The program below uses pointer arithmetic to determine the size of a 'char' variable. By using pointer arithmetic we can find out the value of 'cp' and the value of 'cp+1'. Since cp is a pointer, this addition involves pointer arithmetic: adding one to a pointer makes the pointer point to the next element of the same type. For a pointer to a char, adding 1 really just means adding 1 to the address, but this is only because each char is 1 byte. 1. Compile and run the program and see what it does. 2. Write some code that does pointer arithmetic with a pointer to an int and determine how big an int is. 3. Same idea – figure out how big a double is, by using pointer arithmetic and printing out the value of the pointer before and after adding 1. 4. What should happen if you added 2 to the pointers from exercises 1 through 3, instead of 1? Use your program to verify your answer. #include int main( ) { char c = 'Z'; char *cp = &c; printf("cp is %p\n", cp); printf("The character at cp is %c\n", *cp); /* Pointer arithmetic - see what cp+1 is */ cp = cp+1; printf("cp is %p\n", cp); /* Do not print *cp, because it points to memory not allocated to your program */ return 0; }
The
variable. By using pointer arithmetic we can find out the value of 'cp' and the
value of 'cp+1'. Since cp is a pointer, this addition involves pointer arithmetic:
adding one to a pointer makes the pointer point to the next element of the same
type.
For a pointer to a char, adding 1 really just means adding 1 to the address, but
this is only because each char is 1 byte.
1. Compile and run the program and see what it does.
2. Write some code that does pointer arithmetic with a pointer to an int and
determine how big an int is.
3. Same idea – figure out how big a double is, by using pointer arithmetic and
printing out the value of the pointer before and after adding 1.
4. What should happen if you added 2 to the pointers from exercises 1
through 3, instead of 1? Use your program to verify your answer.
#include <stdio.h>
int main( )
{
char c = 'Z';
char *cp = &c;
printf("cp is %p\n", cp);
printf("The character at cp is %c\n", *cp);
/* Pointer arithmetic - see what cp+1 is */
cp = cp+1;
printf("cp is %p\n", cp);
/* Do not print *cp, because it points to
memory not allocated to your program */
return 0;
}

Step by step
Solved in 5 steps with 8 images

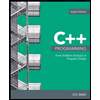
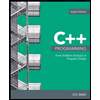