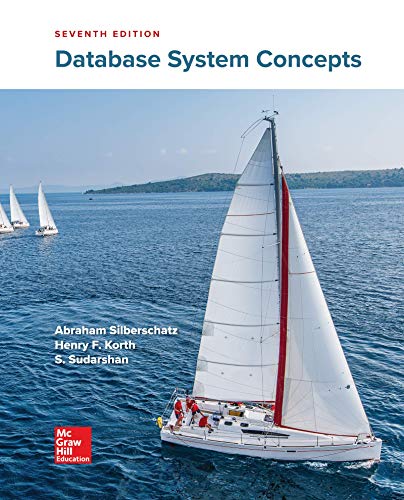
Concept explainers
This is Java Programming! You need to make a BstMaxHeap.java file.
Instructions: Make a file named BstMaxHeap that will implement that implements the Driver.java and MaxHeapInterface.java. The codes are below. If you haven't looked at the code, look at them now.
Driver.java:
/**
A driver that demonstrates the class BstMaxHeap.
@author Frank M. Carrano
@author Timothy M. Henry
@version 5.0
*/
public class Driver
{
public static void main(String[] args)
{
String jared = "Jared";
String brittany = "Brittany";
String brett = "Brett";
String doug = "Doug";
String megan = "Megan";
String jim = "Jim";
String whitney = "Whitney";
String matt = "Matt";
String regis = "Regis";
MaxHeapInterface<String> aHeap = new BstMaxHeap<>();
aHeap.add(jared);
aHeap.add(brittany);
aHeap.add(brett);
aHeap.add(doug);
aHeap.add(megan);
aHeap.add(jim);
aHeap.add(whitney);
aHeap.add(matt);
aHeap.add(regis);
if (aHeap.isEmpty())
System.out.println("The heap is empty - INCORRECT");
else
System.out.println("The heap is not empty; it contains " +
aHeap.getSize() + " entries.");
System.out.println("The largest entry is " + aHeap.getMax());
System.out.println("\n\nRemoving entries in descending order:");
while (!aHeap.isEmpty())
System.out.println("Removing " + aHeap.removeMax());
System.out.println("\n\nTesting constructor with array parameter:\n");
String[] nameArray = {jared, brittany, brett, doug, megan,
jim, whitney, matt, regis};
MaxHeapInterface<String> anotherHeap = new BstMaxHeap<>(nameArray);
if (anotherHeap.isEmpty())
System.out.println("The heap is empty - INCORRECT");
else
System.out.println("The heap is not empty; it contains " +
anotherHeap.getSize() + " entries.");
System.out.println("The largest entry is " + anotherHeap.getMax());
System.out.println("\n\nRemoving entries in descending order:");
while (!anotherHeap.isEmpty())
System.out.println("Removing " + anotherHeap.removeMax());
} // end main
} // end Driver
MaxHeapInterface.java:
/**
An interface for the ADT maxheap.
@author Frank M. Carrano
@author Timothy M. Henry
@version 5.0
*/
public interface MaxHeapInterface<T extends Comparable<? super T>>
{
/** Adds a new entry to this heap.
@param newEntry An object to be added. */
public void add(T newEntry);
/** Removes and returns the largest item in this heap.
@return Either the largest object in the heap or,
if the heap is empty before the operation, null. */
public T removeMax();
/** Retrieves the largest item in this heap.
@return Either the largest object in the heap or,
if the heap is empty, null. */
public T getMax();
/** Detects whether this heap is empty.
@return True if the heap is empty, or false otherwise. */
public boolean isEmpty();
/** Gets the size of this heap.
@return The number of entries currently in the heap. */
public int getSize();
/** Removes all entries from this heap. */
public void clear();
} // end MaxHeapInterface
This is the output we're suppsed to get after we make the BstMaxHeap file:
/*
The heap is not empty; it contains 9 entries.
The largest entry is Whitney
Removing entries in descending order:
Removing Whitney
Removing Regis
Removing Megan
Removing Matt
Removing Jim
Removing Jared
Removing Doug
Removing Brittany
Removing Brett
Testing constructor with array parameter:
The heap is not empty; it contains 9 entries.
The largest entry is Whitney
Removing entries in descending order:
Removing Whitney
Removing Regis
Removing Megan
Removing Matt
Removing Jim
Removing Jared
Removing Doug
Removing Brittany
*/

Trending nowThis is a popular solution!
