The project contains three (3) arrays already declared and initialized. You need to implement the following fivemethods in the sections indicated by the comments: Method printo Parameter: An array of integers and number of elements.o Prints out all the elements of the array on the same line and separated by a space. o See format in the sample output shown below. Method printLargesto Parameter: An array of integers and number of elements.o Use a FOR loop to find the largest element in the array.o Call the method print to print out the entire array.o Print the largest element as shown in the sample output. Method printAllEvenso Parameter: An array of integers and number of elements.o Call the method print to print out the entire array.o Use a FOR loop to print out all the even numbers in the array. Numbers should be on the sameline and separated by a space.o See format in the sample output shown below. Method printSumo Parameter: An array of integers and number of elements.o Call the method print to print out the entire array.o Use a FOR loop to calculate the sum of all numbers in the array. o Output the final result in the format shown in the sample output below. Method searcho Parameters: An array of integers, the number of elements, and an integer to search for.o Call the method print to print out the entire array.o Use a WHILE loop to find the element.o The while loop should STOP when the element is found or when the search has reached theend of the array.o Output the final result in the format shown in the sample output below.Thus, you will actually implement 5 void methods. The method print is called by the other 4 and these 4 arecalled by the main method several times each as indicated by the starter project and the sample output below.(Thus, you will not call the method print directly from the main method.)The method main has comments to guide you in implementing the function calls. In the main method: Do NOT add any additional System.out.print statements. Do NOT add any additional code other than the function calls that are specified in the comments.(See next page for sample output.)Sample Output:Array: 23 43 12 45 75 32 12Largest element: 75Array: 34 54 23 34 98 45 76 98 42 48 61 44Largest element: 98Array: 69 62 28 75 28 99 20 45 34 75Largest element: 99Array: 23 43 12 45 75 32 12Even numbers: 12 32 12Array: 34 54 23 34 98 45 76 98 42 48 61 44Even numbers: 34 54 34 98 76 98 42 48 44Array: 69 62 28 75 28 99 20 45 34 75Even numbers: 62 28 28 20 34Array: 23 43 12 45 75 32 12Sum: 242Array: 34 54 23 34 98 45 76 98 42 48 61 44Sum: 657Array: 69 62 28 75 28 99 20 45 34 75Sum: 535Array: 23 43 12 45 75 32 12Number 100 is not in the array.Array: 34 54 23 34 98 45 76 98 42 48 61 44Number 44 is in the array.Array: 69 62 28 75 28 99 20 45 34 75Number 78 is not in the array.Array: 23 43 12 45 75 32 12Number 45 is in the array https://coastdistrict.instructure.com/courses/67490/files/5564580/download?wrap=1
The project contains three (3) arrays already declared and initialized. You need to implement the following five
methods in the sections indicated by the comments:
Method print
o Parameter: An array of integers and number of elements.
o Prints out all the elements of the array on the same line and separated by a space.
o See format in the sample output shown below.
Method printLargest
o Parameter: An array of integers and number of elements.
o Use a FOR loop to find the largest element in the array.
o Call the method print to print out the entire array.
o Print the largest element as shown in the sample output.
Method printAllEvens
o Parameter: An array of integers and number of elements.
o Call the method print to print out the entire array.
o Use a FOR loop to print out all the even numbers in the array. Numbers should be on the same
line and separated by a space.
o See format in the sample output shown below.
Method printSum
o Parameter: An array of integers and number of elements.
o Call the method print to print out the entire array.
o Use a FOR loop to calculate the sum of all numbers in the array.
o Output the final result in the format shown in the sample output below.
Method search
o Parameters: An array of integers, the number of elements, and an integer to search for.
o Call the method print to print out the entire array.
o Use a WHILE loop to find the element.
o The while loop should STOP when the element is found or when the search has reached the
end of the array.
o Output the final result in the format shown in the sample output below.
Thus, you will actually implement 5 void methods. The method print is called by the other 4 and these 4 are
called by the main method several times each as indicated by the starter project and the sample output below.
(Thus, you will not call the method print directly from the main method.)
The method main has comments to guide you in implementing the function calls. In the main method:
Do NOT add any additional System.out.print statements.
Do NOT add any additional code other than the function calls that are specified in the comments.
(See next page for sample output.)
Sample Output:
Array: 23 43 12 45 75 32 12
Largest element: 75
Array: 34 54 23 34 98 45 76 98 42 48 61 44
Largest element: 98
Array: 69 62 28 75 28 99 20 45 34 75
Largest element: 99
Array: 23 43 12 45 75 32 12
Even numbers: 12 32 12
Array: 34 54 23 34 98 45 76 98 42 48 61 44
Even numbers: 34 54 34 98 76 98 42 48 44
Array: 69 62 28 75 28 99 20 45 34 75
Even numbers: 62 28 28 20 34
Array: 23 43 12 45 75 32 12
Sum: 242
Array: 34 54 23 34 98 45 76 98 42 48 61 44
Sum: 657
Array: 69 62 28 75 28 99 20 45 34 75
Sum: 535
Array: 23 43 12 45 75 32 12
Number 100 is not in the array.
Array: 34 54 23 34 98 45 76 98 42 48 61 44
Number 44 is in the array.
Array: 69 62 28 75 28 99 20 45 34 75
Number 78 is not in the array.
Array: 23 43 12 45 75 32 12
Number 45 is in the array
https://coastdistrict.instructure.com/courses/67490/files/5564580/download?wrap=1

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

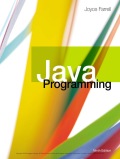
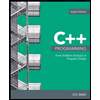
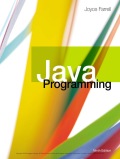
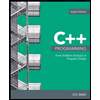