This is my code in c++. When the chosen class is full I want the code to automatically assign it to the next available class (Economy). Also when the economy class is full I want the code to automatically assign it to the next available First Class seat. #include using namespace std; void ShowInfo(); void printInfo(char input[9][4]); void DesiredRow(int row[2], char seat); int main () { int ROW = 9, SEATS = 4; int row[9]; char airchar[9][4]; char seat; string answer; string ticket; string name; string newanswer; for (int i = 0; i < ROW; i++) { for (int j = 0; j < SEATS; j++) { airchar[i][j] = '*'; } } cout << "Please enter your full name: " << endl; getline(cin, name); while ("Yes") { cout << " Do you wish to book a seat: " << endl; cout << " Yes or No: " << endl; cin >> answer; if (answer == "Yes") { ShowInfo(); cin >> ticket; printInfo(airchar); cout << "Desired Row? " << endl; cin >> row[0]; cout << "Desired Seat (A, B, C, D) : "<> seat; DesiredRow(row, seat); if (ticket == "FS") { if (row[0] + 1 || row[0] + 1 == 2) { if (airchar[row[0]][row[1]] == '*') { airchar[row[0]][row[1]] = 'X'; printInfo(airchar); } } else if(airchar[row[0]][row[1]] == 'X') { cout<<"Message: Seat "<> newanswer; if (newanswer == "Yes") { } } else { cout << "Wrong class " << endl; } } else if(ticket == "EC") { if(row[0]+1 == 3 || row[0]+1 == 4 || row[0]+1 == 5 || row[0]+1 == 6|| row[0]+1 == 7|| row[0]+1 == 8|| row[0]+1 == 9) { if(airchar[row[0]][row[1]] == '*') { airchar[row[0]][row[1]] = 'X'; printInfo(airchar); } else if(airchar[row[0]][row[1]] == 'X') { cout<<"Message: Seat "<
This is my code in c++. When the chosen class is full I want the code to automatically assign it to the next available class (Economy). Also when the economy class is full I want the code to automatically assign it to the next available First Class seat.
#include <iostream>
using namespace std;
void ShowInfo();
void printInfo(char input[9][4]);
void DesiredRow(int row[2], char seat);
int main ()
{
int ROW = 9, SEATS = 4;
int row[9];
char airchar[9][4];
char seat;
string answer;
string ticket;
string name;
string newanswer;
for (int i = 0; i < ROW; i++)
{
for (int j = 0; j < SEATS; j++)
{
airchar[i][j] = '*';
}
}
cout << "Please enter your full name: " << endl;
getline(cin, name);
while ("Yes")
{
cout << " Do you wish to book a seat: " << endl;
cout << " Yes or No: " << endl;
cin >> answer;
if (answer == "Yes")
{
ShowInfo();
cin >> ticket;
printInfo(airchar);
cout << "Desired Row? " << endl;
cin >> row[0];
cout << "Desired Seat (A, B, C, D) : "<<endl;
cin >> seat;
DesiredRow(row, seat);
if (ticket == "FS")
{
if (row[0] + 1 || row[0] + 1 == 2)
{
if (airchar[row[0]][row[1]] == '*')
{
airchar[row[0]][row[1]] = 'X';
printInfo(airchar);
}
}
else if(airchar[row[0]][row[1]] == 'X')
{
cout<<"Message: Seat "<<row[0] + 1<<seat<<" is already occupied"<<endl;
cout <<"Book a different seat? " << endl;
cout << "Yes or No" << endl;
cin >> newanswer;
if (newanswer == "Yes")
{
}
}
else
{
cout << "Wrong class " << endl;
}
}
else if(ticket == "EC")
{
if(row[0]+1 == 3 || row[0]+1 == 4 || row[0]+1 == 5
|| row[0]+1 == 6|| row[0]+1 == 7|| row[0]+1 == 8|| row[0]+1 == 9)
{
if(airchar[row[0]][row[1]] == '*')
{
airchar[row[0]][row[1]] = 'X';
printInfo(airchar);
}
else if(airchar[row[0]][row[1]] == 'X')
{
cout<<"Message: Seat "<<row[0] + 1<<seat<<" is already occupied"<<endl;
}
}
else
{
cout<<"Wrong Class"<<endl;
}
}//end of else if loop
} // end of nst loop
else if (answer == "No")
{
cout << "Have a good day" << endl;
cout << name << " your current seating plan is" << endl;
printInfo(airchar);
return 0;
}
row[0] = 0;
row[1] = 0;
}// end of while (yes) loop
}
void ShowInfo()
{
cout<<"Rows 1 and 2 are first class (FC)"<<endl;
cout<<"Rows 3 through 7 are business class (BC)"<<endl;
cout<<"Rows 8 through 13 are economy class (EC)"<<endl;
cout<<endl;
cout<<"* - Available"<<endl;
cout<<"X - Occupied"<<endl;
cout<<endl;
cout << "Enter desired ticket type " << endl;
cout << "FS - first class \n EC - economy" << endl;
}
void printInfo(char input[9][4])
{
cout << "\t\t"
<< "A\tB\tC\tD" << endl;
for (int i = 0; i < 9; i++)
{
cout << "Row " << i+1 << "\t";
for (int j = 0; j < 4; j++)
{
cout << input[i][j] << "\t";
}
cout << endl;
}
}
void DesiredRow(int row[2], char seat)
{
switch (seat)
{
case 'A':
row[0] = row[0] - 1;
row[1] = 1;
row[1] = row[1] - 1;
break;
case 'B':
row[0] = row[0] - 1;
row[1] = 2;
row[1] = row[1] - 1;
break;
case 'C':
row[0] = row[0] - 1;
row[1] = 3;
row[1] = row[1] - 1;
break;
case 'D':
row[0] = row[0] - 1;
row[1] = 4;
row[1] = row[1] - 1;
break;
}
}

Step by step
Solved in 3 steps with 2 images

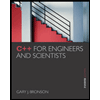
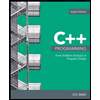
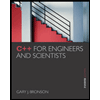
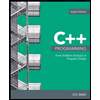