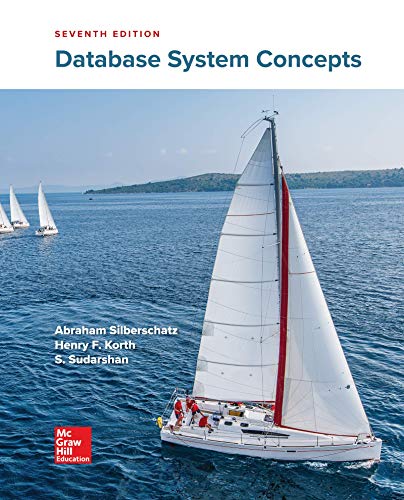
Concept explainers
JAVA Program ASAP
Modify this program below so it is a Filesorting.java program so it passes all the test cases when I upload it to Hypergrade. Also there needs to be NO MAIN METHOD IN THE Filesorting.java program. I have provided the failed test cases a screenshot.
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
public class FileSorting {
public static void main(String[] args) {
try {
BufferedReader reader = new BufferedReader(new FileReader(getFileName()));
String line;
while ((line = reader.readLine()) != null) {
processAndPrintSortedLine(line);
}
reader.close();
} catch (IOException e) {
System.out.println("Error reading the file: " + e.getMessage());
}
}
private static String getFileName() {
String fileName;
java.util.Scanner scanner = new java.util.Scanner(System.in);
do {
System.out.println("Please enter the file name or type QUIT to exit:");
fileName = scanner.nextLine();
if (fileName.equalsIgnoreCase("QUIT")) {
System.exit(0);
}
if (!fileExists(fileName)) {
System.out.println("File " + fileName + " is not found. Please re-enter the file name.");
}
} while (!fileExists(fileName));
return fileName;
}
private static boolean fileExists(String fileName) {
try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) {
return true;
} catch (IOException e) {
return false;
}
}
private static void processAndPrintSortedLine(String line) {
String[] tokens = line.split(",");
ArrayList<Integer> numbers = new ArrayList<>();
for (String token : tokens) {
try {
numbers.add(Integer.parseInt(token));
} catch (NumberFormatException ignored) {
// Handle non-integer tokens here, if needed
}
}
// Sorting the numbers
Collections.sort(numbers);
// Convert ArrayList<Integer> to CharSequence[]
CharSequence[] charSequences = new CharSequence[numbers.size()];
for (int i = 0; i < numbers.size(); i++) {
charSequences[i] = numbers.get(i).toString();
}
// Print the sorted numbers on the console
System.out.println(String.join(",", charSequences));
}
}
input2x2.csv
-67,-11
-27,-70
input1.csv
10
input10x10.csv
56,-19,-21,-51,45,96,-46
-27,29,-58,85,8,71,34
50,51,40,50,100,-82,-87
-47,-24,-27,-32,-25,46,88
-47,95,-41,-75,85,-16,43
-78,0,94,-77,-69,78,-25
-80,-31,-95,82,-86,-32,-22
68,-52,-4,-68,10,-14,-89
26,33,-59,-51,-48,-34,-52
-47,-24,80,16,80,-66,-42
input0.csv
Test Case 1
input1.csvENTER
10\n
Test Case 2
input2x2.csvENTER
-67,-11\n
-70,-27\n
Test Case 3
input10x10.csvENTER
-51,-46,-21,-19,45,56,96\n
-58,-27,8,29,34,71,85\n
-87,-82,40,50,50,51,100\n
-47,-32,-27,-25,-24,46,88\n
-75,-47,-41,-16,43,85,95\n
-78,-77,-69,-25,0,78,94\n
-95,-86,-80,-32,-31,-22,82\n
-89,-68,-52,-14,-4,10,68\n
-59,-52,-51,-48,-34,26,33\n
-66,-47,-42,-24,16,80,80\n
Test Case 4
input0.csvENTER
File input0.csv is empty.\n
Test Case 5
input2.csvENTER
File input2.csv is not found.\n
Please re-enter the file name or type QUIT to exit:\n
input1.csvENTER
10\n
Test Case 6
quitENTER
Test Case 7
input2.csvENTER
File input2.csv is not found.\n
Please re-enter the file name or type QUIT to exit:\n
QUITENTER
![Test Case 1 Failed Show what's missing
Error: Main method not found in class Sort, please define the main me... OUTPUT TOO LONG
Test Case 2 Failed Show what's missing
Error: Main method not found in class Sort, please define the main method as:\n
publ... OUTPUT TOO LONG
Test Case 3 Failed Show what's missing
Error: Main method not found in class Sort, please define the main method as: \n
public static void main(String[] args) \n
or a JavaFX application class must extend javafx.application. Application\n
Test Case 4 Failed Show what's missing D
Error: Main method not found in class Sort, please define the main method as: \n
public static... OUTPUT TOO LONG](https://content.bartleby.com/qna-images/question/c3fe77f3-9d48-40aa-bb9c-abf8aedf62a2/56b27925-d9ac-4455-9847-8ee6442bb45a/si336vv_thumbnail.png)
![Test Case 5 Failed Show what's missing
Error: Main method not found in class Sort, please define the main method as: \n
public static void main (String[] args)\n
