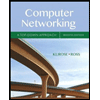
Use the code provided copied to a new file named compulsory_task_1.py:
1. Add another method in the Course class that prints the head office location: Woodstock, Cape Town
2. Create a subclass of the Course class named OOPCourse
3. Create a constructor that initialises the following attributes and assigns these values:
--- "description" with a value "OOP Fundamentals"
--- "trainer" with a value "Mr Anon A. Mouse"
4. Create a method in the subclass named "trainer_details" that prints what the
course is about and the name of the trainer by using the description and trainer attributes.
5. Create a method in the subclass named "show_course_id" that prints the ID number of the course: #12345
6. Create an object of the subclass called course_1 and call the following methods
contact_details
trainer_details
show_course_id
These methods should all print out the correct information to the terminal
On a side note, this task covers single inheritance. multiple inheritance is also possible in Python and
we encourage you to do some research on multiple inheritance when you have finished this course
"""
class Course:
name = "Fundamentals of Computer Science"
contact_website = "www.hyperiondev.com"
def contact_details(self):
print("Please contact us by visiting", self.contact_website)
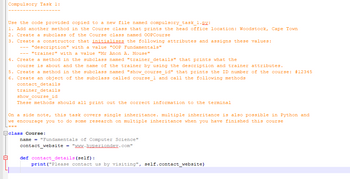

Step by stepSolved in 3 steps with 1 images

- Create a class called fun. Add to the class a static integer variable called count. Add a constructor that has count++; Add a function getCount() { return count ; } Instantiate 4 instance of the class. F1, F2, F3 and F4. Print out the value of count for each instance. What do you note about the static variable called count ? Filename: week6YourNameProgStaticarrow_forwardPlease read all parts of this question before starting the question. Include the completed code for all parts of this question in a file called superheroes.py. NOTE: To implement encapsulation, all of the instance variables/fields should be considered private. Question 2 (a) Whenever folks on Earth get into trouble they look towards the Superheroes Federation to save them. Design and implement a Python class called Hero that includes the following class variable(s), instance variable(s) and method(s) for the class. Class variable(s): • TotalNumHeroes is the total number of heroes listed with the Federation • TotalNumChallengesMet is the total number of challenges that all the federation members have successfully overcome Attribute(s)/instance variable(s): • herolD is the unique ID number for the superhero (default value "N/A") • lifeName is the real-life name of the superhero (e.g. Bruce Wayne) (default value "N/A") • alterEgo is the secondary self or secret identity of the superhero…arrow_forwardread carefully. object must be passed to method not variablesarrow_forward
- 18arrow_forwardJava Code. Create a Driver class to use your Farm and Animal classes and create instances of them. In the main method do the following: Create a Farm of size 10 Create 5 Animal Objects with the details specified in the table below Add the 5 Animal objects to the Farm Call the printDetails method from the Farm to print all the Farm and Animal details.arrow_forwardTest your newly created subclasses inside the same TestComputer program you previouslycreated: create two new Laptops and two new Desktops with the data of your choice. UsetoString() method to show all data fields (including the data fields in the super class and sub class)Hint: Make sure to avoid redundancy in your code – no fields implemented in the Parent Classshould be repeated/reimplemented/redefined in children. package project2;class Desktop extends Computer { private int width; private int height; public Desktop() { super(); width = height = 0; } public Desktop(String manufacturer, String diskSize, String manufacturingDate, int numberOfCores, int width, int height) { super(manufacturer, diskSize, manufacturingDate, numberOfCores); this.width = width; this.height = height; } public int getWidth() { return width; } public int getHeight() { return height; } public void setWidth(int width) {…arrow_forward
- ques7 plz provide handwritten ans asaparrow_forwardCreate three files: Payroll class.java -Base class definition PayrollOvertime.jave - Derived class definition PayrollOvertimeClient.java - contains main() method Implement the two user define classes with the following specifications: Payroll class (the base class) 5 data fields(protected) String name - Initialized in default constructor to "John Doe" int ID - Initialized in default constructor to 9999 doulbe payRate - Initialized in default constructor to 15.0 doulbe hrWorked - Initialized in default constructor to 40 static int data field named nOfObjs for tracking the number of employees, (initialized to 0 and updated nOfObjs++ in the constructors) 2 constructors (public) Default constructor (make sure to update the nOfObjs member) A constructor that accepts the employee’s name, ID, and pay rate as arguments. These values should be assigned to the object’s name, ID, and payRate fields, also update the nOfObjs member 8 public member methods (mutators, accessors, and…arrow_forwardTests: In the test_sun.py module, create tests for the planet objects. You can (and should) do this before you have written any Planet code at all. Implementation: In the sun.py module (see template), implement a Sun class as described in Section 10.4. Your Sun class should include the following methods: __init__, get_mass, get_radius, get_temperature, and __str__. Your __str__ method should include the following information for each planet: Name, distance from sun, radius, and mass. section 10.4arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
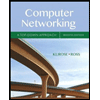
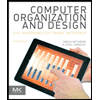
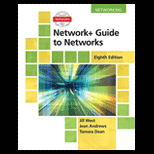
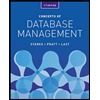
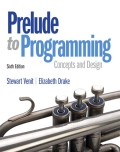
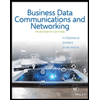