// MichiganCities.cpp - This program prints a message for invalid cities in Michigan. // Input: Interactive // Output: Error message or nothing
// MichiganCities.cpp - This program prints a message for invalid cities in Michigan. // Input: Interactive // Output: Error message or nothing
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section: Chapter Questions
Problem 6PP: (Numerical) a. Define an array with a maximum of 20 integer values, and fill the array with numbers...
Related questions
Question
// MichiganCities.cpp - This program prints a message for invalid cities in Michigan.
// Input: Interactive
// Output: Error message or nothing
#include <iostream>
#include <string>
using namespace std;
int main()
{
// Declare variables
string inCity; // name of city to look up in array
const int NUM_CITIES = 10;
// Initialized array of cities
string citiesInMichigan[] = {"Acme", "Albion", "Detroit", "Watervliet", "Coloma", "Saginaw", "Richland", "Glenn", "Midland", "Brooklyn"};
bool foundIt = false; // Flag variable
int x; // Loop control variable
// Get user input
cout << "Enter name of city: ";
cin >> inCity;
// Write your loop here
// Write your test statement here to see if there is
// a match. Set the flag to true if city is found.
// Test to see if city was not found to determine if
// "Not a city in Michigan" message should be printed.
return 0;
} // End of main()
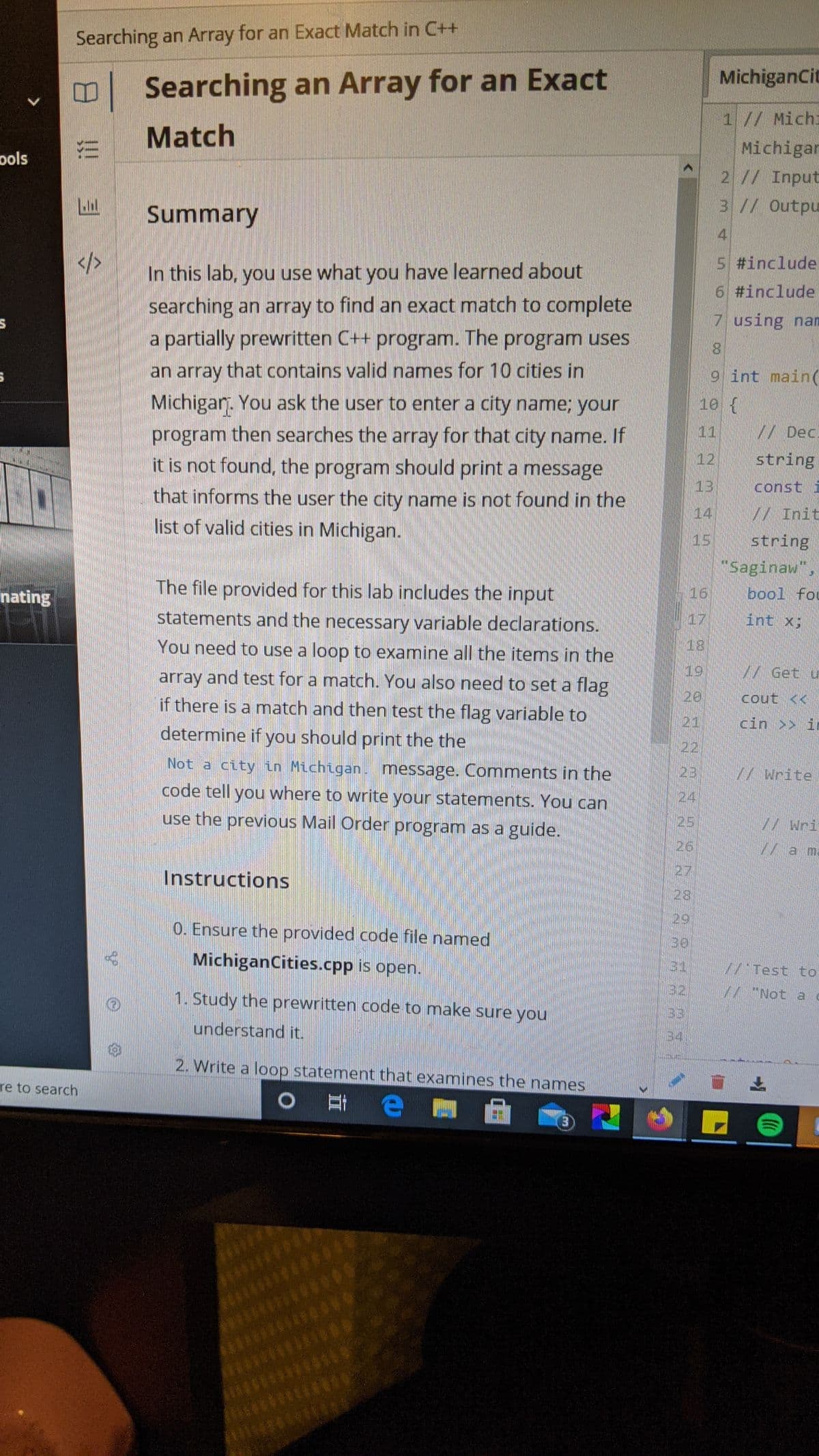
Transcribed Image Text:Searching an Array for an Exact Match in C++
MichiganCit
Searching an Array for an Exact
1// Mich:
Match
三
Michigan
2// Input
