We are tasked stopping the program from printing negative numbers. How would I express that in Python? I don't need strings for every single converter. The program ideally would take note of the negative number. Then we have the program print a response and then terminate. MY PYTHON PROGRAM: #LAB 3 VERSION #Greeting the cousin and instructions. print( 'Hello cousin William! I have created a program \ to convert my U.S.measurements to your metric system!') # A simple folllow up to the greeting explaining how the sequential statements will work. print( '\nSimply type in the measurement when prompted \ by the sequence of conversions.') #MILES TO KM CONVERSION miles = float(input("William, enter the number of miles you'd like to convert to kilometers: ")) #The miles are prompted from the user. kilometers = miles * 1.6 # This is the basic conversion of miles to kilometers print("William, according to my calculations: \n%.2f Miles equals %.2f Kilometers " %(miles, kilometers)) #The output printed to the nearest 2 decimal places. #FARENHEIT TO CELSIUS farenheit = float(input("William, enter the farenheit temperature you'd like to convert to celsius: " )) #The F temperature is prompted for. celsius = (farenheit - 32) * 5 / 9 #The conversion function arithmetic. print("William, here is the result for you: \n%.2f farenheit to %.2f celsius" %(farenheit, celsius)) #The result of the previous function with the units tacked on at the end. #GALLONS TO LITERS Gal = float(input("William, enter the number of gallons you'd like converted to liters: " )) Li = Gal * 3.9 print("William, no wonder gas is so expensive in Europe. Look at this conversion: \n%.2f Gal to %.2f Li " %(Gal, Li)) #Lbs to Kg Lbs = float(input("William, enter the number of pounds you'd like converted to kilograms: " )) Kg = Lbs * 0.45 print("William, it is so easy converting these units. Look at this one: \n%.2f Lbs to %.2f Kg " %(Lbs, Kg)) #In to cm In = float(input("William, enter the number of inches you'd like converted to centimeters: " )) cm = In * 2.54 print("Ending this short length program with a length conversion: \n%.2f In to %.2f cm " %(In, cm)) print( 'What do you think William? It is quite handy!') #print message to conclude the program. #The verbiage makes it messy and harder to read. I could've prompted more line skips, but I chose not to.
We are tasked stopping the
How would I express that in Python?
I don't need strings for every single converter.
The program ideally would take note of the negative number.
Then we have the program print a response and then terminate.
MY PYTHON PROGRAM:
#LAB 3 VERSION
#Greeting the cousin and instructions.
print( 'Hello cousin William! I have created a program \
to convert my U.S.measurements to your metric system!')
# A simple folllow up to the greeting explaining how the sequential statements will work.
print( '\nSimply type in the measurement when prompted \
by the sequence of conversions.')
#MILES TO KM CONVERSION
miles = float(input("William, enter the number of miles you'd like to convert to kilometers: ")) #The miles are prompted from the user.
kilometers = miles * 1.6 # This is the basic conversion of miles to kilometers
print("William, according to my calculations: \n%.2f Miles equals %.2f Kilometers " %(miles, kilometers)) #The output printed to the nearest 2 decimal places.
#FARENHEIT TO CELSIUS
farenheit = float(input("William, enter the farenheit temperature you'd like to convert to celsius: " )) #The F temperature is prompted for.
celsius = (farenheit - 32) * 5 / 9 #The conversion function arithmetic.
print("William, here is the result for you: \n%.2f farenheit to %.2f celsius" %(farenheit, celsius)) #The result of the previous function with the units tacked on at the end.
#GALLONS TO LITERS
Gal = float(input("William, enter the number of gallons you'd like converted to liters: " ))
Li = Gal * 3.9
print("William, no wonder gas is so expensive in Europe. Look at this conversion: \n%.2f Gal to %.2f Li " %(Gal, Li))
#Lbs to Kg
Lbs = float(input("William, enter the number of pounds you'd like converted to kilograms: " ))
Kg = Lbs * 0.45
print("William, it is so easy converting these units. Look at this one: \n%.2f Lbs to %.2f Kg " %(Lbs, Kg))
#In to cm
In = float(input("William, enter the number of inches you'd like converted to centimeters: " ))
cm = In * 2.54
print("Ending this short length program with a length conversion: \n%.2f In to %.2f cm " %(In, cm))
print( 'What do you think William? It is quite handy!') #print message to conclude the program.
#The verbiage makes it messy and harder to read. I could've prompted more line skips, but I chose not to.

Step by step
Solved in 3 steps with 2 images

I don't think you're understanding my dilemma.
I am not ALLOWED to use exit functions.
Yes, I know they work, but the professor said to NOT use them.
I'll be more clear again:
DO NOT use loops, functions, list, a menu etc…) In addition break, quit, exit,
sys.exit, return, continue, or any other shortcut ion NEVER allowed.
The other thought I had was with def main function, but I AM NOT ALLOWED TO USE EXIT FUNCTIONS, otherwise this would be a very easy
The exit function still persists and does not logically exit. I don't understand.
My apologies, I did not specify enough.
I got the
I assume this has to do with the indentation.
We are also no permitted to use EXIT functions.
We need to utilize: Boolean Logic, If, If-Else, If-Elif-Else Control Structure.
Would I utilize an if else statement like
PSEUDOCODE:
If value<0
print(NO NEG NUMBERS ALLOWED)
else value>0
then use the code I had previously?
I don't know how to make the program logically exit without exit functions.
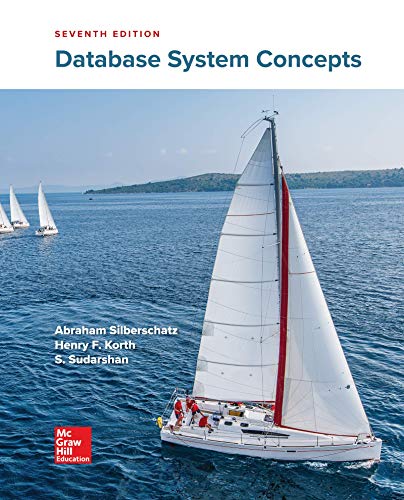
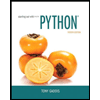
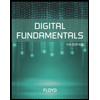
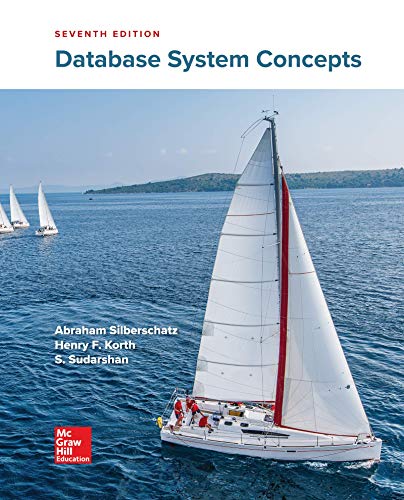
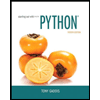
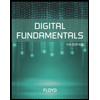
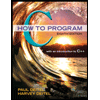
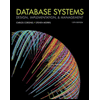
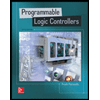