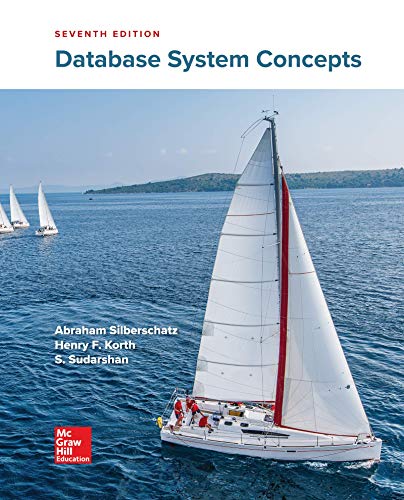
This lab must be done in C++
Assignment:
In cryptography, encryption is the process of encoding a message or information in such a way that only authorized parties can access it. In this lab you will write a program to decode a message that has been encrypted.
Detailed specifications:
Define three classes. Each one should be in a separate file. You can choose to define the class and its functions inline, all in a header file, or have a .h and .cpp file for each class.
- Abstract base class with the following:
- A variable to hold an encrypted message. This variable should be a string which is initialized in the constructor.
- A status variable that will tell whether the message was loaded successfully.
- A constructor that receives one parameter: a string variable with a file name and uploads its content to the string variable that is supposed to store it.
- A pure virtual function called decode. This function will be defined in derived classes.
- A function that prints the message on the screen
- A derived class that implements a version of decode according to the following
algorithm :- input character: abcdefghijklmnopqrstuvwxyz
- decoded character: iztohndbeqrkglmacsvwfuypjx
- That means each 'a' in the input text should be replaced with an 'i', each 'b' with a 'z' and so forth.
- A second derived class that implements a version of decode according
algorithm known as "rotational cypher". In this encryption method, a key is added to each letter of the original text. For example:
Cleartext: A P P L E - Key: 4 4 4 4 4
- Ciphertext: E T T P I
In order to decode, you need to subtract 4.
I will provide a program that tests your classes.
Testing/Output:
Please use the following files as input text files: EncryptedA.txt and EncryptedB.txt
Input Files Content to easily copy:
EncryptedA.txt (Content is paragraph right below):
ifqkwxcadf ar cei fpoi masif cd cei xkdqirr du pxxnwafm pf pnmdkaceo cd p oirrpmi, teaqe rqkpohnir cei gpcp af ac-oplafm ac sikw gauuaqvnc pfg caoi qdfrvoafm, au fdc xkpqcaqpnnw aoxdrrahni, cd gigvqi cei dkamafpn masif dfnw cei ifqdgig gpcp. afxvcr cd cei pnmdkaceo cwxaqpnnw afsdnsi pggacadfpn riqkic gpcp qpnnig liwr, teaqe xkisifcr cei oirrpmi ukdo hiafm giqdgig-isif au cei pnmdkaceo ar xvhnaqnw lfdtf.
EncryptedB.txt (Content is paragraph right below):
mr e wdqqixvmg irgvdtxmsr epksvmxlq, fsxl xli wirhiv erh xli vigmtmirx ywi xli weqi oid (orsbr ew xli wigvix oid) xs irgvdtx erh higvdtx xli qiwweki. sri aivd fewmg wdqqixvmg irgvdtxmsr epksvmxlq mw orsbr ew xli vsxexmsrep gmtliv. mr xlmw epksvmxlq, xli wirhiv wmqtpd "ehhw" xli oid xs iegl glevegxiv sj xli gpievxicx qiwweki xs jsvq xli gmtlivxicx. jsv iceqtpi, mj xli oid mw 2, "e" bsyph figsqi "g", "f" bsyph figsqi "h", erh ws sr. xli vigmtmirx bsyph xlir higvdtx xli qiwweki fd "wyfxvegxmrk" xli oid jvsq iegl glevegxiv sj xli gmtlivxicx xs sfxemr xli svmkmrep qiwweki.
The output should be printed on the screen. Attach the output of your program as a comment at the end of your source code.
