This has to be in C++ Write a program to help determine the cost of an armoire (a fancy kind of cabinet). For this program, the basic armoire cost is $450, but options available to the customer can cause the price to increase. Your program must ask the user the following questions INSIDE a function called “get_measurements” (pass the arguments into the function by REFERENCE): The length of the Armoire (in inches) The width of the Armoire (the "depth" of the armoire in inches) The height of the Armoire (in inches) After these values have been set by the function, the three arguments are then passed by value to another function called calc_Large_Volume to calculate the cubic volume of the Armoire and return a charge amount based on the volume. If the calculated cubic volume (length X width X height) is greater than 25,000 cubic inches, an additional charge of $100 will be set, and returned by the function, otherwise the additional charge will be set to $0 and returned. The program will then ask the user if they want a premium armoire (made of Oak). The premium charge for the armoire is an additional $300. Another option (lining) will be asked for, but only if the user indicates they want the premium Armoire. If the customer wants a premium Armoire, then a function called calc_Charge will be called, passing the base price of the Armoire, the charge for the cubic volume (either $0 or $100 depending on the calculated charge) and the premium charge. All three values will be passed by value. Inside this function, ask the user if they want the custom lining (Teak wood). If they do, an additional $100 charge will be added to this armoire’s total cost. The function will calculate the charge for the Armoire and return it to the main program for display. If the customer does NOT want the premium version of the Armoire, a function called calc_Charge (same name as in option 1) will be called, passing the base price of the Armoire and the charge for cubic volume. The function will calculate the charge for the Armoire and return it to the main program for display. You will need to determine if any other arguments are passed into these functions. If there are additional arguments, you must ensure that the argument lists for each of the two functions called calc_Charge are sufficiently different enough for them to still be considered overloaded. The main program will then display the type of Armoire (Premium or basic), along with all options chosen (including whether the Teak Lining was chosen as an option) and the costs for each option. If the large cubic volume charge is incurred, that needs to be listed as well. input validation: Do not accept any values for the dimensions less than 18 inches, nor more than 60 inches for the height.
This has to be in C++
-
Write a
program to help determine the cost of an armoire (a fancy kind of cabinet). For this program, the basic armoire cost is $450, but options available to the customer can cause the price to increase. Your program must ask the user the following questions INSIDE a function called “get_measurements” (pass the arguments into the function by REFERENCE):- The length of the Armoire (in inches)
- The width of the Armoire (the "depth" of the armoire in inches)
- The height of the Armoire (in inches)
After these values have been set by the function, the three arguments are then passed by value to another function called calc_Large_Volume to calculate the cubic volume of the Armoire and return a charge amount based on the volume. If the calculated cubic volume (length X width X height) is greater than 25,000 cubic inches, an additional charge of $100 will be set, and returned by the function, otherwise the additional charge will be set to $0 and returned.
The program will then ask the user if they want a premium armoire (made of Oak). The premium charge for the armoire is an additional $300. Another option (lining) will be asked for, but only if the user indicates they want the premium Armoire.
- If the customer wants a premium Armoire, then a function called calc_Charge will be called, passing the base price of the Armoire, the charge for the cubic volume (either $0 or $100 depending on the calculated charge) and the premium charge. All three values will be passed by value. Inside this function, ask the user if they want the custom lining (Teak wood). If they do, an additional $100 charge will be added to this armoire’s total cost. The function will calculate the charge for the Armoire and return it to the main program for display.
- If the customer does NOT want the premium version of the Armoire, a function called calc_Charge (same name as in option 1) will be called, passing the base price of the Armoire and the charge for cubic volume. The function will calculate the charge for the Armoire and return it to the main program for display.
- You will need to determine if any other arguments are passed into these functions. If there are additional arguments, you must ensure that the argument lists for each of the two functions called calc_Charge are sufficiently different enough for them to still be considered overloaded.
The main program will then display the type of Armoire (Premium or basic), along with all options chosen (including whether the Teak Lining was chosen as an option) and the costs for each option. If the large cubic volume charge is incurred, that needs to be listed as well.
input validation: Do not accept any values for the dimensions less than 18 inches, nor more than 60 inches for the height.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

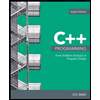
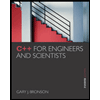
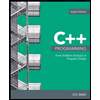
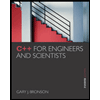