Step by stepSolved in 6 steps with 2 images

- Java prgm basedarrow_forwardThe instructions is on the right side.arrow_forwardIn Java Write a Fraction class that implements these methods:• add ─ This method receives a Fraction parameter and adds the parameter fraction to thecalling object fraction.• multiply ─ This method receives a Fraction parameter and multiplies the parameterfraction by the calling object fraction.• print ─ This method prints the fraction using fraction notation (1/4, 21/14, etc.)• printAsDouble ─ This method prints the fraction as a double (0.25, 1.5, etc.)• Separate accessor methods for each instance variable (numerator , denominator ) in theFraction classProvide a driver class (FractionDemo) that demonstrates this Fraction class. The driver class shouldcontain this main method : public static void main(String[] args) { Scanner stdIn = new Scanner(System.in); Fraction c, d, x; // Fraction objects System.out.println("Enter numerator; then denominator."); c = new Fraction(stdIn.nextInt(), stdIn.nextInt()); c.print(); System.out.println("Enter numerator; then denominator."); d = new…arrow_forward
- #4. Task Programming Language and Technologies▪ C# Programming Language.▪ Visual Studio 2019. Build a method called Encrypt to receive a string and return another string.The method will be a static.Build a method called Decrypt to return original string.The encryption method will be a very simple one: to encrypt add 1 to eachcharacter and to decrypt subtract 1 to each character.Note: we do not need to create any object of type Encrypter..arrow_forwardIn Java Write a Fraction class that implements these methods:• add ─ This method receives a Fraction parameter and adds the parameter fraction to thecalling object fraction.• multiply ─ This method receives a Fraction parameter and multiplies the parameterfraction by the calling object fraction.• print ─ This method prints the fraction using fraction notation (1/4, 21/14, etc.)• printAsDouble ─ This method prints the fraction as a double (0.25, 1.5, etc.)• Separate accessor methods for each instance variable (numerator , denominator ) in theFraction classProvide a driver class (FractionDemo) that demonstrates this Fraction class. The driver class shouldcontain this main method : public static void main(String[] args) { Scanner stdIn = new Scanner(System.in); Fraction c, d, x; // Fraction objects System.out.println("Enter numerator; then denominator."); c = new Fraction(stdIn.nextInt(), stdIn.nextInt()); c.print(); System.out.println("Enter numerator; then denominator."); d = new…arrow_forwardMake any necessary modifications to the RushJob class so that it can be sorted by job number. Modify the JobDemo3 application so the displayed orders have been sorted. Save the application as JobDemo4. An example of the program is shown below: Enter job number 22 Enter customer name Joe Enter description Powerwashing Enter estimated hours 4 Enter job number 6 Enter customer name Joey Enter description Painting Enter estimated hours 8 Enter job number 12 Enter customer name Joseph Enter description Carpet cleaning Enter estimated hours 5 Enter job number 9 Enter customer name Josefine Enter description Moving Enter estimated hours 12 Enter job number 21 Enter customer name Josefina Enter description Dog walking Enter estimated hours 2 Summary: RushJob 6 Joey Painting 8 hours @$45.00 per hour. Rush job adds 150 premium. Total price is $510.00 RushJob 9 Josefine Moving 12 hours @$45.00 per hour. Rush job adds 150 premium. Total price is $690.00 RushJob 12 Joseph Carpet cleaning 5 hours…arrow_forward
- 11. Which among the following classes are used in decoding data? They don't have to be used together every time. Select all answers that apply. Take note that this is a right minus wrong question. Which among the following classes are used in decoding data? They don't have to be used together every time. Select all answers that apply. Take note that this is a right minus wrong question. 1. PropertyListDecoder 2.JSONDecoder 3.URL 4. FileDecoderarrow_forward8) Now use the Rectangle class to complete the following tasks: - Create another object of the Rectangle class named box2 with a width of 100 and height of 50. Note that we are not specifying the x and y position for this Rectangle object. Hint: look at the different constructors) Display the properties of box2 (same as step 7 above). - Call the proper method to move box1 to a new location with x of 20, and y of 20. Call the proper method to change box2's dimension to have a width of 50 and a height of 30. Display the properties of box1 and box2. - Call the proper method to find the smallest intersection of box1 and box2 and store it in reference variable box3. - Calculate and display the area of box3. Hint: call proper methods to get the values of width and height of box3 before calculating the area. Display the properties of box3. 9) Sample output of the program is as follow: Output - 1213 Module2 (run) x run: box1: java.awt. Rectangle [x=10, y=10,width=40,height=30] box2: java.awt.…arrow_forwardBAGEL FILES import javax.swing.JFrame; public class Bagel{//-----------------------------------------------------------------// Creates and displays the controls for a bagel shop.//-----------------------------------------------------------------public static void main (String[] args){JFrame frame = new JFrame ("Bagel Shop");frame.setDefaultCloseOperation (JFrame.EXIT_ON_CLOSE); frame.getContentPane().add(new BagelControls()); frame.pack();frame.setVisible(true);}} import java.awt.*;import java.awt.event.*; import javax.swing.*; public class BagelControls extends JPanel{private JComboBox bagelCombo;private JButton calcButton;private JLabel cost;private double bagelCost;public BagelControls(){String[] types = {"Make A Selection...", "Plain","Asiago Cheese", "Cranberry"};bagelCombo = new JComboBox(types);calcButton = new JButton("Calc");cost = new JLabel("Cost = " + bagelCost);setPreferredSize (new Dimension (400,…arrow_forward
- Using p5* JavaScript on "openprocessing" websitearrow_forwardInteger userValue is read from input. Assume userValue is greater than 1000 and less than 99999. Assign tensDigit with userValue's tens place value. Ex: If the input is 15876, then the output is: The value in the tens place is: 7 2 3 public class ValueFinder { 4 5 6 7 8 9 10 11 12 13 GHE 14 15 16} public static void main(String[] args) { new Scanner(System.in); } Scanner scnr int userValue; int tensDigit; int tempVal; userValue = scnr.nextInt(); Your code goes here */ 11 System.out.println("The value in the tens place is: + tensDigit);arrow_forwardPlease help me with this using java. Please do not make the code complex as I am a beginnerarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
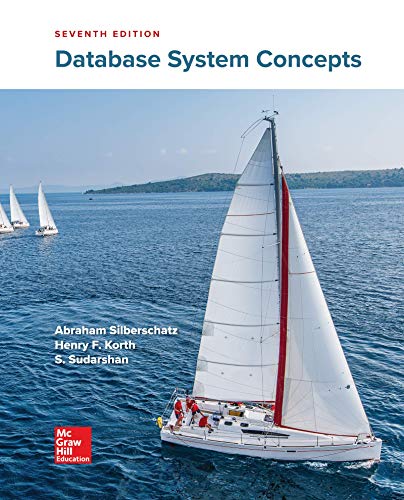
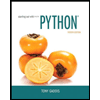
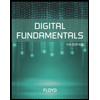
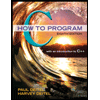
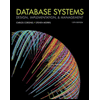
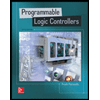