TODO: Polynomial Regression with Ordinary Least Squares (OLS) and Regularization *Please complete the TODOs. * !pip install wget import os import random import traceback from pdb import set_trace import sys import numpy as np from abc import ABC, abstractmethod import traceback from util.timer import Timer from util.data import split_data, feature_label_split, Standardization from util.metrics import mse from datasets.HousingDataset import HousingDataset class BaseModel(ABC): """ Super class for ITCS Machine Learning Class""" @abstractmethod def fit(self, X, y): pass @abstractmethod def predict(self, X): pass class LinearModel(BaseModel): """ Abstract class for a linear model Attributes ========== w ndarray weight vector/matrix """ def __init__(self): """ weight vector w is initialized as None """ self.w = None # check if the matrix is 2-dimensional. if not, raise an exception def _check_matrix(self, mat, name): if len(mat.shape) != 2: raise ValueError(f"Your matrix {name} shape is not 2D! Matrix {name} has the shape {mat.shape}") # add a biases def add_ones(self, X): """ add a column basis to X input matrix """ self._check_matrix(X, 'X') return np.hstack((np.ones((X.shape[0], 1)), X)) #################################################### #### abstract funcitons ############################ @abstractmethod def fit(self, X: np.ndarray, y: np.ndarray): """ train linear model Args: X: Input data y: targets/labels """ pass @abstractmethod def predict(self, X: np.ndarray): """ apply the learned model to input X parameters ---------- X 2d array input data """ pass class PolynomialRegressionRegularized(PolynomialRegression): """ Performs polynomial regression with l2 regularization using the ordinary least squares algorithm attributes: w (np.ndarray): weight matrix that is inherited from OrdinaryLeastSquares degree (int): the number of polynomial degrees to include when adding polynomial features. """ def __init__(self, degree: int, lamb: float): super().__init__(degree) self.lamb = lamb def fit(self, X: np.ndarray, y: np.ndarray) -> None: """ Used to train our model to learn optimal weights. TODO: Finish this method by adding code to perform polynomial regression using the closed form solution OLS with L2 regularization to learn the weights `self.w`. Hint: Add the bias after computing polynomial features. Typically we don't want to include the bias when computing polynomial features. """ pass # TODO replace this line with your code def predict(self, X: np.ndarray) -> np.ndarray: """ Used to make a prediction using the learned weights. TODO: This predict() method is exactly the same as the predict() method in the above class `PolynomialRegression`, so you can just simply copy them here. """ # TODO (REQUIRED) Add code below # TODO (REQUIRED) Store predictions below by replacing np.ones() y_hat = np.ones([len(X), 1]) return y_hat
TODO: Polynomial Regression with Ordinary Least Squares (OLS) and Regularization
*Please complete the TODOs. *
!pip install wget
import os
import random
import traceback
from pdb import set_trace
import sys
import numpy as np
from abc import ABC, abstractmethod
import traceback
from util.timer import Timer
from util.data import split_data, feature_label_split, Standardization
from util.metrics import mse
from datasets.HousingDataset import HousingDataset
class BaseModel(ABC):
""" Super class for ITCS Machine Learning Class"""
@abstractmethod
def fit(self, X, y):
pass
@abstractmethod
def predict(self, X):
pass
class LinearModel(BaseModel):
"""
Abstract class for a linear model
Attributes
==========
w ndarray
weight
"""
def __init__(self):
"""
weight vector w is initialized as None
"""
self.w = None
# check if the matrix is 2-dimensional. if not, raise an exception
def _check_matrix(self, mat, name):
if len(mat.shape) != 2:
raise ValueError(f"Your matrix {name} shape is not 2D! Matrix {name} has the shape {mat.shape}")
# add a biases
def add_ones(self, X):
"""
add a column basis to X input matrix
"""
self._check_matrix(X, 'X')
return np.hstack((np.ones((X.shape[0], 1)), X))
####################################################
#### abstract funcitons ############################
@abstractmethod
def fit(self, X: np.ndarray, y: np.ndarray):
"""
train linear model
Args:
X: Input data
y: targets/labels
"""
pass
@abstractmethod
def predict(self, X: np.ndarray):
"""
apply the learned model to input X
parameters
----------
X 2d array
input data
"""
pass
class PolynomialRegressionRegularized(PolynomialRegression):
"""
Performs polynomial regression with l2 regularization using the ordinary least squares
attributes:
w (np.ndarray): weight matrix that is inherited from OrdinaryLeastSquares
degree (int): the number of polynomial degrees to include when adding
polynomial features.
"""
def __init__(self, degree: int, lamb: float):
super().__init__(degree)
self.lamb = lamb
def fit(self, X: np.ndarray, y: np.ndarray) -> None:
""" Used to train our model to learn optimal weights.
TODO:
Finish this method by adding code to perform polynomial regression using
the closed form solution OLS with L2 regularization to learn
the weights `self.w`.
Hint:
Add the bias after computing polynomial features. Typically we don't want
to include the bias when computing polynomial features.
"""
pass # TODO replace this line with your code
def predict(self, X: np.ndarray) -> np.ndarray:
""" Used to make a prediction using the learned weights.
TODO:
This predict() method is exactly the same as the predict() method in the above class `PolynomialRegression`,
so you can just simply copy them here.
"""
# TODO (REQUIRED) Add code below
# TODO (REQUIRED) Store predictions below by replacing np.ones()
y_hat = np.ones([len(X), 1])
return y_hat

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

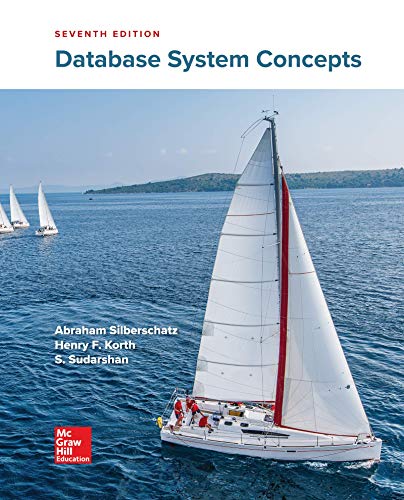
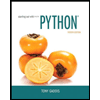
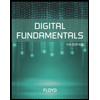
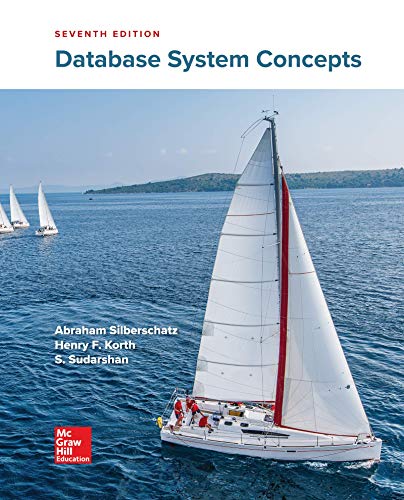
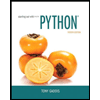
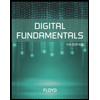
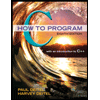
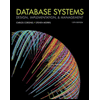
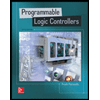