To demonstrate, let's apply the algorithm to n = 100 and see what happens. 1. 100 $ 3 is 1, so our partial answer is "1" 2. 100 / 3 is 33 3. 33 $ 3 is 0, so our partial answer is "01" 4. 33 / 3 is 11 5. 11 3 is 2, so our partial answer is "201" 6. 11 / 3 is 3 7. 3 3 is 0, so our partial answer is "0201" 8. 3 / 3 is 1 1 3 is 1, so our partial answer is "10201" 10. 1 / 3 is 0 9. 11. We reached 0. The final answer is "10201" We can verify tbat the final ans wer is correct because
To demonstrate, let's apply the algorithm to n = 100 and see what happens. 1. 100 $ 3 is 1, so our partial answer is "1" 2. 100 / 3 is 33 3. 33 $ 3 is 0, so our partial answer is "01" 4. 33 / 3 is 11 5. 11 3 is 2, so our partial answer is "201" 6. 11 / 3 is 3 7. 3 3 is 0, so our partial answer is "0201" 8. 3 / 3 is 1 1 3 is 1, so our partial answer is "10201" 10. 1 / 3 is 0 9. 11. We reached 0. The final answer is "10201" We can verify tbat the final ans wer is correct because
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Write Java code please
![C.
Create an int called zeroCount and assign it the value 0. This will count the number of zeroes
in our answer string
d.
Create an int called oneCount and assign it the value 0. This will count the number of ones in
our answer string
Create an int called twoCount and assign it the value 0. This will count the number of twos in
our answer string
е.
f.
Create an int called currentNum and assign it the value initialNum
4. Print initialNum.
5. Create a while loop that terminates when currentNum is equal to 0
Within the while loop, create a local int variable called digit and assign to it currentNum 8 3
7. Use if-else if statements to do the following:
6.
a. If digit is a 0, increment zeroCount
If digit is a 1, increment oneCount
c. If digit is a 2, increment twoCount
Concatenate digit to the front of the string answer
9. Re-assign currentNum to currentNum / 3
b.
8.
10. After the while loop, use three print statements to output the following on separate lines:
a.
"Decimal representation: [initialNum]"
b.
"Ternary representation: [answer]"
"[zeroCount] zeroes, [oneCount] ones, and [twoCount]twos"
C.
11. Create an int variable digitSum and assign it to the sum of the digits in the ternary representation
One way of evaluating this sum is 0* zeroCount +1• oneCount + 2 ° twoCount
12. Finally, use a switch statement to print one of the following statements according to the value of
a.
digitSum % 5:
0: "The ternary digits sum to a multiple of 5!"
1: "The ternary digits almost summed to a multiple 5!"
4: "So close!"
a.
b.
C.
d.
Any other value: "Nope!"
Example Outputs
Please refer to the PE clarification thread on Ed for examples.
HINT: To help debug your code, try inserting print statements in places where variables are changed.
Feature Restrictions
There are a few features and methods in Java that overly simplify the concepts we are trying to teach or break our
auto grader. For that reason, do not use any of the following in your final submission:
var (the reserved keyword)
System.exit
Import Restrictions
You may not import anything for this homework assignment.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5a4b1b2d-f64c-4e21-ba10-42f22541b73f%2Fa1cdd7d6-20a4-4b57-a60d-6b22ed2d3643%2Fwt23rb_processed.jpeg&w=3840&q=75)
Transcribed Image Text:C.
Create an int called zeroCount and assign it the value 0. This will count the number of zeroes
in our answer string
d.
Create an int called oneCount and assign it the value 0. This will count the number of ones in
our answer string
Create an int called twoCount and assign it the value 0. This will count the number of twos in
our answer string
е.
f.