or a JavaFX application class must extend javafx.ap.….. OUTPUT TOO LONG
Test Case 6 Failed Show what's missing
Error: Main method not found in class Sort, please define t... OUTPUT TOO LONG
Test Case 7 Failed Show what's missing
Error: Main method not found in class Sort, please define the main method as: \n
public static void main(String[] args)\n
or a JavaFX application class must extend... OUTPUT TOO LONG](https://content.bartleby.com/qna-images/question/c3fe77f3-9d48-40aa-bb9c-abf8aedf62a2/56b27925-d9ac-4455-9847-8ee6442bb45a/jilwqtg_thumbnail.png)

Step by stepSolved in 4 steps with 7 images

- Im trying ro read a csv file and store it into a 2d array but im getting an error when I run my java code. my csv file contains 69 lines of data Below is my code: import java.util.Scanner; import java.util.Arrays; import java.util.Random; import java.io.File; import java.io.FileNotFoundException; import java.io.FilenameFilter; public class CompLab2 { public static String [][] getEarthquakeDatabase (String Filename) { //will read the csv file and convert it to a string 2-d array String [][] Fileinfo = new String [69][22]; int counter = 0; File file = new File(Filename); try { Scanner scnr = new Scanner(file); scnr.nextLine(); //skips the label in the first row of the file while (scnr.hasNextLine()) { // this while loop will count the number of values in the usgs file counter += 1; // increases by one each time a line is read scnr.nextLine(); } while…arrow_forwardI need help with a Java program described below: import java.util.Scanner;import java.io.FileInputStream;import java.io.IOException; public class LabProgram { public static void main(String[] args) throws IOException { Scanner scnr = new Scanner(System.in); /* Type your code here. */ }}arrow_forwardJava provides a mechanism that enables a program to be executed from the command line, as an application. Java runtime execution works on the basis that the class executed must have a: (constructor? inner class? method?) named `main’ that is: (final? static? local?) , public and void. This should take an : (StringBuffer? ArrayList? Array?) of Strings as its only argument. Execution is begun by (calling this method? clicking the BlueJ Run button? selecting this method from the dropdown menu?) . Select the correct answer.arrow_forward
- can someone help me resize the picture in Java. This is my code: import java.awt.*;import java.awt.image.BufferedImage;import java.io.File;import java.io.IOException;import javax.imageio.ImageIO; class ImageDisplayGUI { public static void main(String[] args) { SwingUtilities.invokeLater(() -> { createAndShowGUI(); }); } private static void createAndShowGUI() { JFrame frame = new JFrame("Bears"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(800, 600); frame.setLayout(new BorderLayout()); // Load and display the JPG image try { BufferedImage image = ImageIO.read(new File("two bears.png")); // Replace with your image path ImageIcon icon = new ImageIcon(image); JLabel imageLabel = new JLabel(icon); frame.add(imageLabel, BorderLayout.CENTER); } catch (IOException e) { // Handle image loading error…arrow_forwardIn Java inside class StaticMethods, create the static method menuInteger that displays a menu for the user to select 1000 , 2000 or 3000 The method returns the INTEGER( int) entered, ONLY when the user types 1000, 2000 or 3000, otherwise prints a message and stays in a loop. Test it from main().arrow_forwardPlease help how do I make the code show on the black screen instead of a txt.file. By the way I have already created a txt.file in this java compiler called Data.txtarrow_forward
- Java Netbeansarrow_forwardJAVA PROGRAM PLEASE FIX AND MODIFY THIS JAVA SO WHEN I UPLOAD IT TO HYPERGRADE IT PASSES ALL TEST CASSES PLEASES. RIGHT NOW IT SAYS 0 OUT 3 PASSED. THE PICTURES THAT I PROVIDED PROOF THAT WHEN I UPLOAD IT TO HYPERGRADES IT FAILS TEST CASSES. THANK YOU. import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;import java.util.InputMismatchException;import java.util.Locale;import java.util.Scanner;public class FileTotalAndAverage { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); String fileName; do { System.out.print("Please enter the file name: "); fileName = scanner.nextLine(); try { // Read numbers from the file double[] numbers = readNumbersFromFile(fileName); if (numbers != null) { // Calculate total and average double total = calculateTotal(numbers);…arrow_forwardMy java code keeps giving me back StringIndexOutOfBounds for the following code. Need help dealing with this Movie.java: import java.io.File;import java.io.FileNotFoundException;import java.util.ArrayList;import java.util.Scanner;public class Movie{public String name;public int year;public String genre;public static ArrayList<Movie> loadDatabase() throws FileNotFoundException {ArrayList<Movie> result=new ArrayList<>();File f=new File("db.txt");Scanner inputFile=new Scanner(f);while(inputFile.hasNext()){String name= inputFile.nextLine();int year=inputFile.nextInt();inputFile.nextLine();String genre= inputFile.nextLine();Movie m=new Movie(name, year, genre);//System.out.println(m);result.add(m);}return result;}public Movie(String name, int year, String genre){this.name=name;this.year=year;this.genre=genre;}public boolean equals(int year, String genre){return this.year==year&&this.genre.equals(genre);}public String toString(){return name+" ("+genre+") "+year;}}…arrow_forward
- In Java, please. Complete the Course class by implementing the findStudentHighestGpa() method, which returns the Student object with the highest GPA in the course. Assume that no two students have the same highest GPA. Given classes: Class LabProgram contains the main method for testing the program. Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here.) Class Student represents a classroom student, which has three fields: first name, last name, and GPA. (Hint: getGPA() returns a student's GPA.) Note: For testing purposes, different student values will be used. Ex. For the following students: Henry Nguyen 3.5 Brenda Stern 2.0 Lynda Robison 3.2 Sonya King 3.9 the output is: Top student: Sonya King (GPA: 3.9) LabProgram.java import java.util.Scanner; public class LabProgram { public static void main(String args[]) { Scanner scnr = new Scanner(System.in); Course course = new Course(); int…arrow_forwardImplement the following in the .NET Console App. Write the Bus class. A Bus has a length, a color, and a number of wheels. a. Implement data fields using auto-implemented Properies b. Include 3 constructors: default, the one that receives the Bus length, color and a number of wheels as input (utilize the Properties) and the Constructor that takes the Bus number (an integer) as input.arrow_forwardUsing the Card.java class file, write a program to simulate a Deck of Cards. See Programming Project 8.7 (PP 8.7) from page 403 of your textbook (or view the attached image) for a description of what your program needs to do. Note that although the book description of the problem states that you should write the Card class, I do not want you to do that. You must use the Card file exactly as it is provided (NO modifications) and only write the DeckOfCards and Driver classes. public class Card{public final static int ACE = 1;public final static int TWO = 2;public final static int THREE = 3;public final static int FOUR = 4;public final static int FIVE = 5;public final static int SIX = 6;public final static int SEVEN = 7;public final static int EIGHT = 8;public final static int NINE = 9;public final static int TEN = 10;public final static int JACK = 11;public final static int QUEEN = 12;public final static int KING = 13; public final static int CLUBS = 1;public final static int DIAMONDS =…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
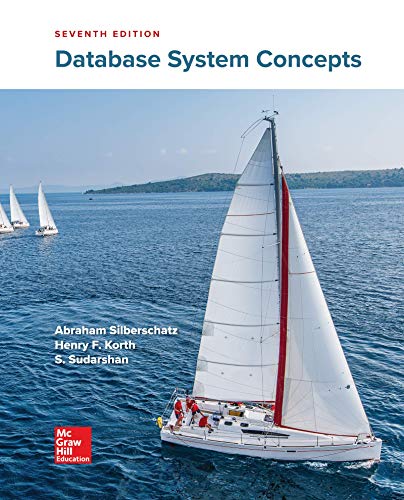
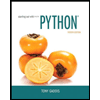
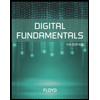
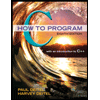
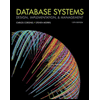
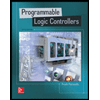