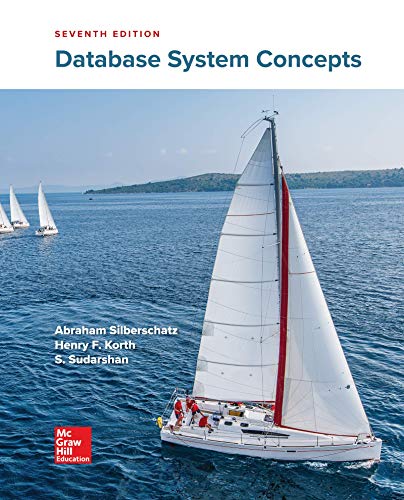
How to fix this code to work...
from tkinter import *
from tkinter import ttk
from tkinter import messagebox
class Student:
def __init__(self, id, fn, ln, dob, m='undefined'):
self.id = id
self.firstName = fn
self.lastName = ln
self.dateOfBirth = dob
self.major = m
list_of_student = []
window = Tk()
window.geometry("800x750")
window.title("Simple Student Management System")
list_of_students = []
id = 1001
def add_student():
global list_of_students
global id
if FirstnameEntry.get() =='':
messagebox.showerror('erorr', "First name is required")
return
if LastnameEntry.get() =='':
messagebox.showerror('erorr', "Last name is required")
return
if DateofbirthEntry.get() =='':
messagebox.showerror('erorr', "Date of Birth is required")
return
if MajorEntry.get() =='':
instance = Student(id, FirstnameEntry.get(), LastnameEntry.get(), DateofbirthEntry.get())
else:
instance = Student(id, FirstnameEntry.get(), LastnameEntry.get(), DateofbirthEntry.get(), MajorEntry.get())
list_of_students.append(instance)
messagebox.showinfo("Information", "Student added")
id += 1
FirstnameEntry.delete(0, END)
LastnameEntry.delete(0, END)
DateofbirthEntry.delete(0, END)
MajorEntry.delete(0, END)
def viewAll():
global list_of_students
t.delete("1.0", END)
for obj in list_of_students:
t.insert(END, Student.print_student_info())
t.insert(END, '\n')
if len(list_of_students) ==0:
messagebox.showerror("error", "No Student Found")
def search_student():
found = False
for student in list_of_students:
if SearchbyNameEntry.get() == Student.first_name or SearchbyNameEntry.get() == Student.last_name:
found = True
t.insert(END, Student.print_student_info())
if (found==False):
messagebox.showerror("error", "No Student Found")
def remove_student():
found = False
for student in list_of_students:
if RemovebyNameEntry.get() ==str(student.id):
found = True
list_of_students.remove(student)
if (found==False):
messagebox.showerror("error", "No Student Found")
else:
messagebox.showinfo("Information", "Student Removed")
Label(text = "First Name").grid(row=1, column = 0, padx=90)
Label(text = "Last Name").grid(row=2, column = 0)
Label(text = "Date of Birth").grid(row=3, column = 0)
Label(text = "Major").grid(row=4, column = 0)
FirstnameValue = StringVar()
LastnameValue = StringVar()
DateofbirthValue = StringVar()
MajorValue = StringVar()
SearchbyNameValue = StringVar()
RemovebyNameValue = StringVar()
ViewallValue = StringVar()
FirstnameEntry = Entry(window, textvariable=FirstnameValue)
LastnameEntry = Entry(window, textvariable=LastnameValue)
DateofbirthEntry = Entry(window, textvariable=DateofbirthValue)
MajorEntry = Entry(window, textvariable=MajorValue)
SearchbyNameEntry = Entry(window, textvariable=SearchbyNameValue)
RemovebyNameEntry = Entry(window, textvariable=RemovebyNameValue)
ViewallEntry=Entry(window, textvariable=ViewallValue)
FirstnameEntry.grid(row=1, column=1, pady=10)
LastnameEntry.grid(row=2, column=1, pady=10)
DateofbirthEntry.grid(row=3, column=1, pady=10)
MajorEntry.grid(row=4, column=1, pady=10)
b1= Button(text="Add Student", command= add_student)
b1.grid(row=5, column =1, pady=10)
ttk.Separator(window, orient=HORIZONTAL).grid(row=7, columnspan=3, ipadx=400, pady=10)
b2 = Button(text="Search by name", command=search_student)
b2.grid(row=8, column=0, pady=5, padx=0)
SearchbyNameEntry.grid(row=8, column=0, columnspan=2, pady=5)
b3 = Button(text="View All", command=viewAll)
b3.grid(row=8, column=1, pady=5)
t = Text(window, width=70, height=30)
t.grid(column=0, row=9, columnspan=2, padx=20)
ttk.Separator(window, orient=HORIZONTAL).grid(row=50, columnspan=3, ipadx=400, pady=10)
b4 = Button(text="Remove ID", command=remove_student)
b4.grid(row=52, column=0, pady=5, padx=0)
b4.grid(row=52, column=0, columnspan=2, pady=5)
window.mainloop()

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 7 images

- C++ You should create a loop which will ask user if they want to insert, delete, display and exit. Then call the corresponding method based on the user's input. The loop will only stop once user entered "Exit" Topic: LinkedListarrow_forwardComputer Science JAVA #7 - program that reads the file named randomPeople.txt sort all the names alphabetically by last name write all the unique names to a file named namesList.txt , there should be no repeatsarrow_forwardGiven the previous Car class, the following members have been added for you: Private: string * parts; //string array of part names int num_parts; //number of parts Public: void setParts(int numpart, string newparts[]) //set the numparts and parts int getNumParts() string * getParts() string getPart(int index) //return the string in parts at index num Please implement the following for the Car class: Add the copy constructor. (deep copy!) Add the copy assignment operator. (deep copy!) Add the destructorarrow_forward
