Using classes and arrays, the team will develop a set of functions for an online shopping system. The system is represented by the following structure: 1- Class Item having the following private attributes: (ID, name, quantity, price) and the following public methods: - Constructors (default, parameterized, and copy) - Setters & Getters - Operator overloading for the ==, +=,-=, >> and << operators Note that the ID member variable is not entered or read from the user. It is automatically set by the class as a serial ID starting with the first item of ID 1 and incrementing with every new object. 2- Class Seller having the following private attributes: (name, email, items,maxItems), where items is a dynamic array of objects of type Item with the size maxItems. The class has the following public methods: - Constructor (parameterized) - Operator overloading for the insertion << operators - Add An Item. - This will take an Item object as a parameter: - If the item already exists in the seller's items you will increase the item’s quantity by the quantity of the parameter item using the (+=) in Item class, and the price of the parameter object will be ignored. Use the == operator for this where an item is equal to another if they have the same name. - Else you will add it to the seller’s items. - The member function should return a boolean that indicates the successful addition of item, which will succeed if there is a place in the array and fail otherwise. - Sell An Item. - This will take an item name and a quantity as parameters - If the quantity is <= item’s quantity you will decrease it from item Using the (-=) in Item class. - Else you will print him “There is only {quantity} left for this item”. - The member function should return a boolean which is true if the item was found, false otherwise. - Print Items. - This will print all the item information for the seller. - You will print each item using the (<<) operator. - Find an Item by ID - This returns an Item object (or a pointer to Item) with the specified ID if there is an item with such ID. - Destructor
Using classes and arrays, the team will develop a set of functions for an online
shopping system. The system is represented by the following structure:
1- Class Item having the following private attributes: (ID, name, quantity, price) and
the following public methods:
- Constructors (default, parameterized, and copy)
- Setters & Getters
- Operator overloading for the ==, +=,-=, >> and << operators
Note that the ID member variable is not entered or read from the user. It is
automatically set by the class as a serial ID starting with the first item of ID 1 and
incrementing with every new object.
2- Class Seller having the following private attributes: (name, email, items,maxItems),
where items is a dynamic array of objects of type Item with the size maxItems. The
class has the following public methods:
- Constructor (parameterized)
- Operator overloading for the insertion << operators
- Add An Item.
- This will take an Item object as a parameter:
- If the item already exists in the seller's items you will increase the item’s
quantity by the quantity of the parameter item using the (+=) in Item class,
and the price of the parameter object will be ignored. Use the == operator
for this where an item is equal to another if they have the same name.
- Else you will add it to the seller’s items.
- The member function should return a boolean that indicates the successful
addition of item, which will succeed if there is a place in the array and fail
otherwise.
- Sell An Item.
- This will take an item name and a quantity as parameters
- If the quantity is <= item’s quantity you will decrease it from item Using
the (-=) in Item class.
- Else you will print him “There is only {quantity} left for this item”.
- The member function should return a boolean which is true if the item was
found, false otherwise.
- Print Items.
- This will print all the item information for the seller.
- You will print each item using the (<<) operator.
- Find an Item by ID
- This returns an Item object (or a pointer to Item) with the specified ID if
there is an item with such ID.
- Destructor

Step by step
Solved in 2 steps

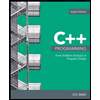
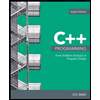