Take the Linked List from Problem 2 and let's create a linked list of songs. To achieve this, create a Song class with the following attributes: 1. Song Title 2. Song Author 3. Song Genre 4. Song Time (i.e., how long is the song in seconds) Songs should be sorted by Title. Write overloaded methods for the relational operations that compares the titles of a song. Create an Ordered Doubly Linked List and store random songs in the Linked List.
Please provide C++ program code to the following question. Please list and provide all steps with code solution and results of code when executed. .
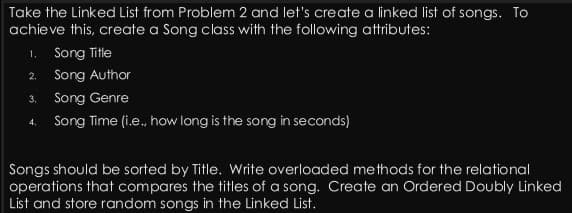

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 8 images

This code is not working. I'm getting errors.Can you check code again.
#include <cctype>
#include <cstring>
#include <iostream>
using namespace std;
class Song {
private:
char* title;
char* author;
char* genre;
float time;
public:
Song();
Song(char* t, char* a, char* g, float ti);
~Song();
void Set(char* t, char* a, char* g, float ti);
void Display();
bool operator<(Song& a);
bool operator<=(Song& a);
bool operator>(Song& a);
bool operator>=(Song& a);
bool operator==(Song& a);
bool operator!=(Song& a);
};
Song::Song() {
title = NULL;
author = NULL;
genre = NULL;
time = 0;
}
Song::Song(char* t, char* a, char* g, float ti) {
title = new char[strlen(t) + 1];
strcpy(title, t);
author = new char[strlen(a) + 1];
strcpy(author, a);
genre = new char[strlen(g) + 1];
strcpy(genre, g);
time = ti;
}
Song::~Song() {
delete[] title;
delete[] author;
delete[] genre;
}
void Song::Set(char* t, char* a, char* g, float ti) {
title = new char[strlen(t) + 1];
strcpy(title, t);
author = new char[strlen(a) + 1];
strcpy(author, a);
genre = new char[strlen(g) + 1];
strcpy(genre, g);
time = ti;
}
void Song::Display() {
cout << "Title: " << title << endl;
cout << "Author: " << author << endl;
cout << "Genre: " << genre << endl;
cout << "Time: " << time << endl;
}
bool Song::operator<(Song& a) {
if (strcmp(title, a.title) < 0)
return true;
return false;
}
bool Song::operator<=(Song& a) {
if (strcmp(title, a.title) <= 0)
return true;
return false;
}
bool Song::operator>(Song& a) {
if (strcmp(title, a.title) > 0)
return true;
return false;
}
bool Song::operator>=(Song& a) {
if (strcmp(title, a.title) >= 0)
return true;
return false;
}
bool Song::operator==(Song& a) {
if (strcmp(title, a.title) == 0)
return true;
return false;
}
bool Song::operator!=(Song& a) {
if (strcmp(title, a.title) != 0)
return true;
return false;
}
int main() {
Song song1("Despacito", "Luis Fonsi", "Pop", 4.42);
Song song2("Shape of You", "Ed Sheeran", "Pop", 3.53);
Song song3("I Don't Wanna Live Forever", "Zayn Malik", "Pop", 4.10);
if (song1 < song2)
cout << "song1 comes before song2" << endl;
else
cout << "song1 does not come before song2" << endl;
if (song2 <= song3)
cout << "song2 comes before or is equal to song3" << endl;
else
cout << "song2 does not come before or is equal to song3" << endl;
if (song1 > song2)
cout << "song1 comes after song2" << endl;
else
cout << "song1 does not come after song2" << endl;
if (song1 >= song2)
cout << "song1 comes after or is equal to song2" << endl;
else
cout << "song1 does not come after or is equal to song2" << endl;
if (song1 == song2)
cout << "song1 is equal to song2" << endl;
else
cout << "song1 is not equal to song2" << endl;
if (song1 != song2)
cout << "song1 is not equal to song2" << endl;
else
cout << "song1 is equal to song2" << endl;
return 0;
}
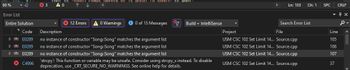
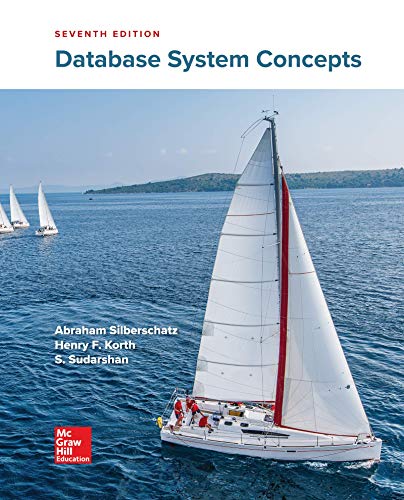
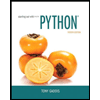
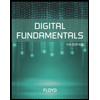
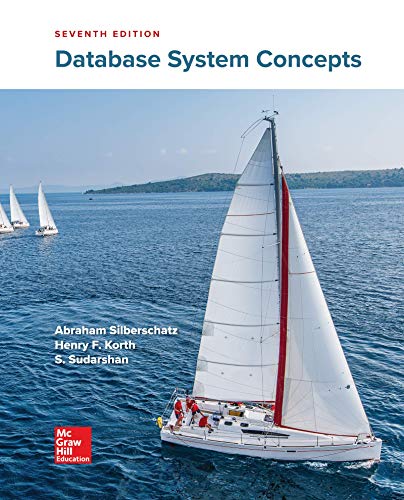
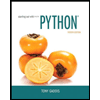
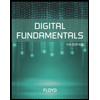
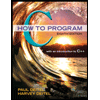
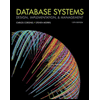
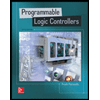