This C++ program accept 10 integers range in between 10 and 15 from a user. If the user enters an integer that is out of the range, an error message appears and the integer will not be stored. All the in- range integers will be stored in an array. The program then counts the frequency of each integer that stores in the array.
This C++ program accept 10 integers range in between 10 and 15 from a user. If the user enters an integer that is out of the range, an error message appears and the integer will not be stored. All the in- range integers will be stored in an array. The program then counts the frequency of each integer that stores in the array.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section: Chapter Questions
Problem 6PP: (Numerical) a. Define an array with a maximum of 20 integer values, and fill the array with numbers...
Related questions
Question
Need this urgent. Thank you
![Question 1
This C++ program accept 10 integers range in between 10 and 15 from a user. If the user enters an
integer that is out of the range, an error message appears and the integer will not be stored. All the in-
range integers will be stored in an array. The program then counts the frequency of each integer that
stores in the array.
The main function is given below. The program calls getInteger (...) function to prompt user to
enter integer numbers and store them into the respective element of the array. It then calls
countFrequency (...) function to compute the frequencies of each integer in the array. Lastly,
displayoutput (...) function is called to display the output on screen.
int main() {
// array declaration
int countArr[6] = {0}, numArr[10];
getInteger (numArr);
countFrequency (numArr, countArr);
displayoutput (countArr);
return 0;
Sample Output
Enter an integer between 10 and 15: 10
Enter an integer between 10 and 15: 10
Enter an integer between 10 and 15: 12
Enter an integer between 10 and 15: 13
Enter an integer between 10 and 15: 8
ERROR: Integer must between 10 and 15
Enter an integer between 10 and 15: 14
Enter an integer between 10 and 15: 15
Enter an integer between 10 and 15: 16
ERROR: Integer must between 10 and 15
Enter an integer between 10 and 15: 15
Enter an integer between 10 and 15: 15
Enter an integer between 10 and 15: 10
Enter an integer between 10 and 15: 12
The frequency of the integer:
Integer 10: 3
Integer 1l: 0
Integer 12: 2
Integer 13: 1
Integer 14: 1
Integer 15: 3
Note: Blue color indicates the user key-in value.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6d59576f-f278-4c8b-89f8-d4efdfea3ed1%2F9df279c2-7d57-42c5-ad86-bc0e3df428b0%2Fbvagacw_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Question 1
This C++ program accept 10 integers range in between 10 and 15 from a user. If the user enters an
integer that is out of the range, an error message appears and the integer will not be stored. All the in-
range integers will be stored in an array. The program then counts the frequency of each integer that
stores in the array.
The main function is given below. The program calls getInteger (...) function to prompt user to
enter integer numbers and store them into the respective element of the array. It then calls
countFrequency (...) function to compute the frequencies of each integer in the array. Lastly,
displayoutput (...) function is called to display the output on screen.
int main() {
// array declaration
int countArr[6] = {0}, numArr[10];
getInteger (numArr);
countFrequency (numArr, countArr);
displayoutput (countArr);
return 0;
Sample Output
Enter an integer between 10 and 15: 10
Enter an integer between 10 and 15: 10
Enter an integer between 10 and 15: 12
Enter an integer between 10 and 15: 13
Enter an integer between 10 and 15: 8
ERROR: Integer must between 10 and 15
Enter an integer between 10 and 15: 14
Enter an integer between 10 and 15: 15
Enter an integer between 10 and 15: 16
ERROR: Integer must between 10 and 15
Enter an integer between 10 and 15: 15
Enter an integer between 10 and 15: 15
Enter an integer between 10 and 15: 10
Enter an integer between 10 and 15: 12
The frequency of the integer:
Integer 10: 3
Integer 1l: 0
Integer 12: 2
Integer 13: 1
Integer 14: 1
Integer 15: 3
Note: Blue color indicates the user key-in value.

Transcribed Image Text:i.
Complete the function definition for getInteger (.).
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
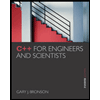
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
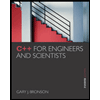
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr