Event Listeners Go to the co_cart.js file in your editor. Directly below the initial comment section, add an event listener for the window load event that does the following when the page is loaded: Runs the calcCart() function. Add an event handler to the modelQty field in the cart form that runs the calcCart() function when the field value is changed. A for loop that loops through every option in the group of shipping option buttons, adding an event handler to run the calcCart() function when each option button is clicked. JavaScript Functions Create the calcCart() function to calculate the cost of the customer’s order using field values in the cart form. Within the calcCart() function do the following: Create a variable named orderCost that is equal to the cost of the espresso machine stored in the modelCost field multiplied by the quantity of machines ordered as stored in the modelQty field. Display the value of the orderCost variable in the orderCost field, formatted as U.S. currency. (Hint: Use the formatUSCurrency() function.) Create a variable named shipCost equal to the value of the selected shipping option from the group of shipping option buttons multiplied by the quantity of machines ordered. Display the value of the shipCost variable in the shippingCost field, formatted with a thousands separator and to two decimals places. (Hint: Use the formatNumber() function.) In the subTotal field, display the sum of orderCost and shipCost formatted with a thousands separator and to two decimal places. Create a variable named salesTax equal to 0.05 times the sum of the orderCost and shipCost variables. Display the value of the salesTax variable in the salesTax field, formatted with a thousands separator and to two decimal places. In the cartTotal field, display the sum of the orderCost, shipCost, and salesTax variables. Format the value as U.S. currency. Store the label text of the shipping option selected by the user from the shipping field in the hidden shippingType field. java script------------------------------------------------------------- "use strict"; /* New Perspectives on HTML5, CSS3, and JavaScript 6th Edition Tutorial 13 Review Assigment Shopping Cart Form Script Author: Date: Filename: co_cart.js Function List ============= calcCart() Calculates the cost of the customer order formatNumber(val, decimals) Format a numeric value, val, using the local numeric format to the number of decimal places specified by decimals formatUSACurrency(val) Formats val as U.S.A. currency */ window.addEventListener("load",function () { calcCart(); var cart = document.forms.cart; cart.elements.modelQty.onchange = calcCart; var shippingOptions = document.querySelectorAll('input[name="shipping"]'); for (var i = 0;i <= shippingOptions.length; i++) { shippingOptions[i].onclick = calcCart; } }); function calcCart() { var cart = document.forms.cart; var machineCost = cart.elements.modelCost.value; var qIndex = cart.elements.modelQty.selectedIndex; var qty = cart.elements.modelQty[qIndex].value; var orderCost = machineCost * qty; cart.elements.orderCost.value = formatUSCurrency(orderCost); var shipCost = document.querySelector('input[name="shipping"]:checked').value*qty; cart.elements.shippingCost.value = formatNumber(shipCost); cart.elements.subTotal.value = formatNumber(orderCost+shipCost, 2); var salesTax = 0.05*(orderCost+shipCost); cart.elements.salesTax.value = formatNumber(salesTax,2); cart.elements.cartTotal.value = formatUSCurrency(orderCost+shipCost+salesTax); } function formatNumber(val, decimals) { return val.toLocaleString(undefined, { minimumFractionDigits: decimals, maximumFractionDigits: decimals }); } function formatUSCurrency(val) { return val.toLocaleString('en-US', { style: "currency", currency: "USD" });
Event Listeners Go to the co_cart.js file in your editor. Directly below the initial comment section, add an event listener for the window load event that does the following when the page is loaded: Runs the calcCart() function. Add an event handler to the modelQty field in the cart form that runs the calcCart() function when the field value is changed. A for loop that loops through every option in the group of shipping option buttons, adding an event handler to run the calcCart() function when each option button is clicked. JavaScript Functions Create the calcCart() function to calculate the cost of the customer’s order using field values in the cart form. Within the calcCart() function do the following: Create a variable named orderCost that is equal to the cost of the espresso machine stored in the modelCost field multiplied by the quantity of machines ordered as stored in the modelQty field. Display the value of the orderCost variable in the orderCost field, formatted as U.S. currency. (Hint: Use the formatUSCurrency() function.) Create a variable named shipCost equal to the value of the selected shipping option from the group of shipping option buttons multiplied by the quantity of machines ordered. Display the value of the shipCost variable in the shippingCost field, formatted with a thousands separator and to two decimals places. (Hint: Use the formatNumber() function.) In the subTotal field, display the sum of orderCost and shipCost formatted with a thousands separator and to two decimal places. Create a variable named salesTax equal to 0.05 times the sum of the orderCost and shipCost variables. Display the value of the salesTax variable in the salesTax field, formatted with a thousands separator and to two decimal places. In the cartTotal field, display the sum of the orderCost, shipCost, and salesTax variables. Format the value as U.S. currency. Store the label text of the shipping option selected by the user from the shipping field in the hidden shippingType field. java script------------------------------------------------------------- "use strict"; /* New Perspectives on HTML5, CSS3, and JavaScript 6th Edition Tutorial 13 Review Assigment Shopping Cart Form Script Author: Date: Filename: co_cart.js Function List ============= calcCart() Calculates the cost of the customer order