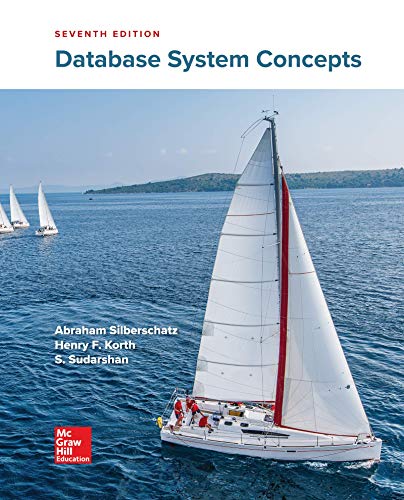
Your task is to: Implement the LinkedList interface ( fill out the implementation shell). Put your implementation through its paces by exercising each of the methods in the test harness. Create a client ( a class with a main ) ‘StagBusClient’ which builds a bus route by performing the following operations on your linked list:
- Create (insert) 4 stations
- List the stations
- Check if a station is in the list (print result). Check for a station that exists, and one that doesn’t
- Remove a station. List the stations
- Add a station before another station. List the stations
- Add a station after another station. Print the stations
LinkedList.java
package linkedList;
public interface LinkedList {
public Boolean isItemInList(String thisItem);
// true if it is, false if not
public Boolean addItem(String thisItem);
// true if added, false if it was already there, or an error
public Integer itemCount();
public void listItems();
public Boolean deleteItem(String thisItem);
// true if deleted, false if not there or error
public Boolean insertBefore(String newItem, String itemToInsertBefore);
public Boolean insertAfter(String newItem, String itemToInsertAfter);
//ExtraCredit
public void sort();
// ascending alphanumeric sort; nothing fancy but ALTERS THE LIST, DOES NOT COPY.
}
// Implement this interface using class ListItem
// Also implement the tester in this package.
LinkedListImpl.java
package linkedList;
public class LinkedListImpl implements LinkedList {
}
LinkedListTester.java
package linkedList;
public class LinkedListTester {
public static void main(String[] args) {
// create implementation, then...
}
}
ListItem.java
package linkedList;
public class ListItem {
public String data;
public ListItem next;
public ListItem(String data) {
this.data = data;
this.next = null;
}
}

Implement the LinkedList interface ( fill out the implementation shell). Put your implementation through its paces by exercising each of the methods in the test harness. Create a client ( a class with a main ) ‘StagBusClient’ which builds a bus route by performing the following operations on your linked list:
- Create (insert) 4 stations
- List the stations
- Check if a station is in the list (print result). Check for a station that exists, and one that doesn’t
- Remove a station. List the stations
- Add a station before another station. List the stations
- Add a station after another station. Print the stations
LinkedList:
LinkedList is a linear data structure that consists of nodes and links that connect these nodes. Each node in a linked list contains a data element and a reference to the next node in the list. The first node in the list is called the head, and the last node is called the tail. Linked lists can be singly linked, where each node has only a reference to the next node or doubly linked, where each node has a reference to both the next and previous nodes.
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Implement a part of functionality for the Netflix DVD queue. It's a service that allows a user to create a list of desired movies and then sends DVDs with movies on top of this list to the subscriber one at a a time. A subscriber should be able to create a list of desired movies and manipulate the order in a movie queue in their account. Your program will implement some of the desired functionality by storing the list of movie titles in a linked list. You are provided with the following files available in "Downloadable files" section: • MovieList.h contains a class declaration for the class that represents a list of movies. • Driver.cpp contains a main function you can use to test your implementation. You will be responsible for providing the MovieList.cpp file, including the implementation of the MovieList member functions (described below): MovieList and ~MovieList: creates an empty list, and deallocates all the nodes in the list, respectively. display(ostream& out) Print movie…arrow_forwardGiven main.py and an IntNode class, complete the IntList class (a linked list of IntNodes) by writing the insert_in_descending_order() method to insert new IntNodes into the IntList in descending order. Ex. If the input is: 3 4 2 5 1 6 7 9 8 the output is: 9 8 7 6 5 4 3 2 1 main.py(can not edit) from IntNode import IntNodefrom IntList import IntList if __name__ == "__main__": int_list = IntList() input_line = input() input_strings = input_line.split(' ') for num_string in input_strings: # Convert from string to integer num = int(num_string) # Insert into linked list in descending order new_node = IntNode(num) int_list.insert_in_descending_order(new_node) int_list.print_int_list() IntNode.py(can not edit) class IntNode: def __init__(self, initial_data, next = None, prev = None): self.data = initial_data self.next = next self.prev = prev IntList.py(need to edit)arrow_forwardGiven the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 #include "MileageTrackerNode.h"#include <string>#include <iostream>using namespace std; int main (int argc, char* argv[]) {// References for MileageTrackerNode objectsMileageTrackerNode* headNode;MileageTrackerNode* currNode;MileageTrackerNode* lastNode; double miles;string date;int i; // Front of nodes listheadNode = new MileageTrackerNode();lastNode = headNode; // TODO: Read in the number of nodes // TODO: For the read in number of nodes, read// in data and insert into the linked list // TODO: Call the PrintNodeData() method// to print the entire linked list //…arrow_forward