Turn in:
Encrypted.h, CypherA.h and CypherB.h (corresponding .cpp files optional)
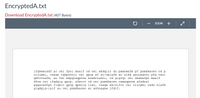
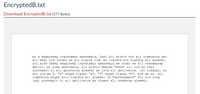

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- In C++ Q1.) Write a Review class that has: • These private data members: • string user: ID of the user • string item: ID of the item • double paid: the price of the item that the user buys • double rating: the rating that the user gives to the item • string review: the review content that the user gives to the item • These public member functions: • A default constructor to set all instance variables to a default value • A parameterized constructor (i.e., a constructor with arguments) to set all instance variables • A method for getting/setting the instance variables. • A method for printing the Review's information.arrow_forwardIn C++, what is the this keyword? a void pointer to the current object a reference to the current object a pointer to the current object a nullptr to the current objectarrow_forwardUsing C++: Write a program that creates a Bus class that includes a bus ID number, number of seats, driver name, and current speed. Create a default constructor without any parameters that initializes the value of ID number, number of seats, and driver name. Create another constructor with three parameters of bus id number, number of seats, and driver name. Car speed should always be initialized as 0. The program will also include the member functions to perform the various operations: modify, set, and display bus information (Bus id, number of seats, driver name, and current speed). For example:- Set the bus id number- Set the number of seats- Set of driver number----------------------------------------------------- Return the bus id number by using get method- Return the number of seats- Return of driver number- Return the current speed-----------------------Member function to display the bus information- busInformation() Also write two member functions, Accelerate() and Brake().…arrow_forward
- in c++ Define a class named “Variable” that manages a variable name (string)and a description (string). It should not provide the default constructor.The class must at least provide the following methods:- toString() method that can return a string in the following format: VAR(<var-name>) DESC(<var-desc>) such as VAR(isSuccessful) DESC(successful flag indicator)- contains() that accepts a search string and it will return true if its variablename or the description contains that string (case-insensitive) and false otherwise.Show how this class being used to create objects and call all its methods and showhow they work.arrow_forwardSubmit code in C++ CODE with comments and output screenshot is must to get Upvote. Thanksarrow_forwardIn C++ create a program that acts like a bank account. It asks the user whether to deposit or withdraw. That has 3 classes. The first class must be a generic class that holds basic info deposit, withdraw, calculate monthly interest rate, and a monthly service fee.The second class must be a savings account class that if the savings account falls below $25 it becomes inactive. The final class must be a checking account if someone withdraws more than what they have a service charge of $15 is added and go into a negative balance. The output should display the basic information how much someone deposited or withdrew and their monthly interest rate.arrow_forward
- Create a User class with the following features (C++): A Private class member fname that is a string A Private class member lname that is a string A setter function setFName(string s) that sets the fname member A setter function setLName(string s) that sets the lname member A getter function getName() that returns the full name with a space between the first and last names Use the following code and save the class in a header file User.hpparrow_forwardC++ Question Hello Please answer the attached C++ programming question correctly, just as the prompt states. Also, make sure that the code is working properly. Thank you.arrow_forwardProblem Statement: Develop an Inventory Management System (IMS) for a small retail business that allows the user to manage their product inventory. The system should enable the user to add, edit, update, and delete product information stored in a .csv file. The IMS should be console-based with a menu-driven interface. Requirements: 1. Classes and Objects: - Create a `Product` class with attributes such as `productID`, `productName`, `price`, `quantity`. - Implement a `InventoryManager` class that will handle operations like adding, editing, updating, and deleting products. - Use a `Main` class with the `main` method to run the program and display the menu. 2. File Operations: - Store product information in a .csv file named `inventory.csv`. - Implement methods in `InventoryManager` for reading and writing to the .csv file. 3. Menu-Driven Interface: - Implement a menu in the `Main` class that allows the user to select operations like Add, Edit, Update, Delete, and View Inventory. - Use…arrow_forward
- a. Write a class BumbleBee that inherits from Insect. Add the public member function void sting() const . This function simply prints "sting!" and a newline. b. Write a class GrassHopper that inherits from Insect. Add the public member function void hop() const . This function simply prints "hop!" and a newline. When you are done your program output should look exactly like the output provided at the end of the file. Do not modify the main program. Do not modify the Insect class.arrow_forwardIn C++ Please: THE PROGRAM CANNOT CONTAIN -> OPERATORS! Create a class AccessPoint with the following: x - a double representing the x coordinate y - a double representing the y coordinate range - an integer representing the coverage radius status - On or Off Add constructors. The default constructor should create an access point object at position (0.0, 0.0), coverage radius 0, and Off. Add accessor and mutator functions: getX, getY, getRange, getStatus, setX, setY, setRange and setStatus. Add a set function that sets the location coordinates and the range. Add the following member functions: move and coverageArea. Add a function overLap that checks if two access points overlap their coverage and returns true if they do. Add a function signalStrength that returns the wireless signal strength as a percentage. The signal strength decreases as one moves away from the access point location. Represent this with bars like, IIIII. Each bar can represent 20% Test your class by writing a…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
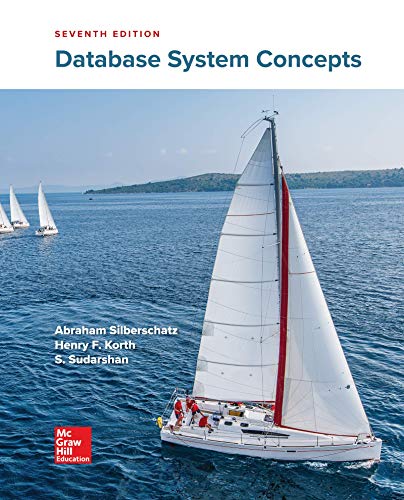
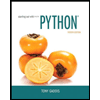
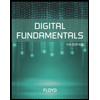
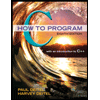
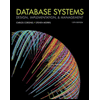
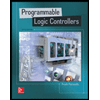