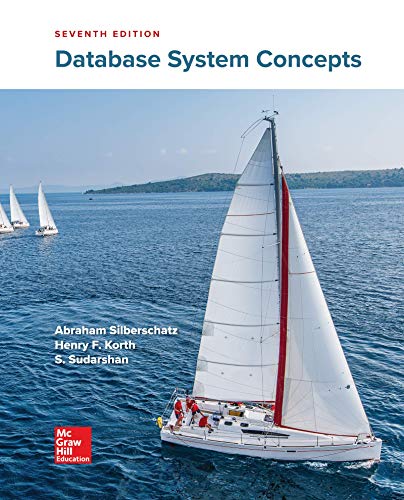
This assignment will require you to implement a generic interface (Queue<T>) with an array based queue implementation class QueueArray<T>. floating front design.
Once you have implemented the class, the test program will run a client test program on the enqueue, dequeue, display and full methods. The test program will create queues of Integers as well as String objects. Enjoying the power of your generic implementation both will work with no additional changes.
There are TODO based comments in the implementation file. Create a project and demonstrate that you can successfully create the required output file, shown below.
Thr file includes:
Queue.java
package queueHw;
public interface Queue <T>
{
public boolean empty();
public boolean full();
public boolean enqueue(T data);
public T dequeue();
public void display();
}
...
TestQueue.java
package queueHw;
public class TestQueue {
public static void main(String[] args) {
//TEST QueueArray with Int
System.out.println("-- testing with QueueArray with INT-- ");
Queue<Integer> qi2 = new QueueArray<>();
TEST_QUEUE_WITH_INT(qi2);
System.out.println("--------- ");
System.out.println("--------- ");
//TEST QueueArray with Int
System.out.println("-- testing with QueueArray with STRING-- ");
Queue<String> qs2 = new QueueArray<>();
TEST_QUEUE_WITH_STRING(qs2);
System.out.println("--------- ");
System.out.println("--------- ");
}
public static void TEST_QUEUE_WITH_INT(Queue<Integer> q) {
q.enqueue(3);
q.enqueue(4);
q.enqueue(8);
q.enqueue(0);
q.enqueue(2);
q.enqueue(9);
q.display();
while (!q.empty()) {
q.dequeue();
q.display();
}
System.out.printf("%n");
for (int i = 2; i < 23; i += 2)
q.enqueue(i);
q.display();
while (!q.empty()) {
q.dequeue();
q.display();
}
System.out.printf("%n");
for (int i = 21; i < 60; i += 2) {
q.enqueue(i);
try {
Thread.currentThread().sleep(125);
} catch (InterruptedException ie) {
}
q.display();
}
}
public static void TEST_QUEUE_WITH_STRING(Queue<String> qs) {
String[] tokens = { "hello", "bye", "green", "house", "desk", "freedom", "element", "automobile" };
qs.display();
for (String str : tokens) {
qs.enqueue(str);
qs.display();
try {
Thread.currentThread().sleep(125);
} catch (InterruptedException ie) {
}
}
System.out.println();
while (!qs.empty()) {
qs.dequeue();
qs.display();
try {
Thread.currentThread().sleep(125);
} catch (InterruptedException ie) {
}
}
}
}
...
QueueArray.java

Implementation of the QueueArray class that implements the Queue interface, with TODOs replaced with appropriate code:
public class QueueArray<T> implements Queue<T> {
private int front;
private int rear;
private final int MAX_SIZE = 100;
private T[] data;
private int numElements = 0;
public QueueArray() {
data = (T[]) new Object[MAX_SIZE];
front = 0;
rear = -1;
}
@Override
public boolean empty() {
return numElements == 0;
}
@Override
public boolean full() {
return numElements == MAX_SIZE;
}
@Override
public boolean enqueue(T el) {
if (full()) {
return false;
}
if (empty()) {
front = 0;
}
rear = bump(rear);
data[rear] = el;
numElements++;
return true;
}
@Override
public T dequeue() {
if (empty()) {
return null;
}
T removedElement = data[front];
if (numElements == 1) {
front = 0;
rear = -1;
} else {
front = bump(front);
}
numElements--;
return removedElement;
}
@Override
public void display() {
if (empty()) {
System.out.println("[ ]");
} else if (numElements == 1) {
System.out.println("[ " + data[front] + " ]");
} else {
System.out.print("[ ");
int i = front;
while (true) {
System.out.print(data[i] + " ");
if (i == rear) {
break;
}
i = bump(i);
}
System.out.print("] ");
System.out.println(" ");
}
}
private int bump(int index) {
return (index + 1) % data.length;
}
}
Step by stepSolved in 2 steps with 1 images

- Modify the simulate() method in the Simulator class to work with the revised interface of ParkingLot and the new peek() method in Queue. In addition, implement the getIncomingQueueSize() method of Simulator using the size() method of Queue. The getIncomingQueueSize() is going to be used in the CapacityOptimizer class (next task) to determine the size of the incoming queue after a simulation run. CODE TO MODIFY IN JAVA: public class Simulator { /** * Length of car plate numbers */ public static final int PLATE_NUM_LENGTH = 3; /** * Number of seconds in one hour */ public static final int NUM_SECONDS_IN_1H = 3600; /** * Maximum duration a car can be parked in the lot */ public static final int MAX_PARKING_DURATION = 8 * NUM_SECONDS_IN_1H; /** * Total duration of the simulation in (simulated) seconds */ public static final int SIMULATION_DURATION = 24 * NUM_SECONDS_IN_1H; /** * The probability distribution for a car leaving the lot based on the duration * that the car has been parked in…arrow_forwardJava. Please use the template in the picture, thank you!arrow_forwardI have already done number 1. I just need help with number 2. Implement Stack<E> with an internal LineNodePlus<E>. You have been given the code for the StackADT<E> interface and all of the List files needed. You must come up with a new Stack.java that implements the StackADT interface and uses a LineNodesPlus internally to store the stack. Construct test cases for the Stack (StackTest.java) to ensure it works correctly. StackADT.java public interface StackADT<E>{ // Stack class ADT /** * Clears the stack. */ public void clear(); /** * Pushes argument it onto the stack. Argument is stored in the top of the * stack. Returns false if the stack is out of space. * * @param it value to be pushed onto the stack * @return true if the value is stored */ public boolean push(E it); /** * Pop and return the value at the top of the stack. * * @return value stored at the top of the stack */ public E…arrow_forward
- In python. Write a LinkedList class that has recursive implementations of the add and remove methods. It should also have recursive implementations of the contains, insert, and reverse methods. The reverse method should not change the data value each node holds - it must rearrange the order of the nodes in the linked list (by changing the next value each node holds). It should have a recursive method named to_plain_list that takes no parameters (unless they have default arguments) and returns a regular Python list that has the same values (from the data attribute of the Node objects), in the same order, as the current state of the linked list. The head data member of the LinkedList class must be private and have a get method defined (named get_head). It should return the first Node in the list (not the value inside it). As in the iterative LinkedList in the exploration, the data members of the Node class don't have to be private. The reason for that is because Node is a trivial class…arrow_forwardUse a SinglyLinked List to implement a Queuea. Define a Queue interface.b. Define a LinkedQueue class (Node class)..c. Define a Test class to test all methods please help me make a test class first code package LinkedQueue; import linkedstack.Node; public class SLinkedList<E> { private Node <E> head = null; private Node <E> tail = null; private int size = 0; public SLinkedList() { head = null; tail = null; size = 0; } public int getSize() { return size; } public boolean isEmpty() { return size == 0; } public E getFirst() { if(isEmpty()) { return null; } return head.getElement(); } public E getLast() { if(isEmpty()) { return null; } return tail.getElement(); } public void addFirst(E e) { Node<E> newest = new Node<>(e, null); newest.setNext(head); head = newest; if(getSize()==0) { tail = head; } size++; } public void addLast(E e) { Node<E> newest = new Node<>(e, null); if(isEmpty()) { head = newest; } else {…arrow_forwardJava Code: Below is Parser.java and there are errors. getType() and getStart() is undefined for the type Optional<Token> and there is an error in addNode(). Make sure to get rid of all the errors in the code. Attached is images of the errors. Parser.java import java.text.ParseException;import java.util.LinkedList;import java.util.List;import java.util.Optional; import javax.swing.ActionMap; public class Parser { private TokenHandler tokenHandler; private LinkedList<Token> tokens; public Parser(LinkedList<Token> tokens) { this.tokenHandler = new TokenHandler(tokens); this.tokens = tokens; } public boolean AcceptSeparators() { boolean foundSeparator = false; while (tokenHandler.MoreTokens()) { Optional<Token> currentToken = tokenHandler.getCurrentToken(); if (currentToken.getType() == Token.TokenType.NEWLINE || currentToken.getType() == Token.TokenType.SEMICOLON) {…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
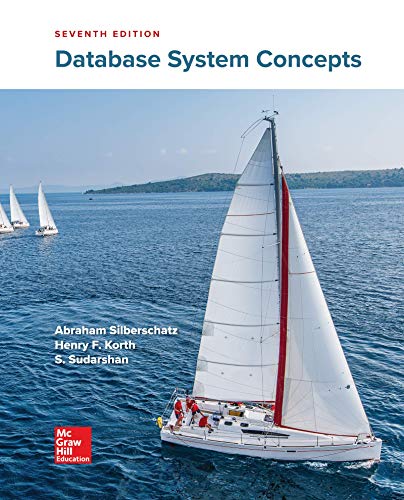
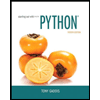
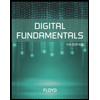
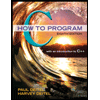
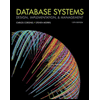
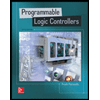