- Implement a linked list of integers as aclass LinkedList. Build the following methods:✓ print that prints the content of the linked list;✓ addFirst that adds a new node to the beginning (the head) of thelinked list;✓ addLast that adds a new node to the end (the tail) of the linked list;✓ indexOf that finds a specific node by its value, and returns node’sindex (node’s position from the left in the linked list); if the valueis not present in the linked list, it returns −1;✓ deleteFirst that deletes the first node in the linked list;✓ deleteLast that deletes the last node in the linked list.Test your class creating a list in the main and1) adding one by one nodes 2, 4, 8 to the tail;2) adding nodes -2, -8 to the head;3) adding a node 9 to the tail;4) printing the list;5) printing indexOf(4);6) printing contains(9);7) deleting one by one all the nodes in the list – either from the tail orfrom the head – and printing the result after each deletion. THEN AFTER THIS Add a new method IN THE…arrow_forwardGiven the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user- input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 7/16/18 5arrow_forwardEach of the iterators must implement the java.util.Iterator interface by providing the two public methods hasNext () and next(). The Iterator interface also lists two additional methods, remove () and forEachRemaining (). These are marked as default, so the interface itself does not require implementation. Of these methods, this assignment only requires you to implement the remove () method of the linked list iterator. 1 ArrayList iterator This is an external iterator, meaning it is a public class named ICS211ArrayListIterator, and and the code is not part of the ArrayList class. Your ICS211ArrayListIterator class should have a constructor ICS211ArrayListIterator(java.util.ArrayList gives access to all the elements of the array list. This array must be saved as the value of a class variable of the iterator. data). The constructor must call one of the two toArray methods of ArrayList to obtain an array that Other than this single call to java.util.ArrayList.toArray in the constructor,…arrow_forward
- In python. Write a LinkedList class that has recursive implementations of the add and remove methods. It should also have recursive implementations of the contains, insert, and reverse methods. The reverse method should not change the data value each node holds - it must rearrange the order of the nodes in the linked list (by changing the next value each node holds). It should have a recursive method named to_plain_list that takes no parameters (unless they have default arguments) and returns a regular Python list that has the same values (from the data attribute of the Node objects), in the same order, as the current state of the linked list. The head data member of the LinkedList class must be private and have a get method defined (named get_head). It should return the first Node in the list (not the value inside it). As in the iterative LinkedList in the exploration, the data members of the Node class don't have to be private. The reason for that is because Node is a trivial class…arrow_forwardCreate an implementation of each LinkedList, Queue Stack interface provided For each implementation create a tester to verify the implementation of thatdata structure performs as expected Your task is to: Implement the LinkedList interface ( fill out the implementation shell). Put your implementation through its paces by exercising each of the methods in the test harness Create a client ( a class with a main ) ‘StagBusClient’ which builds a bus route by performing the following operations on your linked list: Create (insert) 4 stations List the stations Check if a station is in the list (print result) Check for a station that exists, and one that doesn’t Remove a station List the stations Add a station before another station. List the stations Add a station after another station. Print the stations StagBusClient.java package app; import linkedList.LinkedList; import linkedList.LinkedListImpl; public class StagBusClient { public static void main(String[] args) { // create…arrow_forwardI don't get the "//To be implemented" comments, what am I supposed to do?Also, are the header files and header files implementation different? Like is the linkedList.h different than the linkedList.h (implementation)?arrow_forward
- Given main.py and a PersonNode class, complete the PersonList class by writing find_first() and find_last() methods at the end of the PersonList.py file. The find_first() method should find the first occurrence of an age value in the linked list and return the corresponding node. Similarly, the find_last() method should find the last occurrence of the age value in the linked list and return the corresponding node. For both methods, if the age value is not found, None should be returned. The program will replace the name value of each found node with a new name. Ex. If the input is: Alex 23 Tom 41 Michelle 34 Vicky 23 -1 23 Connor 34 Michela main.py(can not edit) from PersonNode import PersonNodefrom PersonList import PersonList if __name__ == "__main__": person_list = PersonList() # Read input lines until encountering '-1' input_line = input() while input_line != '-1': split_input = input_line.split(' ') name = split_input[0] age =…arrow_forwardGiven the source code of linked List, answer the below questions(image): A. Fill out the method printList that print all the values of the linkedList: Draw the linked list. public void printList() { } // End of print method B. Write the lines to insert 10 at the end of the linked list. You must draw the final linked List. Notice that you can’t use second or third nodes. Feel free to define a new node. Assume you have only a head node C. Write the lines to delete node 2. You must draw the final linked list. Notice that you can’t use second or third node. Feel free to define a new node. Assume you have only a head nodearrow_forwardExplain the differences between GenericServlet and HttpServlet, two Java classes.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
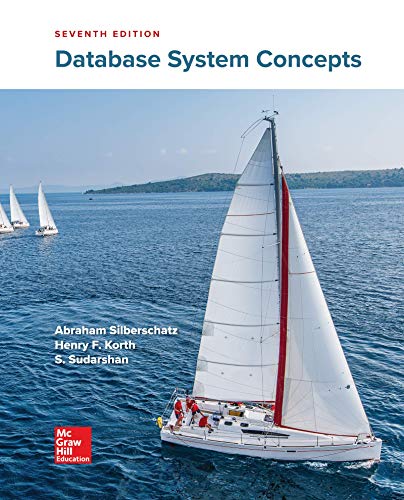
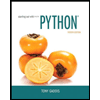
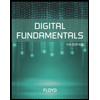
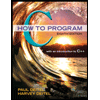
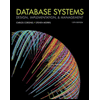
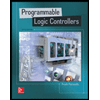