This assignment involves the stack data structure and file processing A common application of stacks is the parsing and evahuation of arithmetic expressions. Compilers use stacks to check if the brackets in an arithmetic expression are balanced. For example, the expression [ (a-b) * (5+4)]/(g/h) is balanced in the sense that for each matching left bracket, there is a matching right bracket. On the other hand, the expression [ (a-b) )1" is not balanced because the mumber of left C is not equal to the number of right ). Also, an expression like { (a+x}) would be incorrect because the inner C should be closed with a )' first before outer '{" is closed with the '}'. Your task is to write a program that reads an input text file consisting of a list of anithmetic expressions, one expression on each line. Each arithmetic expression may consist of three kinds of brackpts, namely, (). [ and { }. There could either be spaces or no space between the brackets and arithmetic operators and variables. As an example, your input text file could be the following (5 + { a (b - [c + d] [e/f ]) (x+y ) } i [ I {( (x+y+z ) ) } } 1 1 [a-b ] *[c+d ] /[e- i * (g+h ) { 2+8} ( ( foo + bo0)) {m + (g/h ) } (y + (a/b } )* (z+y) } {x + 6 | (a + x } ) (p + (g - ( I x + Y) 1 (a* (b (c * d) 1 ) ) [ { (x+y) 1 (2 + 3 ) } ) Your program should read each line and check if it is balanced with respect to the three symbols. For this, you should use a stack. You may use any of the three Stack classes (Stack as an array, Stack as an arraylist or Stack as a linked list) that was discussed in the lectures. Your program should report whether each line is correct or incorrect. The output for the above example is given below. It would be displayed on the screen, not to a file. (5 + { a (b - [c + d] [e/1 ] ) (*+y ) } [ [ { ( ( (x+y+z ) ) } } ] ] [a-b ] *[c+d ] /[e- (g+h ) *{ 2+8} ( ( foo + boo) ) (m + (g/h ) } (x + 6 (y + (a/b } )* (z+y) } { (a + x }) (p + (g - (2 + 3) } ) ([ x + y) 1 (a* (b * (c * d) 1 ) ) [ { (x+y) 1 → correct → correct → correct → correct → correct → correct → incorrect → incorrect > incorrect → incorrect → incorrect Note: You need not check the validity of the expression with respect to other parameters, such as addition, subtraction, multiplication, division, etc. Just check if the brackets are balanced.
This assignment involves the stack data structure and file processing A common application of stacks is the parsing and evahuation of arithmetic expressions. Compilers use stacks to check if the brackets in an arithmetic expression are balanced. For example, the expression [ (a-b) * (5+4)]/(g/h) is balanced in the sense that for each matching left bracket, there is a matching right bracket. On the other hand, the expression [ (a-b) )1" is not balanced because the mumber of left C is not equal to the number of right ). Also, an expression like { (a+x}) would be incorrect because the inner C should be closed with a )' first before outer '{" is closed with the '}'. Your task is to write a program that reads an input text file consisting of a list of anithmetic expressions, one expression on each line. Each arithmetic expression may consist of three kinds of brackpts, namely, (). [ and { }. There could either be spaces or no space between the brackets and arithmetic operators and variables. As an example, your input text file could be the following (5 + { a (b - [c + d] [e/f ]) (x+y ) } i [ I {( (x+y+z ) ) } } 1 1 [a-b ] *[c+d ] /[e- i * (g+h ) { 2+8} ( ( foo + bo0)) {m + (g/h ) } (y + (a/b } )* (z+y) } {x + 6 | (a + x } ) (p + (g - ( I x + Y) 1 (a* (b (c * d) 1 ) ) [ { (x+y) 1 (2 + 3 ) } ) Your program should read each line and check if it is balanced with respect to the three symbols. For this, you should use a stack. You may use any of the three Stack classes (Stack as an array, Stack as an arraylist or Stack as a linked list) that was discussed in the lectures. Your program should report whether each line is correct or incorrect. The output for the above example is given below. It would be displayed on the screen, not to a file. (5 + { a (b - [c + d] [e/1 ] ) (*+y ) } [ [ { ( ( (x+y+z ) ) } } ] ] [a-b ] *[c+d ] /[e- (g+h ) *{ 2+8} ( ( foo + boo) ) (m + (g/h ) } (x + 6 (y + (a/b } )* (z+y) } { (a + x }) (p + (g - (2 + 3) } ) ([ x + y) 1 (a* (b * (c * d) 1 ) ) [ { (x+y) 1 → correct → correct → correct → correct → correct → correct → incorrect → incorrect > incorrect → incorrect → incorrect Note: You need not check the validity of the expression with respect to other parameters, such as addition, subtraction, multiplication, division, etc. Just check if the brackets are balanced.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 1TF
Related questions
Question
Quickly
![This assignment involves the stack data structure and file processing. A common application of stacks is
the parsing and evaluation of arithmetic expressions. Compilers use stacks to check if the brackets in an
arithmetic expression are balanced. For example, the expression
[ (a-b) * (5+4)1/(g/h)
is balanced in the sense that for each matching left bracket, there is a matching right bracket. On the other
hand, the expression
[ (a-b) )1"
is not balanced because the mumber of left C is not equal to the number of right )'. Also, an expression
like
{ (a+x}) would be incorrect because the imner ( should be closed with a )' first before outer (* is
closed with the }'.
