval;
int i;
minval = x[0];
for (i=1; i<n; i+){
if ( x[i] < minval {
minval = x[i];
}
}
return minval;
}
Implementation Strategy
While you can attempt to code everything at once and hope you can mold it into a working program, it
would be better to approach this professionally and implement and test one feature at a time. You might
employ the following strategy.
1. Define and call the min() function. Have it print a message.
2. Add a counted loop to print 0 to 9.
3. Pass n, the array length, as the first parameter to min and print the values 0 to n-1.
4. Pass an array address as the second parameter and print the array contents.
5. Add the code to find the array minimum value, and return it in register $v0.
The main Function
Your main function should define three integer arrays, each of a different size. It should call the min()
function with each array, and print the returned minimum value.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0bd43c16-53f4-4a1b-b8cb-203edadf5783%2Fbc91eab0-25fc-4945-968a-bb5a5850d992%2Fcm8n7l6_processed.png&w=3840&q=75)
Transcribed Image Text:Objectives
• Implement loop in MIPS assembly code
• Implement decision statement in MIPS assembly code
Implement array in MIPS assembly code
• Pass array as a function parameter and access it in a function in MIPS assembly code
• Process array data in MIPS assembly code
Task
Implement and call the following C-style function in MIPS assembly code. You do not have to worry about
invalid values of n.
The min() function
int min(int n, int x[]){
int minval;
int i;
minval = x[0];
for (i=1; i<n; i+){
if ( x[i] < minval {
minval = x[i];
}
}
return minval;
}
Implementation Strategy
While you can attempt to code everything at once and hope you can mold it into a working program, it
would be better to approach this professionally and implement and test one feature at a time. You might
employ the following strategy.
1. Define and call the min() function. Have it print a message.
2. Add a counted loop to print 0 to 9.
3. Pass n, the array length, as the first parameter to min and print the values 0 to n-1.
4. Pass an array address as the second parameter and print the array contents.
5. Add the code to find the array minimum value, and return it in register $v0.
The main Function
Your main function should define three integer arrays, each of a different size. It should call the min()
function with each array, and print the returned minimum value.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
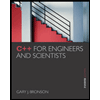
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
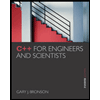
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr