I have a syntax error with my program code. Can someone help me? Here's the instructions for my C++ program: Instructions Write a program that uses the function isPalindrome given in Example 6-6 (Palindrome). Test your program on the following strings:madam, abba, 22, 67876, 444244, trymeuemyrt Modify the function isPalindrome of Example 6-6 so that when determining whether a string is a palindrome, cases are ignored, that is, uppercase and lowercase letters are considered the same. The isPalindrome function from Example 6-6 has been included below for your convenience. bool isPalindrome(string str) {int length = str.length(); for (int i = 0; i < length / 2; i++) {if (str[i] != str[length – 1 – i]) {return false;} // if } // for loopreturn true;}// isPalindrome Your program should print a message indicating if a string is a palindrome: madam is a palindrome Here's what I have so far: #include <iostream>#include <string.h> using namespace std; //My user defined function that checks whether given string is palindromebool isPalindrome(string str){//Finding the string lengthint length = str.length(); //Iterating over stringfor (int i = 0; i < length / 2; i++){//Converting both characters into lower-case and then comparing them,//So now, the comparison function will ignore the caseif (tolower(str[i]) != tolower(str[length - 1 - i])) {return false;}}//String is a palindromereturn true;} //Define the main functionint main(){//Array of stringsstring s[7] = {"Madam", "22", "abBa", "67876", "444244", "trYmeuemyRT"};/st/.isPalindrome("Madam") #=> #<MatchData "st">/st/.isPalindrome("22") #=> #<MatchData "st">/st/.isPalindrome("abBa") #=> #<MatchData "st">/st/.isPalindrome("67876") #=> #<MatchData "st">/st/.isPalindrome("444244") #=> #<MatchData "st">/st/.isPalindrome("trYmeuemyRT") #<MatcData "st"> int i; //Checking five stringsfor (i = 0; i < 6; i++){//Testing the boolean isPalindrome user defined function against the string variables.if (isPalindrome(s[i])){cout << "\n " << s[i] << " is a palindrome";}else{cout << "\n " << s[i] << " is not a palindrome";} }return 0;} As you can see from my code, I've added 6 lines of regexp code that satisfies my lab environment's requirements. But it has to be placed in a different location in my program so it can execute properly. I'm unsure where to place them. Can someone help me?
I have a syntax error with my
Here's the instructions for my C++ program:
Instructions
Write a program that uses the
function isPalindrome given in
Example 6-6 (Palindrome). Test
your program on the following
strings:
madam, abba, 22, 67876, 444244, trymeuemyrt
Modify the function isPalindrome
of Example 6-6 so that when
determining whether a string is a
palindrome, cases are ignored, that
is, uppercase and lowercase letters
are considered the same.
The isPalindrome function from
Example 6-6 has been included
below for your convenience.
bool isPalindrome(string str)
{
int length = str.length();
for (int i = 0; i < length / 2; i++) {
if (str[i] != str[length – 1 – i]) {
return false;
} // if
} // for loop
return true;
}// isPalindrome
Your program should print a
message indicating if a string is a
palindrome:
madam is a palindrome
Here's what I have so far:
#include <iostream>
#include <string.h>
using namespace std;
//My user defined function that checks whether given string is palindrome
bool isPalindrome(string str)
{
//Finding the string length
int length = str.length();
//Iterating over string
for (int i = 0; i < length / 2; i++)
{
//Converting both characters into lower-case and then comparing them,
//So now, the comparison function will ignore the case
if (tolower(str[i]) != tolower(str[length - 1 - i])) {
return false;
}
}
//String is a palindrome
return true;
}
//Define the main function
int main()
{
//Array of strings
string s[7] = {"Madam", "22", "abBa", "67876", "444244", "trYmeuemyRT"};
/st/.isPalindrome("Madam") #=> #<MatchData "st">
/st/.isPalindrome("22") #=> #<MatchData "st">
/st/.isPalindrome("abBa") #=> #<MatchData "st">
/st/.isPalindrome("67876") #=> #<MatchData "st">
/st/.isPalindrome("444244") #=> #<MatchData "st">
/st/.isPalindrome("trYmeuemyRT") #<MatcData "st">
int i;
//Checking five strings
for (i = 0; i < 6; i++)
{
//Testing the boolean isPalindrome user defined function against the string variables.
if (isPalindrome(s[i]))
{
cout << "\n " << s[i] << " is a palindrome";
}
else
{
cout << "\n " << s[i] << " is not a palindrome";
}
}
return 0;
}
As you can see from my code, I've added 6 lines of regexp code that satisfies my lab environment's requirements. But it has to be placed in a different location in my program so it can execute properly. I'm unsure where to place them. Can someone help me?

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

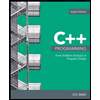
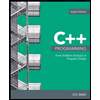