Add a method to the Parking Office to return the collection of permit ids for a specific customer (getPermitIds(Customer)) I have already coded the permit id class, but I attached the class diagram for you to see. Current Java Code: package parkingsystem4; import java.util.LinkedList; import java.util.List; public class ParkingOffice { String name; String address; List cars = new LinkedList(); List customers = new LinkedList(); List lots = new LinkedList(); List charges = new LinkedList(); // Default constructor ParkingOffice(){ } // Parameterized constructor ParkingOffice(String name, String address, List parkedCars, List lots, List charges){ this.name = name; this.address = address; this.cars = parkedCars; this.lots = lots; this.charges = charges; } public void registerCustomer(Customer registeringCustomer){ customers.add(registeringCustomer); } public void registerCar(Car registeringCar){ cars.add(registeringCar); } public void addParkingLot(ParkingLot parkingLot){ lots.add(parkingLot); } public void addCharge(ParkingCharge parkingCharge) { charges.add(parkingCharge); } public void printRegisteredCars() { System.out.println(""); System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++"); System.out.println("The Parking Office has the following cars registered"); System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++"); for (int i = 0; i < cars.size(); i++) { System.out.println(cars.get(i)); } System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++"); System.out.println(""); } public void printParkingLots() { System.out.println(""); System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++"); System.out.println("The Parking Office has the following parking lots"); System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++"); for (int i = 0; i < lots.size(); i++) { System.out.println(lots.get(i)); } System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++"); System.out.println(""); } public void printCustomers() { System.out.println(""); System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++"); System.out.println("The Parking Office has the following registered customers"); System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++"); for (int i = 0; i < customers.size(); i++) { System.out.println(customers.get(i)); } System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++"); System.out.println(""); } public void printCharges() { System.out.println(""); System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++"); System.out.println("The Parking Office has the following charges"); System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++"); }
- Add a method to the Parking Office to return the collection of permit ids for a specific customer (getPermitIds(Customer)) I have already coded the permit id class, but I attached the class diagram for you to see.
Current Java Code:
package parkingsystem4;
import java.util.LinkedList;
import java.util.List;public class ParkingOffice {
String name;
String address;
List<Car> cars = new LinkedList<Car>();
List<Customer> customers = new LinkedList<Customer>();
List<ParkingLot> lots = new LinkedList<ParkingLot>();
List<ParkingCharge> charges = new LinkedList<ParkingCharge>();
// Default constructor
ParkingOffice(){
}// Parameterized constructor
ParkingOffice(String name, String address, List<Car> parkedCars, List<ParkingLot> lots, List<ParkingCharge> charges){
this.name = name;
this.address = address;
this.cars = parkedCars;
this.lots = lots;
this.charges = charges;
}
public void registerCustomer(Customer registeringCustomer){
customers.add(registeringCustomer);
}
public void registerCar(Car registeringCar){
cars.add(registeringCar);
}
public void addParkingLot(ParkingLot parkingLot){
lots.add(parkingLot);
}
public void addCharge(ParkingCharge parkingCharge) {
charges.add(parkingCharge);
}
public void printRegisteredCars() {
System.out.println("");
System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++");
System.out.println("The Parking Office has the following cars registered");
System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++");
for (int i = 0; i < cars.size(); i++) {
System.out.println(cars.get(i));
}
System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++");
System.out.println("");
}
public void printParkingLots() {
System.out.println("");
System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++");
System.out.println("The Parking Office has the following parking lots");
System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++");
for (int i = 0; i < lots.size(); i++) {
System.out.println(lots.get(i));
}
System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++");
System.out.println("");
}
public void printCustomers() {
System.out.println("");
System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++");
System.out.println("The Parking Office has the following registered customers");
System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++");
for (int i = 0; i < customers.size(); i++) {
System.out.println(customers.get(i));
}
System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++");
System.out.println("");
}
public void printCharges() {
System.out.println("");
System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++");
System.out.println("The Parking Office has the following charges");
System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++++++++");}
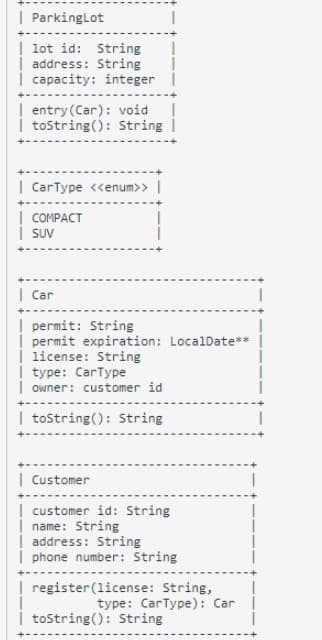

Step by step
Solved in 4 steps with 1 images

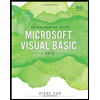
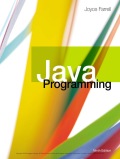
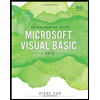
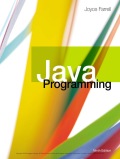