efine a JavaScript comparator function named rossorder with 2 parameters. Both parameters will be objects. Each object will have 3 keys: "points", "goals", and "games". T alue paired with each key will be a Number. Each object represents an NHL player. Since rossOrder is a comparator function, it must return a Number as follows: • a negative value - indicating that the value of the first parameter comes before the value of the second parameter • a positive value - indicating that the value of the first parameter comes after the value of the second parameter • zero-indicating that the values of the two parameters appear at the same location in the order. o determine how the objects should be ordered, rossOrder should use the following rules (based upon the NHL's Ross Trophy): • when the objects have different values for "points", the object with fewer points comes first; • when the objects have the same number of points but unequal values for "goals" key, the object with fewer goals comes first; • when the objects have equal points and goals, the objects are equivalent. ample test cases: et mcdavid = {"points": 64, "goals": 22, "games": 43}; et draisaitl= {"points": 64, "goals": 23, "games": 43); et madeup= {"points": 64, "goals": 22, "games": 45}; ossOrder (mcdavid, madeup) would evaluate to 0 ossOrder (draisaitl, mcdavid) would evaluate to any Number greater than 0 ossOrder (madeup, draisaitl) would evaluate to any Number less than 0 2. efine a JavaScript custom sorting function named rossTrophy with 1 parameter. This parameter will be an array of objects. The objects will be as described in D1. Your function
efine a JavaScript comparator function named rossorder with 2 parameters. Both parameters will be objects. Each object will have 3 keys: "points", "goals", and "games". T alue paired with each key will be a Number. Each object represents an NHL player. Since rossOrder is a comparator function, it must return a Number as follows: • a negative value - indicating that the value of the first parameter comes before the value of the second parameter • a positive value - indicating that the value of the first parameter comes after the value of the second parameter • zero-indicating that the values of the two parameters appear at the same location in the order. o determine how the objects should be ordered, rossOrder should use the following rules (based upon the NHL's Ross Trophy): • when the objects have different values for "points", the object with fewer points comes first; • when the objects have the same number of points but unequal values for "goals" key, the object with fewer goals comes first; • when the objects have equal points and goals, the objects are equivalent. ample test cases: et mcdavid = {"points": 64, "goals": 22, "games": 43}; et draisaitl= {"points": 64, "goals": 23, "games": 43); et madeup= {"points": 64, "goals": 22, "games": 45}; ossOrder (mcdavid, madeup) would evaluate to 0 ossOrder (draisaitl, mcdavid) would evaluate to any Number greater than 0 ossOrder (madeup, draisaitl) would evaluate to any Number less than 0 2. efine a JavaScript custom sorting function named rossTrophy with 1 parameter. This parameter will be an array of objects. The objects will be as described in D1. Your function
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter11: Introduction To Classes
Section11.4: A Case Study: Constructing A Date Class
Problem 3E
Related questions
Question
need help writing these two functions. they ar in two seperate images if it helps you view them.
![D2.
Define a JavaScript custom sorting function named rossTrophy with 1 parameter. This parameter will be an array of objects. The objects will be as described in D1. Your function
should result in the array's objects being ordered from the object ranked lowest for the NHL's Ross Trophy to the object ranked highest for the NHL's Ross Trophy (from the smallest
to largest points; when objects have equal numbers of points, they are ordered from smallest to largest numbers of goals). Because JavaScript's built-in sort function reorders an
array's entries "in place", your rossTrophy function must return undefined.
Sample test cases:
let nh1 = [ {"points" : 64, "goals": 22, "games": 43},
{"points" : 64, "goals": 23, "games": 43} ];
rossTrophy (nh1) // the order of nh1's entries remains the same. (finds bug if sorting is not done using the built-in in-place function or if sorting is not done by rossorder)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F89b7b4a3-edc3-4856-b3b8-970ee403a9a8%2F69b2f09c-e511-498a-85cd-caf9dd1a4d9d%2Fr5j5m3_processed.png&w=3840&q=75)
Transcribed Image Text:D2.
Define a JavaScript custom sorting function named rossTrophy with 1 parameter. This parameter will be an array of objects. The objects will be as described in D1. Your function
should result in the array's objects being ordered from the object ranked lowest for the NHL's Ross Trophy to the object ranked highest for the NHL's Ross Trophy (from the smallest
to largest points; when objects have equal numbers of points, they are ordered from smallest to largest numbers of goals). Because JavaScript's built-in sort function reorders an
array's entries "in place", your rossTrophy function must return undefined.
