The program should Compute an investment report based on the paycheck amount from program #1 by doing the following: 1. Modify Program #1 and Provided code for Program #2: - Remove the paycheck display part from the code of program 1 (so that you leave only netpay to pass it to program #2 - Modify the program 2 to accept the netpay from program 1 as the startBalance for investment - set the number of years to 4 years - set the interest rate at 6% (an integer percent) 3. The report is displayed in tabular form with a header (see output below) 4. Computations and outputs: - For each year ▪ compute the interest and add it to the investment ▪ print a formatted row of results for that year 5. The total investment and interest earned are also displayed (format the output according the example below Program 1: def calcWeeklyWages(totalHours, hourlyWage): # return the total weekly wages for a worked working totalHours, # with a given regular hourlyWage. Include overtime for hours over 40 if totalHours <= 40: totalWages = hourlyWage*totalHours print("you had no overtime hours this week") else: overtime = totalHours - 40 totalWages = hourlyWage*40 + (1.5*hourlyWage)*overtime print("your overtime hours this week are: ", overtime, "hours") return totalWages # main program (that you will need to modify) hours_in_week1 = float(input('Enter hours worked in first: ')) wage_in_week1 = float(input('Enter dollars paid per hour: ')) week1_earned = calcWeeklyWages(hours_in_week1, wage_in_week1) hours_in_week2 = float(input('Enter hours worked in second week: ')) wage_in_week2 = float(input('Enter dollars paid per hour in second week: ')) week2_earned = calcWeeklyWages(hours_in_week2, wage_in_week2) # Modification below: # 1. Modify the main program section by creating new user defined functions called weeklypay() # your weeklypay() will prompt the user to enter hours for this week, # set the wage to 15 and call calcWeeklyWages(totalHours, hourlyWage) # then return the total pay for that week # 2. To calculate the weekly pay for week 1 and week 2, call weeklypay() twice (for week 1 & week 2) print('Wages_in_week1 for {hours_in_week1} hours at ${wage_in_week1:.2f} per hour are ${week1_earned:.2f}.'.format(**locals())) print('Wages_in_week2 for {hours_in_week2} hours at ${wage_in_week2:.2f} per hour are ${week2_earned:.2f}.'.format(**locals())) # 3. Add the wages from week 1 and week 2 into a variable called paycheck_amount total_hours=hours_in_week1+hours_in_week2 total_earned=week1_earned+week2_earned print('total pay earned for two weeks {total_hours} hours at wages_in_week1 ${wage_in_week1:.2f} and at wages_in_week2 ${wage_in_week2:.2f} per hour are ${total_earned:.2f}.'.format(**locals())) # 4. Calculate the income tax amount that your employer withhold from your paycheck # by multiplying paycehck amount * .18 (18 percent) amount_of_taxes =total_earned*18/100 print('amount of taxes withheld based on 18% of the {total_earned:.2f}. is {amount_of_taxes:.2f}'.format(**locals())) # 5. Calculate the net pay by taking the income tax amount off the paycheck amount # 6. Finally, display the earned pay (paycheck amount), tax amount and net pay amount # based on the output example that you will see print('earned pay is:',total_earned) print('taxes withheld:',amount_of_taxes) print('net pay is:',total_earned-amount_of_taxes); Program 2: provided code # Accept the inputs from the user startBalance = float(input("Enter the investment amount: ")) years = int(input("Enter the number of years: ")) rate = int(input("Enter the rate as a %: ")) # Convert the rate to a decimal number rate = rate / 100 # Initialize the accumulator for the interest totalInterest = 0.0 # Display the header for the table print("%4s%18s%10s%16s" % \ ("Year", "Starting balance", "Interest", "Ending balance")) # Compute and display the results for each year for year in range(1, years + 1): interest = startBalance * rate endBalance = startBalance + interest print("%4d%18.2f%10.2f%16.2f" % \ (year, startBalance, interest, endBalance)) startBalance = endBalance totalInterest += interest # Display the totals for the period print("Ending balance: $%0.2f" % endBalance) print("Total interest earned: $%0.2f" % totalInterest)
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