ools
B// Outpu
Summary
4
5 #include
In this lab, you use what you have learned about
6 #include
searching an array to find an exact match to complete
7 using nam
a partially prewritten C++ program. The program uses
an array that contains valid names for 10 cities in
9 int main(
Michigan. You ask the user to enter a city name; your
10 {
/ Dec.
program then searches the array for that city name. If
it is not found, the program should print a message
11
12
string
13
const i
that informs the user the city name is not found in the
/ Init
string
14
list of valid cities in Michigan.
15
"Saginaw",
nating
The file provided for this lab includes the input
16
bool for
statements and the necessary variable declarations.
17 int x;
You need to use a loop to examine all the items in the
18
19
/ Get u
array and test for a match. You also need to set a flag
if there is a match and then test the flag variable to
20
cout <<
21
cin >> in
determine if you should print the the
22
Not a city in Michigan. message. Comments in the
code tell you where to write your statements. You can
23
//Write
24
use the previous Mail Order program as a guide.
25
/ Wri
26
/am
27
Instructions
28
29
0. Ensure the provided code file named
30
MichiganCities.cpp is open.
31
Test to
1. Study the prewritten code to make sure you
32
/ "Not a c
understand it.
34.
2. Write a loop statement that examines the names
re to search
上
耳e■
! ヨ$
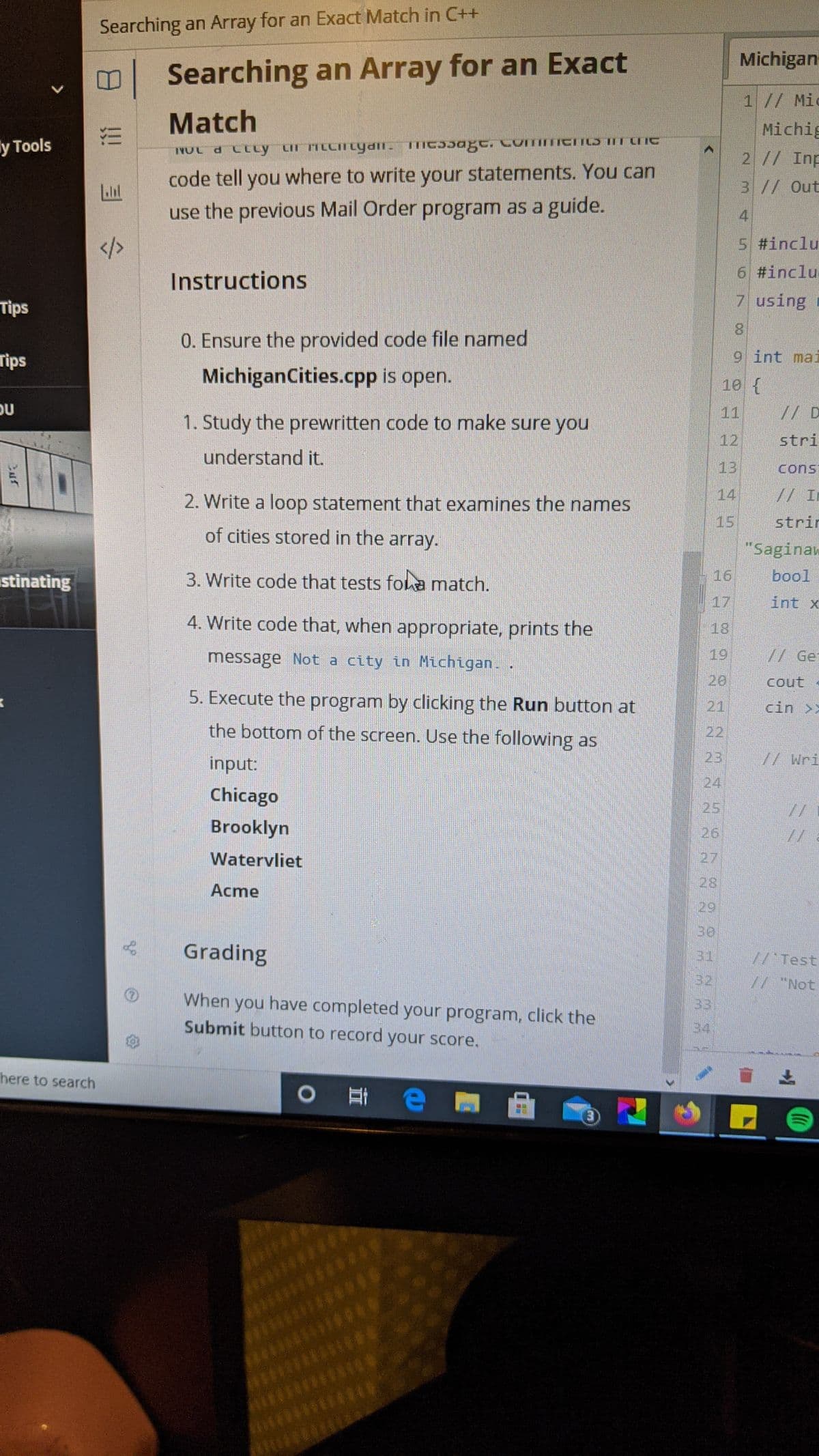
Transcribed Image Text:Searching an Array for an Exact Match in C++
Michigan
Searching an Array for an Exact
1// Mi
Match
Michig
