UnsortedMapsOfLists.py: from UnsortedTableMap import UnsortedTableMap class UnsortedMapOfLists(UnsortedTableMap): def __getitem__(self, position: tuple): # Write a comment here to explain why we need this if statement if len(position) == 1: return super().__getitem__(position) key, index = position head: list = super().__getitem_
The UnsortedTableMap class is located in the maps folder. In the attachment, you will find a partially implemented class called UnsortedMapOfLists, which extends UnsortedTableMap. The values associated with the keys in UnsortedMapOfLists are arrays. So you have an array with each key. Off course you can save an array in the value of UnsortedTableMap class without the class we are going to develop, but our class makes this easier, as it allows using a syntax like this:
myMap["somekey", 1] to reach element at index 1 in the array associated with key "somekey". I already implemented methods __setitem__ and __getitem__. Your task is to:
- implement method __delitem__ . Deletion will be performed for an element in the array. If index is not given, delete the array associated with the key.
- implement a function (not a method in the class) to swap the arrays associated with two given keys.
- Add the required comment in method __getitem__.
- implement a main to verify that your code is working properly. You can decide how to do that.
All of these go in the same file.
UnsortedMapsOfLists.py:
from UnsortedTableMap import UnsortedTableMap
class UnsortedMapOfLists(UnsortedTableMap):
def __getitem__(self, position: tuple):
# Write a comment here to explain why we need this if statement
if len(position) == 1:
return super().__getitem__(position)
key, index = position
head: list = super().__getitem__(key)
if index >= len(head):
raise IndexError("Size of array associated with key: ", key, " is: ", len(head))
return head[index]
def __setitem__(self, position: tuple, value):
if len(position) == 1:
super().__setitem__(position, value)
return
key, index = position
try:
head: list = super().__getitem__(key)
if index < len(head):
# overrite exiting value, or append
head[index] = value
elif index == len(head):
head.append(value)
else:
raise IndexError("Size of array associated with key: ", key, " is: ", len(head))
except KeyError:
# new key, create a list for the key
head = [value]
self._table.append(self._Item(key, head))
def __delitem__(self, position: tuple):
"""Remove item associated with key k and index, if index not given, remove the item (array)
associated with the key
raise KeyError if not found
raise IndexError if index out of bound
"""
raise Exception("Implement me")
m = UnsortedMapOfLists()
m["a", 0] = 5
m['a', 0] = 50
m['a', 1] = 60
m['b', 0] = 2
m['c', 1] = 10
m['d'] = [10, 20, 30]
print(m['a', 0])
print(m['a', 1])
print(m['b', 0])
print(m['a'])
print(m['d'])
### Test with the following after you implement the required methods
#del m['b', 0]
#del m['c']
(if needed, here are two modules that support UnsortedMapOfLists.py)
UnsortedTableMap.py:
from MapBase import MapBase
class UnsortedTableMap(MapBase):
"""Map implementation using an unordered list."""
def __init__(self):
"""Create an empty map."""
self._table = [] # list of _Item's
def __getitem__(self, k):
"""Return value associated with key k (raise KeyError if not found)."""
for item in self._table:
if k == item._key:
return item._value
raise KeyError('Key Error: ' + repr(k))
def __setitem__(self, k, v):
"""Assign value v to key k, overwriting existing value if present."""
for item in self._table:
if k == item._key: # Found a match:
item._value = v # reassign value
return # and quit
# did not find match for key
self._table.append(self._Item(k, v))
def __delitem__(self, k):
"""Remove item associated with key k (raise KeyError if not found)."""
for j in range(len(self._table)):
if k == self._table[j]._key: # Found a match:
self._table.pop(j) # remove item
return # and quit
raise KeyError('Key Error: ' + repr(k))
def __len__(self):
"""Return number of items in the map."""
return len(self._table)
def __iter__(self):
"""Generate iteration of the map's keys."""
for item in self._table:
yield item._key # yield the KEY
MapBase.py:
from collections import MutableMapping
class MapBase(MutableMapping):
#------------------------------- nested _Item class -------------------------------
class _Item:
"""Lightweight composite to store key-value pairs as map items."""
__slots__ = '_key', '_value'
def __init__(self, k, v):
self._key = k
self._value = v
def __eq__(self, other):
return self._key == other._key # compare items based on their keys
def __ne__(self, other):
return not (self == other) # opposite of __eq__
def __lt__(self, other):
return self._key < other._key # compare items based on their keys

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

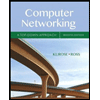
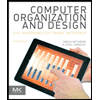
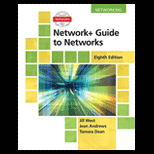
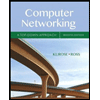
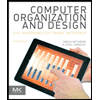
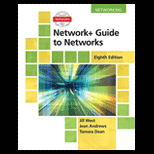
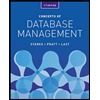
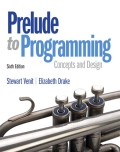
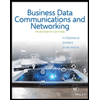