tinue to run until the user picks exit. Functionalities: 1. Each playlist and song node is defined by the structure below: *refer to the photo below* NOTE: You can add additional members that you think may help you finish the exercise. 2. The program must load the data each time it is run and must save the data each time the user picks exit. (a) Saves Data - You must check whether there is a data to be saved. If there are none,
Instruction: Create a program that implements a music playlist using linked lists that is sorted by playlist name. The program must continue to run until the user picks exit.
Functionalities:
1. Each playlist and song node is defined by the structure below: *refer to the photo below* NOTE: You can add additional members that you think may help you finish the exercise.
2. The program must load the data each time it is run and must save the data each time the user picks exit.
(a) Saves Data
- You must check whether there is a data to be saved. If there are none, simply print a prompt saying so. Also, save count 0 so that you will be able to keep track if file is empty.
- You can use whatever format of data you want as long as you will be able to load the data properly.
(b) Load Data
- You must check whether the file exists. If the file exists, check if the count is 0. If the count is not 0, read each of the data in the file and save it in the linked lists.
3. Create a menu that shows:
(a) Add Playlist
- Create an instance of the playlist and ask for the details.Make sure to initialize the members properly. Remember to check that the name of the playlist is unique. If it already exists, it musn’t be added to the system.
- The new playlist node must be added to the linked list in such a way that the list remains sorted by playlist name (alphabetical).
(b) Add Song to Playlist
- Make sure to check if there is a playlist to add to. Ask which playlist to add the song to. If the playlist is found, create an instance of the song and ask for the details. Make sure to initialize the members properly.
- The new song node must be added to the linked list in such a way that the list remains sorted by song title (alphabetical).
(c) Remove Song from Playlist
- Make sure to check if there is a playlist to remove from. Ask which playlist to remove songs from. If the playlist exists, and it isn’t empty, ask which song to remove using the song title and artist. If found, remove the song. Else, print the appropriate prompts.
(d) View a Playlist
- Make sure to check if there is a playlist to print. Ask which playlist to print. If the playlist exists, rint all the details about it along with the songs. Else, print the appropriate prompts.
(e) View all Data
- This simply prints all of the available data in the system. Both the playlist and songs. Make sure to check if there is data to print.
(f) Exit
- When the user picks this, the program terminates. However, before ending the program, all dynamically-allocated variables must be freed first.
4. Each choices except for the exit must be implemented inside a function.
5. Do not forget to put documentation in your code.
![typedef struct song_tag {
char title [20];
char artist [20];
char album [20];
} song;
typedef struct playlist_tag {
char name [50];
Int songCount;
song *songHead;
struct playlist_tag *next;
} playlist;
struct song_tag *nextSong;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1bd2caa7-4030-4543-bf98-cdb517a562be%2F5c2c7cd8-29d3-4656-8f31-1cb8bc9997b6%2Fiwj79kp_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 1 images

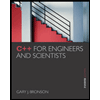
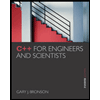