У Тools
2// Inp
code tell you where to write your statements. You can
3//Out
use the previous Mail Order program as a guide.
4
5 #inclu
6 #inclu
Instructions
Tips
7 using i
8.
0. Ensure the provided code file named
Tips
9 int mai
MichiganCities.cpp is open.
10 {
11 // D
1. Study the prewritten code to make sure you
12 stri
understand it.
13
cons
2. Write a loop statement that examines the names
14 // In
15 strir
of cities stored in the array.
"Saginaw
stinating
3. Write code that tests foua match.
16
bool
|17
int x
4. Write code that, when appropriate, prints the
18
message Not a city in Michigan. .
19
// Ge
20
cout e
5. Execute the program by clicking the Run button at
21
cin >>
the bottom of the screen. Use the following as
22
input:
23
//Wri
24
Chicago
25
//
Brooklyn
26
//
Watervliet
27
28
Acme
29
30
Grading
31 //Test
32
// "Not
When you have completed your program, click the
Submit button to record your score.
34
here to search
LEGO
LEGO
ヨ
Cur
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
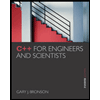
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
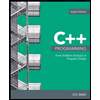
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
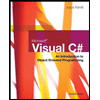
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
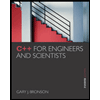
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
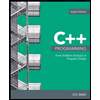
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
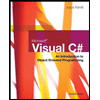
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,