The
1. Modify Program #1 and Provided code for Program #2:
- Remove the paycheck display part from the code of program 1 (so that you leave only netpay to pass it to program #2
- Modify the program 2 to accept the netpay from program 1 as the startBalance for investment
- set the number of years to 4 years
- set the interest rate at 6% (an integer percent)
3. The report is displayed in tabular form with a header (see output below)
4. Computations and outputs:
- For each year
▪ compute the interest and add it to the investment
▪ print a formatted row of results for that year
5. The total investment and interest earned are also displayed (format the output according the example below
Program 1:
def calcWeeklyWages(totalHours, hourlyWage):
# return the total weekly wages for a worked working totalHours,
# with a given regular hourlyWage. Include overtime for hours over 40
if totalHours <= 40:
totalWages = hourlyWage*totalHours
print("you had no overtime hours this week")
else:
overtime = totalHours - 40
totalWages = hourlyWage*40 + (1.5*hourlyWage)*overtime
print("your overtime hours this week are: ", overtime, "hours")
return totalWages
# main program (that you will need to modify)
hours_in_week1 = float(input('Enter hours worked in first: '))
wage_in_week1 = float(input('Enter dollars paid per hour: '))
week1_earned = calcWeeklyWages(hours_in_week1, wage_in_week1)
hours_in_week2 = float(input('Enter hours worked in second week: '))
wage_in_week2 = float(input('Enter dollars paid per hour in second week: '))
week2_earned = calcWeeklyWages(hours_in_week2, wage_in_week2)
# Modification below:
# 1. Modify the main program section by creating new user defined functions called weeklypay()
# your weeklypay() will prompt the user to enter hours for this week,
# set the wage to 15 and call calcWeeklyWages(totalHours, hourlyWage)
# then return the total pay for that week
# 2. To calculate the weekly pay for week 1 and week 2, call weeklypay() twice (for week 1 & week 2)
print('Wages_in_week1 for {hours_in_week1} hours at ${wage_in_week1:.2f} per hour are ${week1_earned:.2f}.'.format(**locals()))
print('Wages_in_week2 for {hours_in_week2} hours at ${wage_in_week2:.2f} per hour are ${week2_earned:.2f}.'.format(**locals()))
# 3. Add the wages from week 1 and week 2 into a variable called paycheck_amount
total_hours=hours_in_week1+hours_in_week2
total_earned=week1_earned+week2_earned
print('total pay earned for two weeks {total_hours} hours at wages_in_week1 ${wage_in_week1:.2f} and at wages_in_week2 ${wage_in_week2:.2f} per hour are ${total_earned:.2f}.'.format(**locals()))
# 4. Calculate the income tax amount that your employer withhold from your paycheck
# by multiplying paycehck amount * .18 (18 percent)
amount_of_taxes =total_earned*18/100
print('amount of taxes withheld based on 18% of the {total_earned:.2f}. is {amount_of_taxes:.2f}'.format(**locals()))
# 5. Calculate the net pay by taking the income tax amount off the paycheck amount
# 6. Finally, display the earned pay (paycheck amount), tax amount and net pay amount
# based on the output example that you will see
print('earned pay is:',total_earned)
print('taxes withheld:',amount_of_taxes)
print('net pay is:',total_earned-amount_of_taxes);
Program 2: provided code
# Accept the inputs from the user
startBalance = float(input("Enter the investment amount: "))
years = int(input("Enter the number of years: "))
rate = int(input("Enter the rate as a %: "))
# Convert the rate to a decimal number
rate = rate / 100
# Initialize the accumulator for the interest
totalInterest = 0.0
# Display the header for the table
print("%4s%18s%10s%16s" % \
("Year", "Starting balance",
"Interest", "Ending balance"))
# Compute and display the results for each year
for year in range(1, years + 1):
interest = startBalance * rate
endBalance = startBalance + interest
print("%4d%18.2f%10.2f%16.2f" % \
(year, startBalance, interest, endBalance))
startBalance = endBalance
totalInterest += interest
# Display the totals for the period
print("Ending balance: $%0.2f" % endBalance)
print("Total interest earned: $%0.2f" % totalInterest)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

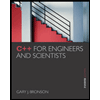
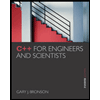