Sample test cases:
let nh1 = [ {"points" : 64, "goals": 22, "games": 43},
{"points" : 64, "goals": 23, "games": 43} ];
rossTrophy (nh1) // the order of nh1's entries remains the same. (finds bug if sorting is not done using the built-in in-place function or if sorting is not done by rossorder)
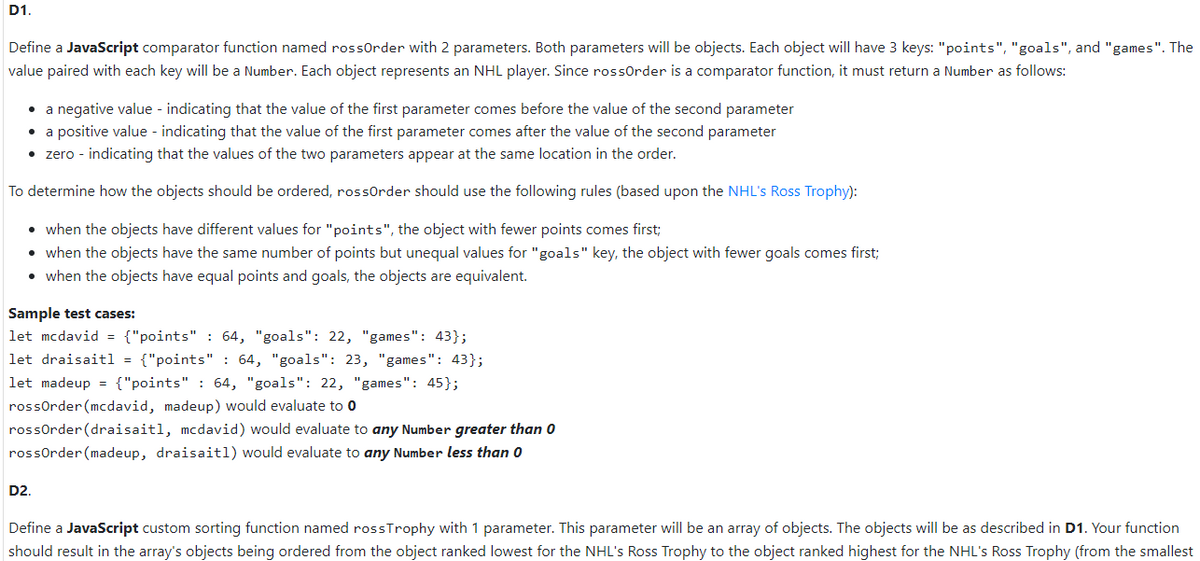
Transcribed Image Text:D1.
Define a JavaScript comparator function named rossOrder with 2 parameters. Both parameters will be objects. Each object will have 3 keys: "points", "goals", and "games". The
value paired with each key will be a Number. Each object represents an NHL player. Since rossOrder is a comparator function, it must return a Number as follows:
• a negative value - indicating that the value of the first parameter comes before the value of the second parameter
• a positive value - indicating that the value of the first parameter comes after the value of the second parameter
• zero - indicating that the values of the two parameters appear at the same location in the order.
To determine how the objects should be ordered, rossOrder should use the following rules (based upon the NHL's Ross Trophy):
• when the objects have different values for "points", the object with fewer points comes first;
• when the objects have the same number of points but unequal values for "goals" key, the object with fewer goals comes first;
• when the objects have equal points and goals, the objects are equivalent.
Sample test cases:
let mcdavid = {"points" : 64, "goals": 22, "games": 43};
let draisaitl = {"points": 64, "goals": 23, "games": 43};
let madeup = {"points" : 64, "goals": 22, "games": 45};
rossOrder (mcdavid, madeup) would evaluate to 0
rossOrder (draisaitl, mcdavid) would evaluate to any Number greater than 0
rossOrder (madeup, draisaitl) would evaluate to any Number less than 0
D2.
Define a JavaScript custom sorting function named rossTrophy with 1 parameter. This parameter will be an array of objects. The objects will be as described in D1. Your function
should result in the array's objects being ordered from the object ranked lowest for the NHL's Ross Trophy to the object ranked highest for the NHL's Ross Trophy (from the smallest
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
This cant be right.
first, first function needs to be called "rossOrder"
second, the second function requires only 1 parameter. this has 2.
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
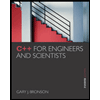
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
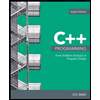
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
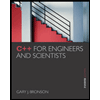
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
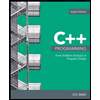
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning