THIS IS WHAT I HAVE SO FAR BUT CANNNOT GET IT TO RUN: business.py class Task: def __init__(self, id=0, description=None, completed=0): self.id = id self.description = description self.completed = completed def __str__(self): if completed==1: return self.id + str(self.description) + "(DONE!)" else: return None #Database layer for CIS3145 by James Porter import sys import os import sqlite3 from contextlib import closing from ch17_objects import Task from objects import Movie conn = None def connect(): global conn if not conn: if sys.platform == "win32": DB_FILE = "task_list_db.sqlite" else: HOME = os.environ["HOME"] DB_FILE = HOME + "/Documents/CIS3145/Week 13/homework/task_list_db.sqlite" conn = sqlite3.connect(DB_FILE) conn.row_factory = sqlite3.Row def close(): if conn: conn.close() def make_task(row): return Task(row["taskID"], row["description"], row["completed"]) def get_tasks(): query = '''SELECT taskID, description as tasks FROM Task''' with closing(conn.cursor()) as c: c.execute(query) results = c.fetchall() tasks = [] for row in results: tasks.append(make_task(row)) return tasks def get_task(task_id): query = '''SELECT taskID, description AS tasks FROM Task WHERE taskID = ?''' with closing(conn.cursor()) as c: c.execute(query, (task_id,)) row = c.fetchone() if row: return make_task(row) else: return None def get_task_by_taskID(task_id): query = '''SELECT taskID, description as selection FROM Task WHERE taskID = ?''' with closing (conn.cursor()) as c: c.execute(query, (task_id, )) results = c.fetchone() task = make_task(row) return task def get_tasks_by_completed(task_id): query = '''SELECT taskID, description AS completed FROM Task WHERE completed = 1''' with closing(conn.cursor()) as c: c.execute(query, (task_id,)) results = c.fetchall() tasks_completed = [] for row in results: tasks_completed.append(make_task(row)) return movies def add_task(task): sql = '''INSERT INTO Task (taskID, description, completed) VALUES (?,?,?)''' with closing(conn.cursor()) as c: c.execute(sql, (Task.taskID, Task.description, Task.completed)) conn.commit() def delete_task(task_id): sql = '''DELETE FROM Task WHERE movieID = ?''' with closing(conn.cursor()) as c: c.execute(sql, (movie_id,)) test = conn.commit() print("Test", test) def delete_task_by_new(): task_id = int(input("Task ID: ")) task = database.get_task_by_taskID() print(task) choice = input("Are you sure you want to delete '" + task.description + "'? (y/n).\n") if choice == "y": db.delete_movie(movie_id) print("'"+task.description +"'was deleted.\n") else: print("'"+task.description +"'was deleted.\n") #Presentation layer for CIS3145 by James Porter import database from business import Task #Variable should store the list of tasks retrieved from the database by history or view methods #Should be incorporated into the delete command so that the user selects from the most recently displayed list list_of_tasks = [] def display_title(): print("Task List") print() display_menu() #Command List of following commands from assignment: view, history, add, #complete, delete, and exit def display_menu(): print("COMMAND MENU") #View should only display tasks that have not been completed #this should be accomplished from a database function which gets list of non-completed tasks print("view - View pending tasks") #History should allow user to view tasks that have been completed, but not deleted. Also should call database function to return list #complete tasks and then displays this list print("history - View completed tasks") #Add command should add new task to the database print("add - Add a task") #Complete command should mark task as completed, not delete from database print("complete - Complete a task") #Delete command should remove a task from the database based on the most recently displayed list of tasks print("delete - Delete a task") #Exit leaves the presentation layer and program print("exit - Exit program") print() def display_tasks(): print("tasks") tasks = database.get_tasks() for task in tasks: print(task.id + ". " + task.description) print() def display_tasks_by_taskID(): task_id = int(input("Task ID: ")) task = database.get_task(task_id) if task == None: print("There is no task with that ID.\n") else: print() tasks = database.get_tasks_by_taskID(task_id) display_tasks(movies, category.name.upper()) def add_task(): description = input("Description: ") task_id = ((get_tasks()).length +1) completed = 0 task = database.get_task(task_id) database.add_task(task) print(description + " was added to database.\n") def delete_task(): task_id = 5 database.delete_task() print("Most recent task was deleted from database.\n") def main(): database.connect() display_title() display_tasks() while True: command = input("Command: ") if command == "view": display_tasks_by_taskID() elif command == "history": get_tasks_by_completed(task_id) elif command == "add": add_task() elif command == "complete": get_task(task_id) elif command == "delete": delete_movie_new() elif command == "exit": break else: print("Not a valid command. Please try again.\n") display_menu() db.close() print("Bye!") if __name__ == "__main__":
THIS IS WHAT I HAVE SO FAR BUT CANNNOT GET IT TO RUN:
business.py
class Task:
def __init__(self, id=0, description=None, completed=0):
self.id = id
self.description = description
self.completed = completed
def __str__(self):
if completed==1:
return self.id + str(self.description) + "(DONE!)"
else:
return None
#
import sys
import os
import sqlite3
from contextlib import closing
from ch17_objects import Task
from objects import Movie
conn = None
def connect():
global conn
if not conn:
if sys.platform == "win32":
DB_FILE = "task_list_db.sqlite"
else:
HOME = os.environ["HOME"]
DB_FILE = HOME + "/Documents/CIS3145/Week 13/homework/task_list_db.sqlite"
conn = sqlite3.connect(DB_FILE)
conn.row_factory = sqlite3.Row
def close():
if conn:
conn.close()
def make_task(row):
return Task(row["taskID"], row["description"], row["completed"])
def get_tasks():
query = '''SELECT taskID, description as tasks
FROM Task'''
with closing(conn.cursor()) as c:
c.execute(query)
results = c.fetchall()
tasks = []
for row in results:
tasks.append(make_task(row))
return tasks
def get_task(task_id):
query = '''SELECT taskID, description AS tasks
FROM Task WHERE taskID = ?'''
with closing(conn.cursor()) as c:
c.execute(query, (task_id,))
row = c.fetchone()
if row:
return make_task(row)
else:
return None
def get_task_by_taskID(task_id):
query = '''SELECT taskID, description as selection
FROM Task WHERE taskID = ?'''
with closing (conn.cursor()) as c:
c.execute(query, (task_id, ))
results = c.fetchone()
task = make_task(row)
return task
def get_tasks_by_completed(task_id):
query = '''SELECT taskID, description AS completed
FROM Task WHERE completed = 1'''
with closing(conn.cursor()) as c:
c.execute(query, (task_id,))
results = c.fetchall()
tasks_completed = []
for row in results:
tasks_completed.append(make_task(row))
return movies
def add_task(task):
sql = '''INSERT INTO Task (taskID, description, completed)
VALUES (?,?,?)'''
with closing(conn.cursor()) as c:
c.execute(sql, (Task.taskID, Task.description, Task.completed))
conn.commit()
def delete_task(task_id):
sql = '''DELETE FROM Task WHERE movieID = ?'''
with closing(conn.cursor()) as c:
c.execute(sql, (movie_id,))
test = conn.commit()
print("Test", test)
def delete_task_by_new():
task_id = int(input("Task ID: "))
task = database.get_task_by_taskID()
print(task)
choice = input("Are you sure you want to delete '" + task.description + "'? (y/n).\n")
if choice == "y":
db.delete_movie(movie_id)
print("'"+task.description +"'was deleted.\n")
else:
print("'"+task.description +"'was deleted.\n")
#Presentation layer for CIS3145 by James Porter
import database
from business import Task
#Variable should store the list of tasks retrieved from the database by history or view methods
#Should be incorporated into the delete command so that the user selects from the most recently displayed list
list_of_tasks = []
def display_title():
print("Task List")
print()
display_menu()
#Command List of following commands from assignment: view, history, add,
#complete, delete, and exit
def display_menu():
print("COMMAND MENU")
#View should only display tasks that have not been completed
#this should be accomplished from a database function which gets list of non-completed tasks
print("view - View pending tasks")
#History should allow user to view tasks that have been completed, but not deleted. Also should call database function to return list
#complete tasks and then displays this list
print("history - View completed tasks")
#Add command should add new task to the database
print("add - Add a task")
#Complete command should mark task as completed, not delete from database
print("complete - Complete a task")
#Delete command should remove a task from the database based on the most recently displayed list of tasks
print("delete - Delete a task")
#Exit leaves the presentation layer and program
print("exit - Exit program")
print()
def display_tasks():
print("tasks")
tasks = database.get_tasks()
for task in tasks:
print(task.id + ". " + task.description)
print()
def display_tasks_by_taskID():
task_id = int(input("Task ID: "))
task = database.get_task(task_id)
if task == None:
print("There is no task with that ID.\n")
else:
print()
tasks = database.get_tasks_by_taskID(task_id)
display_tasks(movies, category.name.upper())
def add_task():
description = input("Description: ")
task_id = ((get_tasks()).length +1)
completed = 0
task = database.get_task(task_id)
database.add_task(task)
print(description + " was added to database.\n")
def delete_task():
task_id = 5
database.delete_task()
print("Most recent task was deleted from database.\n")
def main():
database.connect()
display_title()
display_tasks()
while True:
command = input("Command: ")
if command == "view":
display_tasks_by_taskID()
elif command == "history":
get_tasks_by_completed(task_id)
elif command == "add":
add_task()
elif command == "complete":
get_task(task_id)
elif command == "delete":
delete_movie_new()
elif command == "exit":
break
else:
print("Not a valid command. Please try again.\n")
display_menu()
db.close()
print("Bye!")
if __name__ == "__main__":
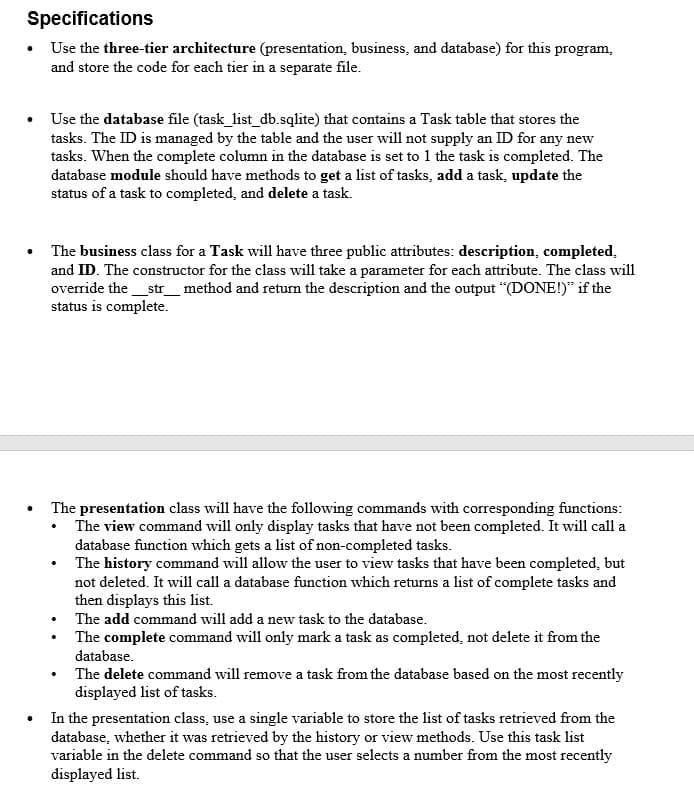
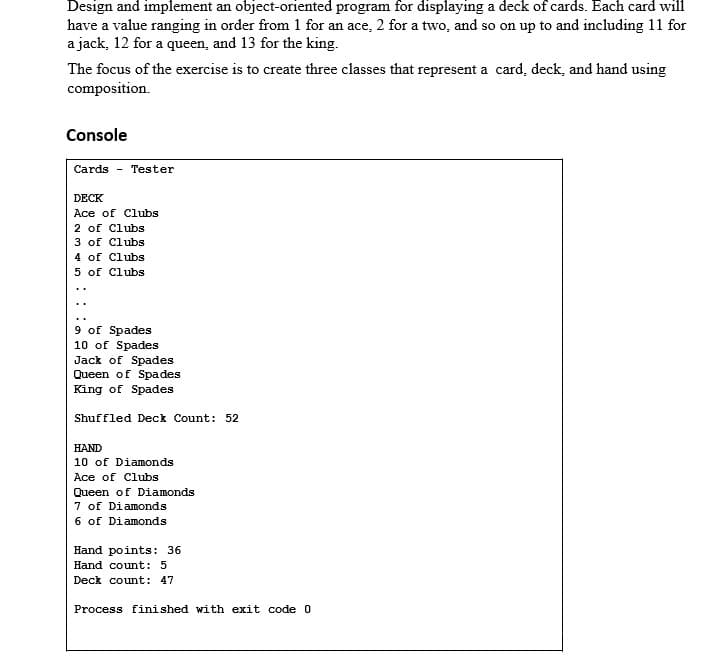

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

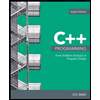
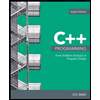