Your task is to write a program that reads an input text file consisting of a list of anthmetic expressions,
one expression on each line. Each arithmetic expression may consist of three kinds of brackets, namely, ().
[ ] and { }. There could either be spaces or no space between the brackets and arithmetic operators and
variables. As an example, your input text file could be the following:
(5 + { a * (b -
[ [{ { ( (x+y+z ) ) } } ] ]
[a-b ] * [c+d ] / [e-f ] * (g+h ) *{ 2+8}
(( foo + bo0) )
{m +
[c + d] * [e/f ] ) } (x+y ) }
(g/h ) }
(x + 6 * (y + (a/b } ) * (z+y) }
{ (a + x } )
(p + (g -
( [ x + y) ]
( a * (b * (c * d ) ]) )
[ { (x+y) 1
(2 + 3 ) } )
Your program should read each line and check if it is balanced with respect to the three symbols. For this,
you should use a stack. You may use any of the three Stack classes (Stack as an array, Stack as an arraylist
or Stack as a linked list) that was discussed in the lectures. Your program should report whether each line is
correct or incorrect. The output for the above example is given below. It would be displayed on the screen,
not to a file.
(5 + { a * (b - [c + d ] * [e/E ]) }* (x+y ) }
[ [ {{ ( (x+y+z ) ) } } ] ]
[a-b ] * [c+d ] /[e-f ] * (g+h ) *{ 2+8}
(( foo + bo0) )
{m +
(x + 6 * (y + (a/b } ) * (z+y) }
(a + x } )
(p + (g
( [ x + y) ]
→ correct
→ correct
> correct
→ correct
→ correct
→ correct
> incorrect
> incorrect
→ incorrect
→ incorrect
→ incorrect
(g/h ) }
{2 + 3 ) } )
(a *
(b * (c * d )))
[ { (x+y) ]
Note: You need not check the validity of the expression with respect to other parameters, such as addition,
subtraction, multiplication, division, etc. Just check if the brackets are balanced.
Submit the following:
1. Source code of the program.
2. Stack class source code.
3. Sample text input file.
4. Output](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F324d9bd6-050d-4d64-a836-5319c4147a10%2Fe4ff87e7-f3d7-4125-b28b-bd0d6f68a5a0%2Fm35rrke_processed.jpeg&w=3840&q=75)
Transcribed Image Text:This assignment involves the stack data structure and file processing. A common application of stacks is
the parsing and evaluation of arithmetic expressions. Compilers use stacks to check if the brackets in an
arithmetic expression are balanced. For example, the expression
[ (a-b) * (5+4)1/(g/h)
is balanced in the sense that for each matching left bracket, there is a matching right bracket. On the other
hand, the expression
[ (a-b) )1"
is not balanced because the mumber of left C is not equal to the number of right )'. Also, an expression
like
{ (a+x}) would be incorrect because the imner ( should be closed with a )' first before outer (* is
closed with the }'.
Your task is to write a program that reads an input text file consisting of a list of anthmetic expressions,
one expression on each line. Each arithmetic expression may consist of three kinds of brackets, namely, ().
[ ] and { }. There could either be spaces or no space between the brackets and arithmetic operators and
variables. As an example, your input text file could be the following:
(5 + { a * (b -
[ [{ { ( (x+y+z ) ) } } ] ]
[a-b ] * [c+d ] / [e-f ] * (g+h ) *{ 2+8}
(( foo + bo0) )
{m +
[c + d] * [e/f ] ) } (x+y ) }
(g/h ) }
(x + 6 * (y + (a/b } ) * (z+y) }
{ (a + x } )
(p + (g -
( [ x + y) ]
( a * (b * (c * d ) ]) )
[ { (x+y) 1
(2 + 3 ) } )
Your program should read each line and check if it is balanced with respect to the three symbols. For this,
you should use a stack. You may use any of the three Stack classes (Stack as an array, Stack as an arraylist
or Stack as a linked list) that was discussed in the lectures. Your program should report whether each line is
correct or incorrect. The output for the above example is given below. It would be displayed on the screen,
not to a file.
(5 + { a * (b - [c + d ] * [e/E ]) }* (x+y ) }
[ [ {{ ( (x+y+z ) ) } } ] ]
[a-b ] * [c+d ] /[e-f ] * (g+h ) *{ 2+8}
(( foo + bo0) )
{m +
(x + 6 * (y + (a/b } ) * (z+y) }
(a + x } )
(p + (g
( [ x + y) ]
→ correct
→ correct
> correct
→ correct
→ correct
→ correct
> incorrect
> incorrect
→ incorrect
→ incorrect
→ incorrect
(g/h ) }
{2 + 3 ) } )
(a *
(b * (c * d )))
[ { (x+y) ]
Note: You need not check the validity of the expression with respect to other parameters, such as addition,
subtraction, multiplication, division, etc. Just check if the brackets are balanced.
Submit the following:
1. Source code of the program.
2. Stack class source code.
3. Sample text input file.
4. Output
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
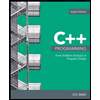
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
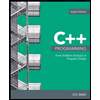
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning