The class dateType was designed to implement the date in a program, but the member function setDate and the constructor do not check whether the date is valid before storing the date in the member variables. Rewrite the definitions of the function setDate and the constructor so that the values for the month, day, and year are checked before storing the date into the member variables. Add a member function, isLeapYear, to check whether a year is a leap year. Moreover, write a test program to test your class. Input should be format month day year with each separated by a space. If the year is a leap year, print the date and a message indicating it is a leap year, otherwise print a message indicating that it is not a leap year. Header file: #ifndef date_H #define date_H class dateType { public: void setDate(int month, int day, int year); //Function to set the date. //The member variables dMonth, dDay, and dYear are set //according to the parameters //Postcondition: dMonth = month; dDay = day; // dYear = year int getDay() const; //Function to return the day. //Postcondition: The value of dDay is returned. int getMonth() const; //Function to return the month. //Postcondition: The value of dMonth is returned. int getYear() const; //Function to return the year. //Postcondition: The value of dYear is returned. void printDate() const; //Function to output the date in the form mm-dd-yyyy. bool isLeapYear(); //Function to determine whether the year is a leap year. dateType(int month = 1, int day = 1, int year = 1900); //Constructor to set the date //The member variables dMonth, dDay, and dYear are set //according to the parameters //Postcondition: dMonth = month; dDay = day; // dYear = year //If no values are specified, the default values are //used to initialize the member variables. private: int dMonth; //variable to store the month int dDay; //variable to store the day int dYear; //variable to store the year }; #endif
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
C++
The class dateType was designed to implement the date in a program, but the member function setDate and the constructor do not check whether the date is valid before storing the date in the member variables. Rewrite the definitions of the function setDate and the constructor so that the values for the month, day, and year are checked before storing the date into the member variables. Add a member function, isLeapYear, to check whether a year is a leap year. Moreover, write a test program to test your class.
Input should be format month day year with each separated by a space.
If the year is a leap year, print the date and a message indicating it is a leap year, otherwise print a message indicating that it is not a leap year.
Header file:

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

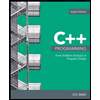
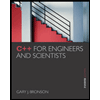
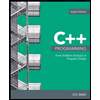
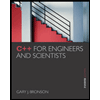