this code is in c++ and i having trouble making It run this is my first time working with classes and header files main.cpp #include #include #include #include"Pet.h" #include "Pet.cpp" using namespace std; int main() { Pet* myPets[2]; myPets[0] = new Pet("Fluffy", 2); myPets[1] = new Pet("Frisky", 4); for (int day = 1; day <= 9; day++) { cout << "======= Day " << day << endl; for (int k = 0; k < 2; k++) { careFor(myPets[k], day); } } cout << "=======" << endl; for (int k = 0; k < 2; k++) { if (myPets[k]->alive()) { cout << "Animal Control has come to rescue " << myPets[k]->name() << endl; } delete myPets[k]; } return 0; } pet.cpp #include #include "Pet.h" Pet::Pet(std::string nm, int initialHealth) { m_name = nm; m_health = initialHealth; } void Pet::eat(int amt) { if (amt < 0) return; m_health += amt; // Increase the pets health by the amount } void Pet::play() { m_health--; // Decrease pets health by1 for the energy consumed } std::string Pet::name() const { //Return the pets name. delete the following line // and replace it with the correct code. return m_name; // this implentation compiles, but is incorrect } int Pet::health() const { // TODO: Return the pet's current health level. Delete the // following line and replace it with the correct code. return m_health; } bool Pet::alive() const { if (m_health < 0) { return true; } else { return false; } // TODO: Return whether pet is alive. (A pet is alive if // its health is greater than zero.) Delete the following // line and replace it with the correct code. // This implementation compiles, but isn’t right } void reportStatus(const Pet* p) { std::cout << p->name() << " has health level " << p->health(); if (!p->alive()) { std::cout << ", so has died"; } std::cout << std::endl; } void careFor(Pet* p, int d) { if (!p->alive()) { std::cout << p->name() << " is still dead" << std::endl; return; } // Every third day, you forget to feed your pet if (d % 3 == 0) { std::cout << "You forgot to feed " << p->name() << std::endl; } else { p->eat(1); // Feed the pet one unit of food std::cout << "You fed " << p->name() << std::endl; } p->play(); reportStatus(p); } Pet.h #ifndef Pet_h #define Pet_h #include class Pet { public: Pet(std::string nm, int intialHealth); void eat(int amt); void play(); std::string name() const; int health() const; bool alive() const; private: std::string m_name; int m_health; }; #endif
this code is in c++ and i having trouble making It run this is my first time working with classes and header files
main.cpp
#include <iostream>
#include<iomanip>
#include <string>
#include"Pet.h"
#include "Pet.cpp"
using namespace std;
int main()
{
Pet* myPets[2];
myPets[0] = new Pet("Fluffy", 2);
myPets[1] = new Pet("Frisky", 4);
for (int day = 1; day <= 9; day++)
{
cout << "======= Day " << day << endl;
for (int k = 0; k < 2; k++) {
careFor(myPets[k], day);
}
}
cout << "=======" << endl;
for (int k = 0; k < 2; k++)
{
if (myPets[k]->alive()) {
cout << "Animal Control has come to rescue "
<< myPets[k]->name() << endl;
}
delete myPets[k];
}
return 0;
}
pet.cpp
#include<iostream>
#include "Pet.h"
Pet::Pet(std::string nm, int initialHealth)
{
m_name = nm;
m_health = initialHealth;
}
void Pet::eat(int amt)
{
if (amt < 0)
return;
m_health += amt;
// Increase the pets health by the amount
}
void Pet::play()
{
m_health--;
// Decrease pets health by1 for the energy consumed
}
std::string Pet::name() const
{
//Return the pets name. delete the following line
// and replace it with the correct code.
return m_name; // this implentation compiles, but is incorrect
}
int Pet::health() const
{
// TODO: Return the pet's current health level. Delete the
// following line and replace it with the correct code.
return m_health;
}
bool Pet::alive() const
{
if (m_health < 0)
{
return true;
}
else
{
return false;
}
// TODO: Return whether pet is alive. (A pet is alive if
// its health is greater than zero.) Delete the following
// line and replace it with the correct code.
// This implementation compiles, but isn’t right
}
void reportStatus(const Pet* p)
{
std::cout << p->name() << " has health level " << p->health();
if (!p->alive()) {
std::cout << ", so has died";
}
std::cout << std::endl;
}
void careFor(Pet* p, int d)
{
if (!p->alive())
{
std::cout << p->name() << " is still dead" << std::endl;
return;
}
// Every third day, you forget to feed your pet
if (d % 3 == 0) {
std::cout << "You forgot to feed " << p->name() << std::endl;
}
else
{
p->eat(1); // Feed the pet one unit of food
std::cout << "You fed " << p->name() << std::endl;
}
p->play();
reportStatus(p);
}
Pet.h
#ifndef Pet_h
#define Pet_h
#include<string>
class Pet
{
public:
Pet(std::string nm, int intialHealth);
void eat(int amt);
void play();
std::string name() const;
int health() const;
bool alive() const;
private:
std::string m_name;
int m_health;
};
#endif

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

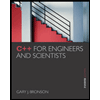
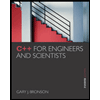