Create an int called currentNum and assign it the value initialNum
4. Print initialNum.
5. Create a while loop that terminates when currentNum is equal to 0
Within the while loop, create a local int variable called digit and assign to it currentNum 8 3
7. Use if-else if statements to do the following:
6.
a. If digit is a 0, increment zeroCount
If digit is a 1, increment oneCount
c. If digit is a 2, increment twoCount
Concatenate digit to the front of the string answer
9. Re-assign currentNum to currentNum / 3
b.
8.
10. After the while loop, use three print statements to output the following on separate lines:
a.
"Decimal representation: [initialNum]"
b.
"Ternary representation: [answer]"
"[zeroCount] zeroes, [oneCount] ones, and [twoCount]twos"
C.
11. Create an int variable digitSum and assign it to the sum of the digits in the ternary representation
One way of evaluating this sum is 0* zeroCount +1• oneCount + 2 ° twoCount
12. Finally, use a switch statement to print one of the following statements according to the value of
a.
digitSum % 5:
0: "The ternary digits sum to a multiple of 5!"
1: "The ternary digits almost summed to a multiple 5!"
4: "So close!"
a.
b.
C.
d.
Any other value: "Nope!"
Example Outputs
Please refer to the PE clarification thread on Ed for examples.
HINT: To help debug your code, try inserting print statements in places where variables are changed.
Feature Restrictions
There are a few features and methods in Java that overly simplify the concepts we are trying to teach or break our
auto grader. For that reason, do not use any of the following in your final submission:
var (the reserved keyword)
System.exit
Import Restrictions
You may not import anything for this homework assignment.
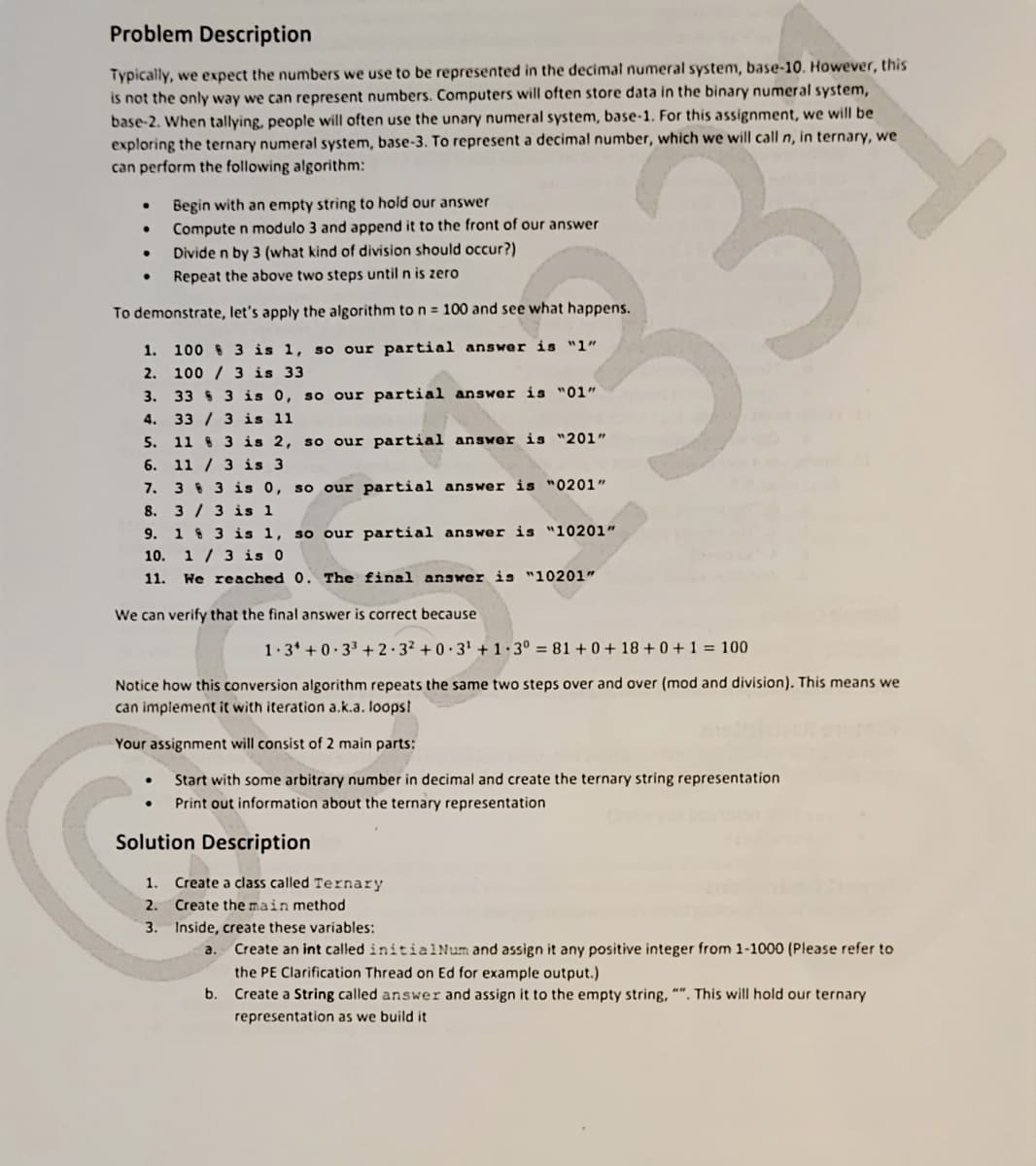
Transcribed Image Text:Problem Description
Typically, we expect the numbers we use to be represented in the decimal numeral system, base-10. However, this
is not the only way we can represent numbers. Computers will often store data in the binary numeral system,
base-2. When tallying, people will often use the unary numeral system, base-1. For this assignment, we will be
exploring the ternary numeral system, base-3. To represent a decimal number, which we will call n, in ternary, we
can perform the following algorithm:
Begin with an empty string to hold our answer
Compute n modulo 3 and append it to the front of our answer
Divide n by 3 (what kind of division should occur?)
Repeat the above two steps until n is zero
To demonstrate, let's apply the algorithm to n = 100 and see what happens.
1. 100 8 3 is 1, so our partial answer is "1"
2. 100 / 3 is 33
3. 33 $ 3 is 0, so our partial answer is "01"
4. 33 / 3 is 11
5.
11 % 3 is 2, so our partial answer is "201"
6. 11 / 3 is 3
3 8 3 is 0, so our partial answer is "0201"
8. 3 / 3 is 1
9. 1 3 is 1, so our partial answer is "10201"
10. 1 / 3 is 0
7.
11.
We reached 0. The final answer is "10201"
We can verify that the final answer is correct because
1.3 + 0• 3³ + 2•3² + 0 •3' +1·3° = 81 + 0 + 18 + 0 + 1 = 100
Notice how this conversion algorithm repeats the same two steps over and over (mod and division). This means we
can implement it with iteration a.k.a. loopst
Your assignment will consist of 2 main parts:
Start with some arbitrary number in decimal and create the ternary string representation
Print out information about the ternary representation
Solution Description
1. Create a class called Ternary
Create the main method
2.
3. Inside, create these variables:
a. Create an int called initialNum and assign it any positive integer from 1-1000 (Please refer to
the PE Clarification Thread on Ed for example output.)
b. Create a String called answer and assign it to the empty string, "". This will hold our ternary
representation as we build it
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
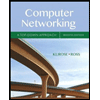
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
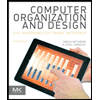
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
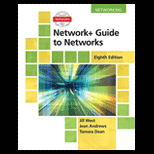
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
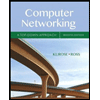
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
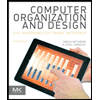
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
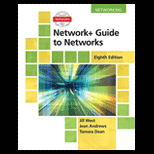
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
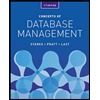
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
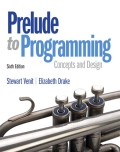
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
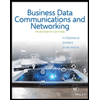
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY