This is part of my hangman simulation in C++. If you compile and run it and type "Easy," the code should run. If you run it though, the body of the hangman doesn't align when you guess wrong. Could you help me with that and implement an if statement to repeat the program if user wants to play again
Hello, This is part of my hangman simulation in C++.
If you compile and run it and type "Easy," the code should run.
If you run it though, the body of the hangman doesn't align when you guess wrong. Could you help me with that and implement an if statement to repeat the program if user wants to play again?
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <string>
#include <iomanip>
using namespace std;
const int MAX_TRIES = 5;
char answer;
int letterFill(char, string, string&);
int main()
{
string name;
char letter;
int num_of_wrong_guesses = 0;
string word;
srand(time(NULL)); // ONLY NEED THIS ONCE!
// welcome the user
cout << "\n\nWelcome to hangman!! Guess a fruit that comes into your mind.";
// Ask user for for Easy, Average, Hard
string level;
cout << "\nChoose a LEVEL(E - Easy, A - Average, H - Hard):" << endl;
cin >> level;
// compare level
if (level == "Easy")
{
//put all the string inside the array here
string easy[] = { "grape", "apple", "banana", "strawberry" };
string word;
int n = rand() % 4;
word = easy[n];
//call the function here for guessing game
// Initialize the secret word with the * character.
string unknown(word.length(), '*');
cout << "\n\nEach letter is represented by an asterisk.";
cout << "\n\nYou have to type only one letter in one try.";
cout << "\n\nYou have " << MAX_TRIES << " tries to try and guess the country.";
cout << "\n~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~";
// Loop until the guesses are used up
while (num_of_wrong_guesses < MAX_TRIES)
{
cout << "\n\n" << unknown;
cout << "\n\nGuess a letter: ";
cin >> letter;
// Fill secret word with letter if the guess is correct,
// otherwise increment the number of wrong guesses.
if (letterFill(letter, word, unknown) == 0)
{
cout << endl << "Whoops! That letter isn't in there!" << endl;
num_of_wrong_guesses++;
if(num_of_wrong_guesses==1){
cout<<" o "<<endl;
}
if(num_of_wrong_guesses==2){
cout<<" o "<<endl;
cout<<"'\'";
}
if(num_of_wrong_guesses==4){
cout<<" \t o "<<endl;
cout<< "'\\' /";
cout<<" | "<<endl;
cout<<"'/' "<<endl;
}
if(num_of_wrong_guesses==3){
cout<<"\t o "<<endl;
cout<<"'\\' /"<<endl;;
cout<<" | "<<endl;
}
}
else
{
cout << endl << "You found a letter! Isn't that exciting?" << endl;
}
// Tell user how many guesses has left.
cout << "You have " << MAX_TRIES - num_of_wrong_guesses;
cout << " guesses left." << endl;
// Check if user guessed the word.
if (word == unknown)
{
cout << word << endl;
cout << "Yeah! You got it!";
break;
}
}
if (num_of_wrong_guesses == MAX_TRIES)
{
cout << "\nSorry, you lose...you've been hanged." << endl;
cout << "The word was : " << word << endl;
cout<<setw(12)<<"\t o "<<endl;
cout<<" '\\' '/'"<<endl;
cout<<setw(12)<<"\t | "<<endl;
cout<<"'/' '\\' "<<endl;
}
cin.ignore();
cin.get();
return 0;
}
}
int letterFill(char guess, string secretword, string &guessword)
{
int i;
int matches = 0;
int len = secretword.length();
for (i = 0; i< len; i++)
{
// Did we already match this letter in a previous guess?
if (guess == guessword[i])
return 0;
// Is the guess in the secret word?
if (guess == secretword[i])
{
guessword[i] = guess;
matches++;
}
}
return matches;
}

Step by step
Solved in 2 steps with 15 images

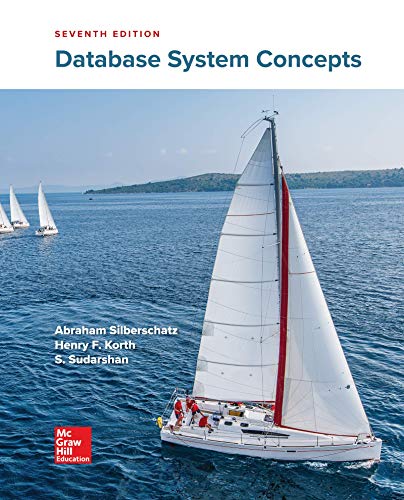
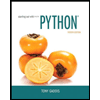
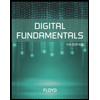
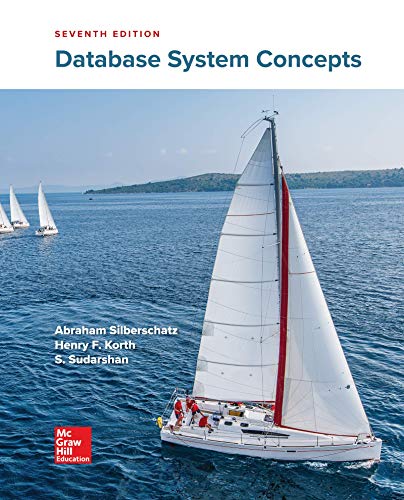
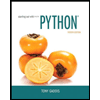
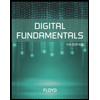
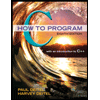
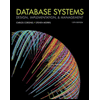
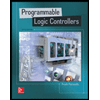