#include using namespace std; #define MAX 1000 //Maximum size of stack class Stack{ int top;// to store top of stack public: int elements[MAX]; //Integer array to store elements Stack(){ //class constructor for initialization top=-1; } bool push(int x) { if(top>=(MAX-1)) //Condition for top when stack because full { cout<<"Stack is full\n"; return false; } else { ++top; //Increasing top value elements[top]=x; //storing it to element in to stack return true; } } int pop(){ if(top<0)// Condition for top when stack is empty { cout<<"stack is empty\n"; return INT_MIN; } else { int x=elements[top--];//poping out the element for stack by decreasing to element return x; } } int display(){ if(top<0)//Condition for top when stack is empty { cout<<"Stack is empty\n"; return INT_MIN; } else{ for(int i=0;i<=top;i++)//running loop from start to top and printing elements cout<>ch; if(ch==1){ int x; cout<<"Enter an integer element to push:\n"; cin>>x; mystack.push(x); } else if(ch==2){ int x=mystack.pop(); if(x!=INT_MIN) cout<<"Element"<>newch; if(newch=='n') break; } return 0; }
#include<bits/stdc++.h>
using namespace std;
#define MAX 1000 //Maximum size of stack
class Stack{
int top;// to store top of stack
public:
int elements[MAX]; //Integer array to store elements
Stack(){ //class constructor for initialization
top=-1;
}
bool push(int x) {
if(top>=(MAX-1)) //Condition for top when stack because full
{
cout<<"Stack is full\n";
return false;
}
else
{
++top; //Increasing top value
elements[top]=x; //storing it to element in to stack
return true;
}
}
int pop(){
if(top<0)// Condition for top when stack is empty
{
cout<<"stack is empty\n";
return INT_MIN;
}
else
{
int x=elements[top--];//poping out the element for stack by decreasing to element
return x;
}
}
int display(){
if(top<0)//Condition for top when stack is empty
{
cout<<"Stack is empty\n";
return INT_MIN;
}
else{
for(int i=0;i<=top;i++)//running loop from start to top and printing elements
cout<<elements[i]<<"";
cout<<endl;
return 1;
}
}
};
int main()
{
cout<<"1.push\n 2. Pop\n 3. Display\n";
char newch;
int ch;
Stack mystack;//Stack class object
while(1){//running loop till ‘n’ is not entered.
cout<<"Enter your choice:\n";
cin>>ch;
if(ch==1){
int x;
cout<<"Enter an integer element to push:\n";
cin>>x;
mystack.push(x);
}
else if(ch==2){
int x=mystack.pop();
if(x!=INT_MIN)
cout<<"Element"<<x<<"popped out\n";
}
else if(ch==3){
mystack.display();
}
else
cout<<"!!!Wrong Choice entered!!!\n";
cout<<"Do you want to continue:\n";
cin>>newch;
if(newch=='n')
break;
}
return 0;
}
Can you give me the output result screenshot for this code representing every operation and satisfying the problem statement.
![Problem Statement: We can store k stacks in a single array if we use the data structure suggested
in Figure 1 shown below, for the case k = 3. We push and pop from each stack as suggested in
connection with Figure 2 below. However, if pushing onto stack i causes TOP(i) to equal
BOTTOM(i – 1), we first move all the stacks so that there is an appropriate size gap between each
adjacent pair of stacks. For example, we might make the gaps above all stacks equal, or we might
make the gap above stack i proportional to the current size of stack i (on the theory that larger
stacks are likely to grow sooner, and we want to postpone as long as possible the next
reorganization).
1
stack 1
3
stack 2
bottom
1
2
stack 3
3
top
stackspace
Figure 1
top
first element
second element
maxlength
last element
elements
Figure 2
1. On the assumption that there is a procedure reorganize to call when stacks collide, write
code for the five stack operations.
2. On the assumption that there is a procedure MakeNewTops that computes newtop[i], the
"appropriate" position for the top of stack i, for 1 <i<k, write the procedure reorganize.
Hint. Note that stack i could move up or down, and it is necessary to move stack i before
stack j if the new position of stack j overlaps the old position of stack i. Consider stacks 1,
2,..., k in order, but keep a stack of "goals," each goal being to move a particular stack.
If on considering stack i, we can move it safely, do so, and then reconsider the stack whose
number is on top of the goal stack. If we cannot safely move stack i, push i onto the goal
stack.
3. What is an appropriate implementation for the goal stack in (2)? Do we really need to keep
it as a list of integers, or will a more succinct representation do?
4. Implement MakeNewTops in such a way that space above each stack is proportional to the
current size of that stack.
The implementation of this stack management software should be as structured as possible.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Feddfdae4-4eb1-4173-94bc-7dfd3da3807a%2Ff02bb28b-b7ba-46db-bb52-7977749f0066%2F0fgoikv_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

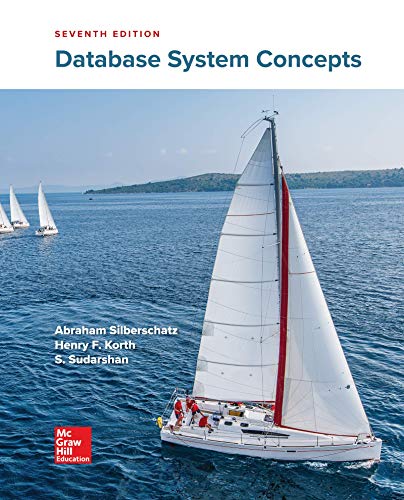
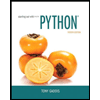
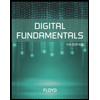
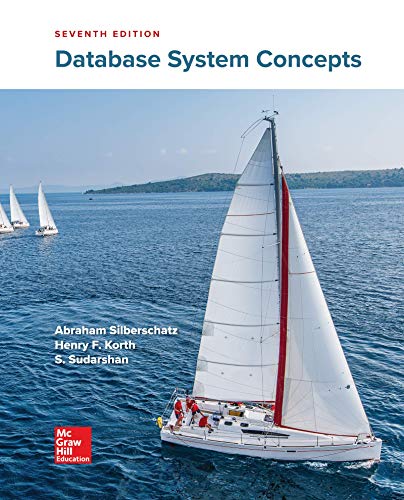
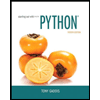
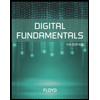
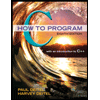
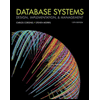
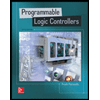