For this program, assume you have an input file with several lines of text. Each line has a student's last name, followed by a space and then 4 numbers which represent his/her grades. Your program should read this input file and produce an output file with the same number of lines, each line showing the last name and the average of the student's scores. "grades.txt" is the file your program should read. "badformat.txt" is an example of a file that your program can't read, so a clear error message should be generated, as shown in the sample output. Your program must catch all exceptions. Your main method must NOT have a "throws" clause - it must catch all exceptions and dea' with them appropriately. Other methods may throw exceptions, as long as the main method catches them. I don't expect you to examine any filenames to make sure they're valid. Instead, you should use the filename the user gives you and try to open a file by that name. That will throw an exception if the filename is invalid, and you should catch that exception. Do not assume there are 6 lines in the input file. Your program should work with any size file, stopping when the end of the file is reached. You may assume there are 4 scores per student, and that each student's data is on its own line. Use the named constant that I provided in the starter code: private static final int NUM_SCORES = 4; for the number of scores per student, to make your code more generalizable. However, if the input file doesn't fit this format, your program should not crash. Instead, you should handle the problem gracefully (maybe output a helpful error message and end the program). Like in the "Programming Exercise for File I/O: Employment Data", I recommend you use the String class's "split" method to help you parse the file. But for this program, instead of splitting the string lineIn on commas: lineIn.split(","), we are splitting on spaces: lineIn.split(" ") Please follow the standard conventions for indentation, meaningful variable names, etc. like the examples in class and in the textbook. Make sure to run your program to test it out (using replit.com or the compiler of your choice), and then submit your Main.java file including your sample output at the bottom, including your output file and the output displayed on the screen. Your job is to write a program to read the grades.txt file in this project, to produce output like shown here. "grades.txt" is the file your program should read. "badformat.txt" is an example of a file that your program can't read, so a clear error message should be generated, as shown in the sample output. */ import java.io.*; import java.util.Scanner; class Main { private static final int NUM_SCORES = 4; // named constant for the number of scores per student in file public static void main(String[] args) { } } badformat.txt file Biden 85 90 Trump 50 70 75 Obama 90 95 98 97 Bush 80 70 75 85 Clinton 90 95 85 99Bush 85 95 90 94 grades txt file Biden 85 90 95 98 Trump 50 70 75 80 Obama 90 95 98 97 Bush 80 70 75 85 Clinton 90 95 85 99 Bush 85 95 90 94 /* ***** Sample Output on Repl, hills, or other Linux (specifying an invalid path will throw an exception): ***** Remember that "cat" is a Linux command to display the contents of a text file on the screen. Welcome to the Grade Average Calculator. Please enter the name of a file to input: trash Unable to open file: trash Please enter the name of a file to input: grades.txt Enter name of output file to create: trash/trash Unable to open file: trash/trash Enter name of output file to create: gradeAvg.txt Average grades saved to gradeAvg.txt > cat grades.txt Biden 85 90 95 98 Trump 50 70 75 80 Obama 90 95 98 97 Bush 80 70 75 85 Clinton 90 95 85 99 Bush 85 95 90 94 > cat gradeAvg.txt Here are the average grades: Biden 92.0 Trump 68.8 Obama 95.0 Bush 77.5 Clinton 92.3 Bush 91.0 > java Main Welcome to the Grade Average Calculator. Please enter the name of a file to input: badformat.txt Enter name of output file to create: gradeAvg.txt The file badformat.txt isn't in the right format: it should have 1 name followed by 4 numbers on each line, separated by spaces. The following error was generated: 4 scores should follow each name, with spaces in between. Grades could not be averaged. > cat badformat.txt Biden 85 90 Trump 50 70 75 Obama 90 95 98 97 Bush 80 70 75 85 Clinton 90 95 85 99Bush 85 95 90 94 */
- For this program, assume you have an input file with several lines of text. Each line has a student's last name, followed by a space and then 4 numbers which represent his/her grades. Your program should read this input file and produce an output file with the same number of lines, each line showing the last name and the average of the student's scores.
- "grades.txt" is the file your program should read. "badformat.txt" is an example of a file that your program can't read, so a clear error message should be generated, as shown in the sample output.
- Your program must catch all exceptions. Your main method must NOT have a "throws" clause - it must catch all exceptions and dea' with them appropriately. Other methods may throw exceptions, as long as the main method catches them.
- I don't expect you to examine any filenames to make sure they're valid. Instead, you should use the filename the user gives you and try to open a file by that name. That will throw an exception if the filename is invalid, and you should catch that exception.
- Do not assume there are 6 lines in the input file. Your program should work with any size file, stopping when the end of the file is reached.
- You may assume there are 4 scores per student, and that each student's data is on its own line. Use the named constant that I provided in the starter code: private static final int NUM_SCORES = 4; for the number of scores per student, to make your code more generalizable. However, if the input file doesn't fit this format, your program should not crash. Instead, you should handle the problem gracefully (maybe output a helpful error message and end the program).
- Like in the "Programming Exercise for File I/O: Employment Data", I recommend you use the String class's "split" method to help you parse the file. But for this program, instead of splitting the string lineIn on commas: lineIn.split(","), we are splitting on spaces: lineIn.split(" ")
- Please follow the standard conventions for indentation, meaningful variable names, etc. like the examples in class and in the textbook.
- Make sure to run your program to test it out (using replit.com or the compiler of your choice), and then submit your Main.java file including your sample output at the bottom, including your output file and the output displayed on the screen.
Your job is to write a program to read the grades.txt file
in this project, to produce output like shown here.
"grades.txt" is the file your program should read. "badformat.txt" is an example of a file that your program can't read, so a clear error message should be generated, as shown in the sample output.
*/
import java.io.*;
import java.util.Scanner;
class Main
{
private static final int NUM_SCORES = 4;
// named constant for the number of scores per student in file
public static void main(String[] args)
{
}
}
badformat.txt file
Biden 85 90
Trump 50 70 75
Obama 90 95 98 97
Bush 80 70 75 85
Clinton 90 95 85 99Bush 85 95 90 94
grades txt file
Biden 85 90 95 98
Trump 50 70 75 80
Obama 90 95 98 97
Bush 80 70 75 85
Clinton 90 95 85 99
Bush 85 95 90 94
/* ***** Sample Output on Repl, hills, or other Linux
(specifying an invalid path will throw an exception): *****
Remember that "cat" is a Linux command to display the
contents of a text file on the screen.
Welcome to the Grade Average Calculator.
Please enter the name of a file to input:
trash
Unable to open file: trash
Please enter the name of a file to input:
grades.txt
Enter name of output file to create:
trash/trash
Unable to open file: trash/trash
Enter name of output file to create:
gradeAvg.txt
Average grades saved to gradeAvg.txt
> cat grades.txt
Biden 85 90 95 98
Trump 50 70 75 80
Obama 90 95 98 97
Bush 80 70 75 85
Clinton 90 95 85 99
Bush 85 95 90 94
> cat gradeAvg.txt
Here are the average grades:
Biden 92.0
Trump 68.8
Obama 95.0
Bush 77.5
Clinton 92.3
Bush 91.0
> java Main
Welcome to the Grade Average Calculator.
Please enter the name of a file to input:
badformat.txt
Enter name of output file to create:
gradeAvg.txt
The file badformat.txt isn't in the right format:
it should have 1 name followed by 4 numbers on each line,
separated by spaces. The following error was generated:
4 scores should follow each name, with spaces in between.
Grades could not be averaged.
> cat badformat.txt
Biden 85 90
Trump 50 70 75
Obama 90 95 98 97
Bush 80 70 75 85
Clinton 90 95 85 99Bush 85 95 90 94
*/

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

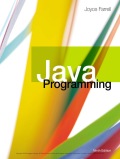
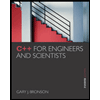
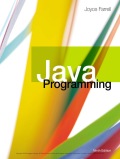
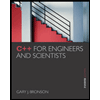