The code below is a C++ code for the istructions that follow this note. I have a issue with lines 53 and 54: static[runCount][0] = total; static[runCount][1] = avg; The = signs have the red error lines under them and I don't know why. If possible could you please find and correct what I did wrong and make sure it runs please. Thank you. Jason, Samantha, Ravi, Sheila, and Ankit are preparing for an upcoming marathon. Each day of the week, they run a certain number of miles and write them into a notebook. At the end of the week, they would like to know the number of miles run each day, the total miles for the week, and the average miles run each day. Write a program to help them analyze their data. Your program must contain parallel arrays: an array to store the names of the runners and a two-dimensional array of five rows and seven columns to store the number of miles run by each runner each day. Furthermore, your program must contain at least the following functions: a function to read and store the runners’ names and the numbers of miles run each day; a function to find the total miles run by each runner and the average number of miles run each day; and a function to output the results (You may assume that the input data is stored in a file and each line of data is in the following form: runnername milesday1 milesday2 milesday3 milesday4 milesday5 milesday6 milesday7.) #include #include #include #include using namespace std; const int COUNT = 5; const int DAYS = 7; void inputData(string names[], int sizeOfName, double numOfMiles[][DAYS], int rowSize) { ifstream infile; infile.open("Data.txt"); int checkNames = 0, checkMiles = 0; while (checkNames < sizeOfName) { infile >> names[checkNames]; while (checkMiles < DAYS) { infile >> numOfMiles[checkNames][checkMiles]; checkMiles++; } checkMiles = 0; checkNames++; } } void computeMiles(double status[][2], double numOfMiles [][DAYS], int rowSize) { double total = 0, avg = 0; int runCount = 0, num = 0; cout << fixed << showpoint << setprecision(2) << endl; while (runCount < rowSize) { while (num < DAYS) { total = total + numOfMiles[runCount][num]; num++; } avg = (total/DAYS); static[runCount][0] = total; //error = static[runCount][1] = avg; // error = total = 0; avg = 0; num = 0; runCount++; } } void displayResult(double status[][2], string names[], int sizeOfName) { cout << setw(10) << "Runner_Name" << setw(12) << "Total miles" << setw(10) << "Average" << endl; int runCount = 0; while (runCount < sizeOfName) { cout << setw(10) << names[runCount] << setw(12) << status[runCount][0] << setw(10) << status[runCount] [1] << endl; runCount++; } } int main() { string names[COUNT]; double milesStatus[COUNT][DAYS]; double output[COUNT][2]; inputData(names, 5, milesStatus, 5); computeMiles(output, milesStatus, 5); displayResult(output, names, 5); system("PAUSE"); return 0; }
The code below is a C++ code for the istructions that follow this note. I have a issue with lines 53 and 54:
static[runCount][0] = total;
static[runCount][1] = avg;
The = signs have the red error lines under them and I don't know why. If possible could you please find and correct what I did wrong and make sure it runs please. Thank you.
Jason, Samantha, Ravi, Sheila, and Ankit are preparing for an upcoming marathon. Each day of the week, they run a certain number of miles and write them into a notebook. At the end of the week, they would like to know the number of miles run each day, the total miles for the week, and the average miles run each day. Write a program to help them analyze their data. Your program must contain parallel arrays: an array to store the names of the runners and a two-dimensional array of five rows and seven columns to store the number of miles run by each runner each day. Furthermore, your program must contain at least the following functions: a function to read and store the runners’ names and the numbers of miles run each day; a function to find the total miles run by each runner and the average number of miles run each day; and a function to output the results (You may assume that the input data is stored in a file and each line of data is in the following form:
runnername milesday1 milesday2 milesday3 milesday4 milesday5 milesday6 milesday7.)
#include <iostream>
#include <fstream>
#include <iomanip>
#include <string>
using namespace std;
const int COUNT = 5;
const int DAYS = 7;
void inputData(string names[], int sizeOfName,
double numOfMiles[][DAYS], int rowSize)
{
ifstream infile;
infile.open("Data.txt");
int checkNames = 0, checkMiles = 0;
while (checkNames < sizeOfName)
{
infile >> names[checkNames];
while (checkMiles < DAYS)
{
infile >> numOfMiles[checkNames][checkMiles];
checkMiles++;
}
checkMiles = 0;
checkNames++;
}
}
void computeMiles(double status[][2], double numOfMiles
[][DAYS], int rowSize)
{
double total = 0, avg = 0;
int runCount = 0, num = 0;
cout << fixed << showpoint << setprecision(2) << endl;
while (runCount < rowSize)
{
while (num < DAYS)
{
total = total + numOfMiles[runCount][num];
num++;
}
avg = (total/DAYS);
static[runCount][0] = total; //error =
static[runCount][1] = avg; // error =
total = 0;
avg = 0;
num = 0;
runCount++;
}
}
void displayResult(double status[][2], string names[],
int sizeOfName)
{
cout << setw(10) << "Runner_Name" << setw(12)
<< "Total miles" << setw(10) << "Average" << endl;
int runCount = 0;
while (runCount < sizeOfName)
{
cout << setw(10) << names[runCount] << setw(12)
<< status[runCount][0] << setw(10) << status[runCount]
[1] << endl;
runCount++;
}
}
int main()
{
string names[COUNT];
double milesStatus[COUNT][DAYS];
double output[COUNT][2];
inputData(names, 5, milesStatus, 5);
computeMiles(output, milesStatus, 5);
displayResult(output, names, 5);
system("PAUSE");
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

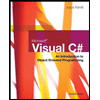
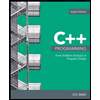
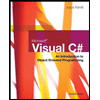
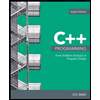