- A for construct is a loop construct that processes a specified list of objects. As a result,it is executed as long as there are remaining objects to process. True or False?arrow_forwardAssignment Submission Instructions:This is an individual assignment – no group submissions are allowed. Submit a script file that contains the SELECT statements by assigned date. The outline of the script file lists as follows:/* ******************************************************************************** * Name: YourNameGoesHere * * Class: CST 235 * * Section: * * Date: * * I have not received or given help on this assignment: YourName * ***********************************************************************************/USE RetailDB;####### Tasks: Write SQL Queries ######### -- Task 1 (Customer Information):-- List your SELECT statement below. Make sure the SQL script file can be run successfully in MySQL and show the outcome of the code on MySQLarrow_forwardin java #6 - program that reads the name data from the files named firstNames.txt and lastNames.txtand produces a list of 1000 random names randomPeople.txtone complete name (firstname lastname) per linearrow_forward
- 个 O codestepbystep.com/problem/view/java/loops/ComputeSumOfDigits?problemsetid=4296 You are working on problem set: HW2- loops (Pause) ComputeSumOfDigits ♡ Language/Type: % Related Links: Java interactive programs cumulative algorithms fencepost while Scanner Write a console program in a class named ComputeSumOfDigits that prompts the user to type an integer and computes the sum of the digits of that integer. You may assume that the user types a non-negative integer. Match the following output format: Type an integer: 827184 Digit sum is 22 JE J @ C % 123 A 2 7 8 9 Class: Write a complete Java class. Need help? Stuck on an exercise? Contact your TA or instructor If something seems wrong with our site, please contact us. Submit t US Oct 13 9:00 Aarrow_forwardPython Programmingarrow_forwardPart 5. Operator Overload: add Next, you will add the ability to use the addition operator (+) in conjunction with Simpy objects and floats. You will implement add such that the left-hand side operand of an addition expression can be either a simpy object or a float value using a Union type. The add_method should return a new Simpy object and should not mutate the object the method is called on. When the right-hand side of an addition expression is also a simpy object, you should assert that both objects' values attributes have equal lengths. Then, you should produce a new simpy object where each item in its values attribute corresponds to the items of the original Simpy objects at the same index added together. For example: a = Simpy([1.0, 1.0, 1.0]) b = Simpy([2.0, 3.0, 4.0]) c = a + b print(c) # Output: Simpy([3.0, 4.0, 5.0]) When the right-hand side of an addition expression is a float value, you should produce a new simpy object where each item corresponds to the item at the same…arrow_forward
- dictionaries = [] dictionaries.append({"First":"Bob", "Last":"Jones"}) dictionaries.append({"First":"Harpreet", "Last":"Kaur"}) dictionaries.append({"First":"Mohamad", "Last":"Argani"}) for i in range(0, len(dictionaries)): print(dictionaries[i]['First']) ************************************************* please modity the above code to "Add an ‘if’ statement(s) to the loop in your solution in Exercise 7 to only show the full name if the first name is “Bob” or if the last name is “Kaur”.arrow_forwardInterval Difference: Please help me with this assertion errorarrow_forwardHELP WITH JAVA PLEASE PROVIDE INDENTED CODES SO I CAN COPY N PASTE MAKE SURE UR OUTPUT COMPILES FINE AND IN A GUI PANEL. TAKE A SCREENSHOT OF OUR OUTPUT AND PASTE HERE AS WELL ALSO, For project 2 you need to create a class for the list nodes, RomanNumeralListNode DO THE FOLLOWING:arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
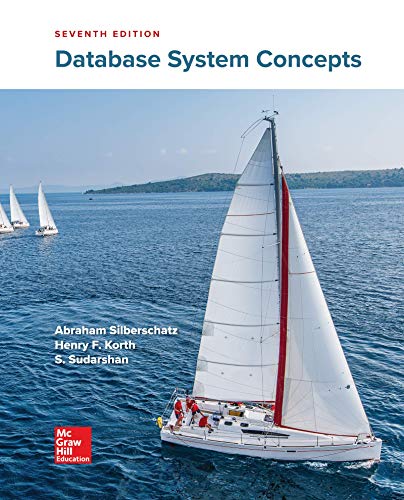
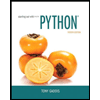
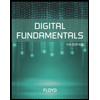
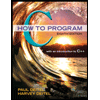
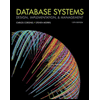
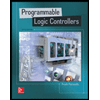