this code is in java. Explore the given classes. Implement a getter method on the WasteRobot class with the following method signature public ArrayList getWasteBin(String wasteType) The method should return the proper waste bin based on the passed wasteType. Compare the given wasteType to each of the public static final constants in the Waste class to help determine which bin to return. Given Classes : WasteRobotTester.java import java.util.*; public class WasteRobotTester { public static void main(String[] args) { WasteRobot wallie = new WasteRobot(); // Your test code. } } WasteRobot.java import java.util.*; public class WasteRobot { private ArrayList trashBin; private ArrayList compostBin; private ArrayList recyclingBin; public WasteRobot() { trashBin = new ArrayList(); compostBin = new ArrayList(); recyclingBin = new ArrayList(); trashBin.add(new Trash("Plastic Bag")); compostBin.add(new Compost("Banana Peel")); recyclingBin.add(new Recycling("Soda Can")); } // Implement this! // getWasteBin(String wasteType) } Waste.java public abstract class Waste { public static final String TRASH_TYPE = "trash"; public static final String COMPOST_TYPE = "compost"; public static final String RECYCLING_TYPE = "recycling"; private String wasteType; private String wasteName; public Waste(String wasteType, String wasteName) { this.wasteType = wasteType; this.wasteName = wasteName; } public String getWasteType() { return wasteType; } public String toString() { return wasteType + ": " + wasteName; } } Trash.java public class Trash extends Waste { public Trash(String wasteName) { super(Waste.TRASH_TYPE, wasteName); } } Recycling.java public class Recycling extends Waste { public Recycling(String wasteName) { super(Waste.RECYCLING_TYPE, wasteName); } } Compost.java public class Compost extends Waste { public Compost(String wasteName) { super(Waste.COMPOST_TYPE, wasteName); } }
this code is in java.
Explore the given classes. Implement a getter method on the WasteRobot class with the following method signature
public ArrayList<Waste> getWasteBin(String wasteType)
The method should return the proper waste bin based on the passed wasteType. Compare the given wasteType to each of the public static final constants in the Waste class to help determine which bin to return.
Given Classes :
WasteRobotTester.java
import java.util.*;
public class WasteRobotTester
{
public static void main(String[] args)
{
WasteRobot wallie = new WasteRobot();
// Your test code.
}
}
WasteRobot.java
import java.util.*;
public class WasteRobot
{
private ArrayList<Waste> trashBin;
private ArrayList<Waste> compostBin;
private ArrayList<Waste> recyclingBin;
public WasteRobot()
{
trashBin = new ArrayList<Waste>();
compostBin = new ArrayList<Waste>();
recyclingBin = new ArrayList<Waste>();
trashBin.add(new Trash("Plastic Bag"));
compostBin.add(new Compost("Banana Peel"));
recyclingBin.add(new Recycling("Soda Can"));
}
// Implement this!
// getWasteBin(String wasteType)
}
Waste.java
public abstract class Waste
{
public static final String TRASH_TYPE = "trash";
public static final String COMPOST_TYPE = "compost";
public static final String RECYCLING_TYPE = "recycling";
private String wasteType;
private String wasteName;
public Waste(String wasteType, String wasteName) {
this.wasteType = wasteType;
this.wasteName = wasteName;
}
public String getWasteType()
{
return wasteType;
}
public String toString()
{
return wasteType + ": " + wasteName;
}
}
Trash.java
public class Trash extends Waste
{
public Trash(String wasteName)
{
super(Waste.TRASH_TYPE, wasteName);
}
}
Recycling.java
public class Recycling extends Waste
{
public Recycling(String wasteName)
{
super(Waste.RECYCLING_TYPE, wasteName);
}
}
Compost.java
public class Compost extends Waste
{
public Compost(String wasteName)
{
super(Waste.COMPOST_TYPE, wasteName);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

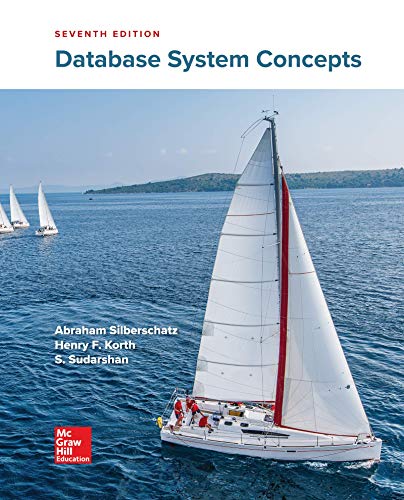
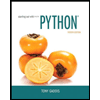
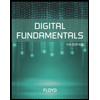
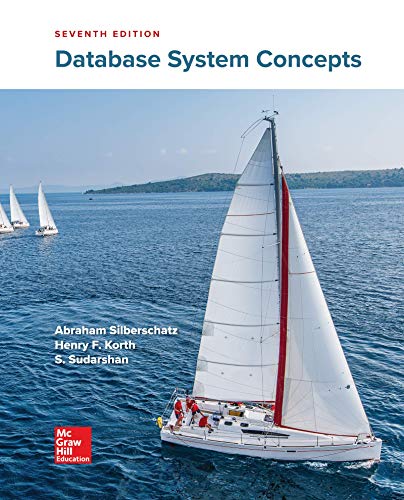
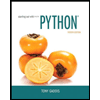
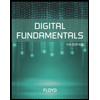
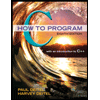
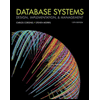
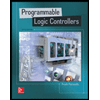