The American Red Cross wants you to write a program that will calculate the average pints of blood donated during a blood drive. The program should take in the number of pints donated during the drive, up to twenty-four-hour drive period, from an input file, bloodDrive.txt. The average pints, highest pints, and lowest pints donated during that period should be calculated and written to a file called bloodResults.txt. We will only run the program once so a yes/no loop is not needed. Step 1: Note that the getHigh, getLow, and getAverage functions do not change. We need to modify modules getPints and displayInfo to meet the new requirements. Complete the pseudocode below for the two modules according to instructions: In the getPints Module (notice there is a new pass-by-reference parameter count) Declare an input file with the name bloodInFile. Open the internal file (bloodInFile) and a text file named bloodDrive.txt. If cannot open file then display an error message Set count = 0 Input one value from the file While not end-of-file, store value in the array[count], add 1 to count, and input another value Close the file. Module getPints(Real pints[], Integer Ref count) End Module In the displayInfo Module Declare an output file with the name bloodOutFile. Open the internal file (bloodOutFile) and a text file named bloodResults.txt. Write the string “Report generated by [YourName] ” to the file. Write the string “Blood drive period: ” to the file. Write the value of hours to the file. Write the string “Average Pints: ” to the file. Write the value of averagePints to the file. Do the same thing for highest pints and lowest pints Close the bloodOutFile. Module displayInfo(Integer hours, Real averagePints, Real highPints, Real lowPints,) End Module Pseudocode for the main module (MAX_HOURS is a global named constant) Module main() //Declare local variables Declare Real pints[MAX_HOURS] Declare Integer hours Declare Real avePints, highPints, lowPints Call getPints(pints, hours) Display “hours: “, hours Display “first value: “, hours[0] Display “last value: “, hours[hours – 1] Set avePints = getAverage(pints, hours) Set highPints = getHigh(pints, hours) Set lowPints = getLow(pints, hours) Call displayInfo(hours, avePints, highPints, lowPints) End Module File Access and C++ Code The goal of this lab is to convert the blood drive program from above to C++ code. Create a text file name bloodDrive.txt in the project folder with the following values (or you can also get it from Canvas). Make sure to put one blank line after the last value. 43 25 64 35 19 37 46 Run your program and check against the following output file, bloodResults.txt. If there are errors, go back through the steps to troubleshoot. You should confirm that the program displays 7, 43, and 46 to the screen for hours, first value, and last value. Report generated by [YourName] Blood drive period: 7 Average pints: 38.4 Highest pints: 64.0 Lowest pints: 19.0 You might want to try another test case with fewer or more than 7 values to make sure your program can handle it. Don’t forget to include for file input and output. You would need to include and set the precision for the output file (not cout) to get one digit after the decimal point. When your code is complete and runs properly, copy/paste both the source code and the output file (bloodResults.txt) for the test case above.
The American Red Cross wants you to write a program that will calculate the average pints of blood donated during a blood drive. The program should take in the number of pints donated during the drive, up to twenty-four-hour drive period, from an input file, bloodDrive.txt. The average pints, highest pints, and lowest pints donated during that period should be calculated and written to a file called bloodResults.txt. We will only run the program once so a yes/no loop is not needed.
Step 1: Note that the getHigh, getLow, and getAverage functions do not change. We need to modify modules getPints and displayInfo to meet the new requirements. Complete the pseudocode below for the two modules according to instructions:
In the getPints Module (notice there is a new pass-by-reference parameter count)
- Declare an input file with the name bloodInFile.
- Open the internal file (bloodInFile) and a text file named bloodDrive.txt.
- If cannot open file then display an error message
- Set count = 0
- Input one value from the file
- While not end-of-file, store value in the array[count], add 1 to count, and input another value
- Close the file.
Module getPints(Real pints[], Integer Ref count)
End Module
In the displayInfo Module
- Declare an output file with the name bloodOutFile.
- Open the internal file (bloodOutFile) and a text file named bloodResults.txt.
- Write the string “Report generated by [YourName] ” to the file.
- Write the string “Blood drive period: ” to the file.
- Write the value of hours to the file.
- Write the string “Average Pints: ” to the file.
- Write the value of averagePints to the file.
- Do the same thing for highest pints and lowest pints
- Close the bloodOutFile.
Module displayInfo(Integer hours, Real averagePints,
Real highPints, Real lowPints,)
End Module
Pseudocode for the main module (MAX_HOURS is a global named constant)
Module main()
//Declare local variables
Declare Real pints[MAX_HOURS]
Declare Integer hours
Declare Real avePints, highPints, lowPints
Call getPints(pints, hours)
Display “hours: “, hours
Display “first value: “, hours[0]
Display “last value: “, hours[hours – 1]
Set avePints = getAverage(pints, hours)
Set highPints = getHigh(pints, hours)
Set lowPints = getLow(pints, hours)
Call displayInfo(hours, avePints, highPints, lowPints)
End Module
File Access and C++ Code
The goal of this lab is to convert the blood drive program from above to C++ code. Create a text file name bloodDrive.txt in the project folder with the following values (or you can also get it from Canvas). Make sure to put one blank line after the last value.
43
25
64
35
19
37
46
Run your program and check against the following output file, bloodResults.txt. If there are errors, go back through the steps to troubleshoot. You should confirm that the program displays 7, 43, and 46 to the screen for hours, first value, and last value.
Report generated by [YourName]
Blood drive period: 7
Average pints: 38.4
Highest pints: 64.0
Lowest pints: 19.0
You might want to try another test case with fewer or more than 7 values to make sure your program can handle it. Don’t forget to include <fstream> for file input and output. You would need to include <iomanip> and set the precision for the output file (not cout) to get one digit after the decimal point. When your code is complete and runs properly, copy/paste both the source code and the output file (bloodResults.txt) for the test case above.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

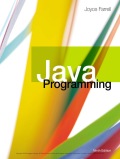
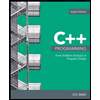
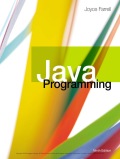
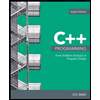