formatNumber(val, decimals) Format a numeric value, val, using the local numeric format to the number of decimal places specified by decimals formatUSACurrency(val) Formats val as U.S.A. currency */ window.addEventListener("load",function () { calcCart(); var cart = document.forms.cart; cart.elements.modelQty.onchange = calcCart; var shippingOptions = document.querySelectorAll('input[name="shipping"]'); for (var i = 0;i <= shippingOptions.length; i++) { shippingOptions[i].onclick = calcCart; } }); function calcCart() { var cart = document.forms.cart; var machineCost = cart.elements.modelCost.value; var qIndex = cart.elements.modelQty.selectedIndex; var qty = cart.elements.modelQty[qIndex].value; var orderCost = machineCost * qty; cart.elements.orderCost.value = formatUSCurrency(orderCost); var shipCost = document.querySelector('input[name="shipping"]:checked').value*qty; cart.elements.shippingCost.value = formatNumber(shipCost); cart.elements.subTotal.value = formatNumber(orderCost+shipCost, 2); var salesTax = 0.05*(orderCost+shipCost); cart.elements.salesTax.value = formatNumber(salesTax,2); cart.elements.cartTotal.value = formatUSCurrency(orderCost+shipCost+salesTax); } function formatNumber(val, decimals) { return val.toLocaleString(undefined, { minimumFractionDigits: decimals, maximumFractionDigits: decimals }); } function formatUSCurrency(val) { return val.toLocaleString('en-US', { style: "currency", currency: "USD" });
New Perspectives on HTML5, CSS3, and JavaScript
6th Edition
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Patrick M. Carey
Chapter7: Designing A Web Form: Creating A Survey Form
Section: Chapter Questions
Problem 4CP3
Related questions
Question
Event Listeners
Go to the co_cart.js file in your editor. Directly below the initial comment section, add an event listener for the window load event that does the following when the page is loaded:
- Runs the calcCart() function.
- Add an event handler to the modelQty field in the cart form that runs the calcCart() function when the field value is changed.
- A for loop that loops through every option in the group of shipping option buttons, adding an event handler to run the calcCart() function when each option button is clicked.
JavaScript Functions
Create the calcCart() function to calculate the cost of the customer’s order using field values in the cart form. Within the calcCart() function do the following:
- Create a variable named orderCost that is equal to the cost of the espresso machine stored in the modelCost field multiplied by the quantity of machines ordered as stored in the modelQty field. Display the value of the orderCost variable in the orderCost field, formatted as U.S. currency. (Hint: Use the formatUSCurrency() function.)
- Create a variable named shipCost equal to the value of the selected shipping option from the group of shipping option buttons multiplied by the quantity of machines ordered. Display the value of the shipCost variable in the shippingCost field, formatted with a thousands separator and to two decimals places. (Hint: Use the formatNumber() function.)
- In the subTotal field, display the sum of orderCost and shipCost formatted with a thousands separator and to two decimal places.
- Create a variable named salesTax equal to 0.05 times the sum of the orderCost and shipCost variables. Display the value of the salesTax variable in the salesTax field, formatted with a thousands separator and to two decimal places.
- In the cartTotal field, display the sum of the orderCost, shipCost, and salesTax variables. Format the value as U.S. currency.
- Store the label text of the shipping option selected by the user from the shipping field in the hidden shippingType field.
java script-------------------------------------------------------------
"use strict";
/*
New Perspectives on HTML5, CSS3, and JavaScript 6th Edition
Tutorial 13
Review Assigment
Shopping Cart Form Script
Author:
Date:
Filename: co_cart.js
Function List
=============
calcCart()
Calculates the cost of the customer order
formatNumber(val, decimals)
Format a numeric value, val, using the local
numeric format to the number of decimal
places specified by decimals
formatUSACurrency(val)
Formats val as U.S.A. currency
*/
window.addEventListener("load",function () {
calcCart();
var cart = document.forms.cart;
cart.elements.modelQty.onchange = calcCart;
var shippingOptions = document.querySelectorAll('input[name="shipping"]');
for (var i = 0;i <= shippingOptions.length; i++) {
shippingOptions[i].onclick = calcCart;
}
});
function calcCart() {
var cart = document.forms.cart;
var machineCost = cart.elements.modelCost.value;
var qIndex = cart.elements.modelQty.selectedIndex;
var qty = cart.elements.modelQty[qIndex].value;
var orderCost = machineCost * qty;
cart.elements.orderCost.value = formatUSCurrency(orderCost);
var shipCost = document.querySelector('input[name="shipping"]:checked').value*qty;
cart.elements.shippingCost.value = formatNumber(shipCost);
cart.elements.subTotal.value = formatNumber(orderCost+shipCost, 2);
var salesTax = 0.05*(orderCost+shipCost);
cart.elements.salesTax.value = formatNumber(salesTax,2);
cart.elements.cartTotal.value = formatUSCurrency(orderCost+shipCost+salesTax);
}
function formatNumber(val, decimals) {
return val.toLocaleString(undefined, {
minimumFractionDigits: decimals,
maximumFractionDigits: decimals
});
}
function formatUSCurrency(val) {
return val.toLocaleString('en-US', { style: "currency", currency: "USD" });
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
This doesn't show the last step; store shipping label text in shippingType field
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
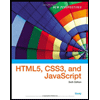
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:
9780357392676
Author:
FREUND, Steven
Publisher:
CENGAGE L
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage
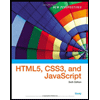
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:
9780357392676
Author:
FREUND, Steven
Publisher:
CENGAGE L
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage