Please use the information in the second screenshot about the BST class and Node class to create a class BSTApp. Please make sure to use the following code as a starting point. import java.util.*; public class BSTTest{ public static void main(String[] args) { // perform at least one test on each operation of your BST } private int[] randomArray(int size) { // remove the two lines int[] arr = new int[1]; return arr; } // the parameters and return are up to you to define; this method needs to be uncommented // private test() { // // } } Base of class Node public class Node { int key; Node left, right, parent; public Node() {} public Node(int num) { key = num; left = null; right = null; parent = null; } } Base of class BST import java.util.*; class BST { // do not change this private Node root; private ArrayList data; // DO NOT MODIFY THIS METHOD public BST() { root = null; data = new ArrayList(0); } // DO NOT MODIFY THIS METHOD public ArrayList getData() { return data; } // DO NOT MODIFY THIS METHOD public void inOrderTraversal() { inOrderTraversal(root); } // DO NOT MODIFY THIS METHOD private void inOrderTraversal(Node node) { if (node != null) { inOrderTraversal(node.left); data.add(node.key); inOrderTraversal(node.right); } } // search public boolean search(int target) { // remove this line return false; } // insert public Node insert(int target) { // remove this line return root; } // note: you may need to implement several supporting methods for delete public Node delete(int target) { // remove this line return root; } // you are welcome to add any supporting methods }
Please use the information in the second screenshot about the BST class and Node class to create a class BSTApp.
Please make sure to use the following code as a starting point.
import java.util.*;
public class BSTTest{
public static void main(String[] args) {
// perform at least one test on each operation of your BST
}
private int[] randomArray(int size) {
// remove the two lines
int[] arr = new int[1];
return arr;
}
// the parameters and return are up to you to define; this method needs to be uncommented
// private test() {
//
// }
}
Base of class Node
public class Node {
int key;
Node left, right, parent;
public Node() {}
public Node(int num) {
key = num;
left = null;
right = null;
parent = null;
}
}
Base of class BST
import java.util.*;
class BST {
// do not change this
private Node root;
private ArrayList<Integer> data;
// DO NOT MODIFY THIS METHOD
public BST() {
root = null;
data = new ArrayList<Integer>(0);
}
// DO NOT MODIFY THIS METHOD
public ArrayList<Integer> getData() {
return data;
}
// DO NOT MODIFY THIS METHOD
public void inOrderTraversal() {
inOrderTraversal(root);
}
// DO NOT MODIFY THIS METHOD
private void inOrderTraversal(Node node) {
if (node != null) {
inOrderTraversal(node.left);
data.add(node.key);
inOrderTraversal(node.right);
}
}
// search
public boolean search(int target) {
// remove this line
return false;
}
// insert
public Node insert(int target) {
// remove this line
return root;
}
// note: you may need to implement several supporting methods for delete
public Node delete(int target) {
// remove this line
return root;
}
// you are welcome to add any supporting methods
}
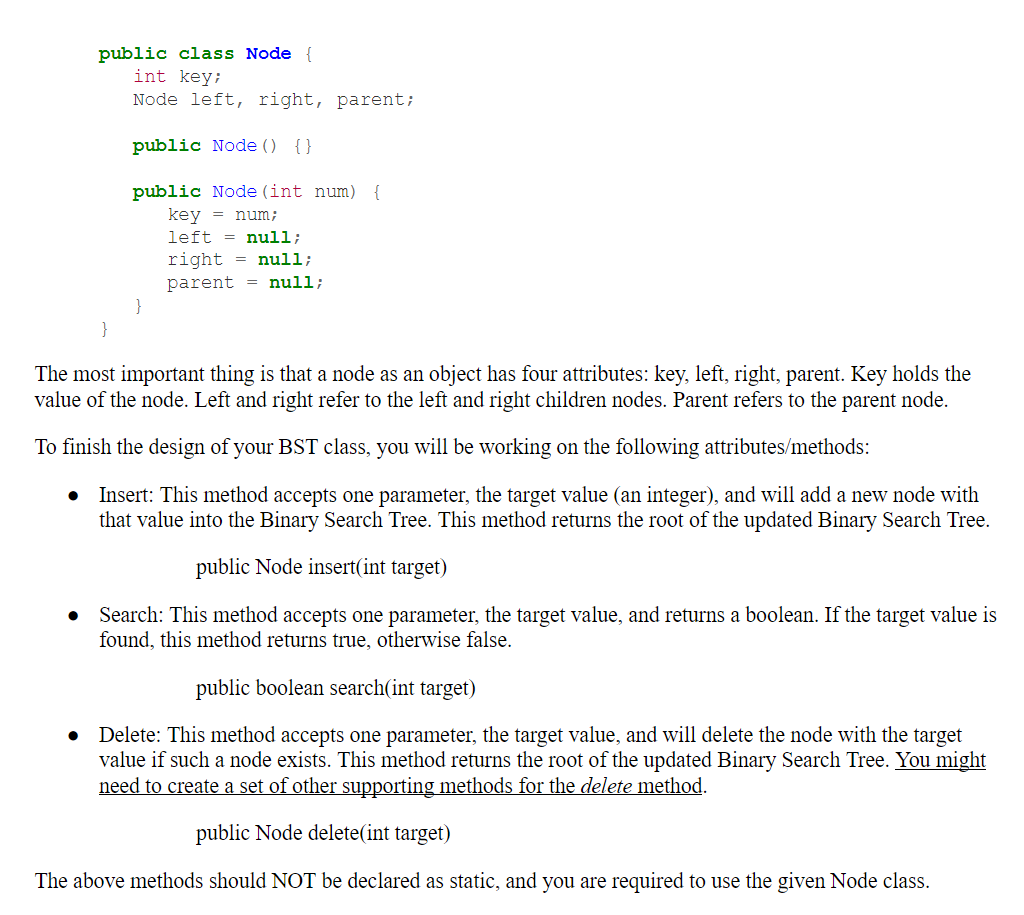
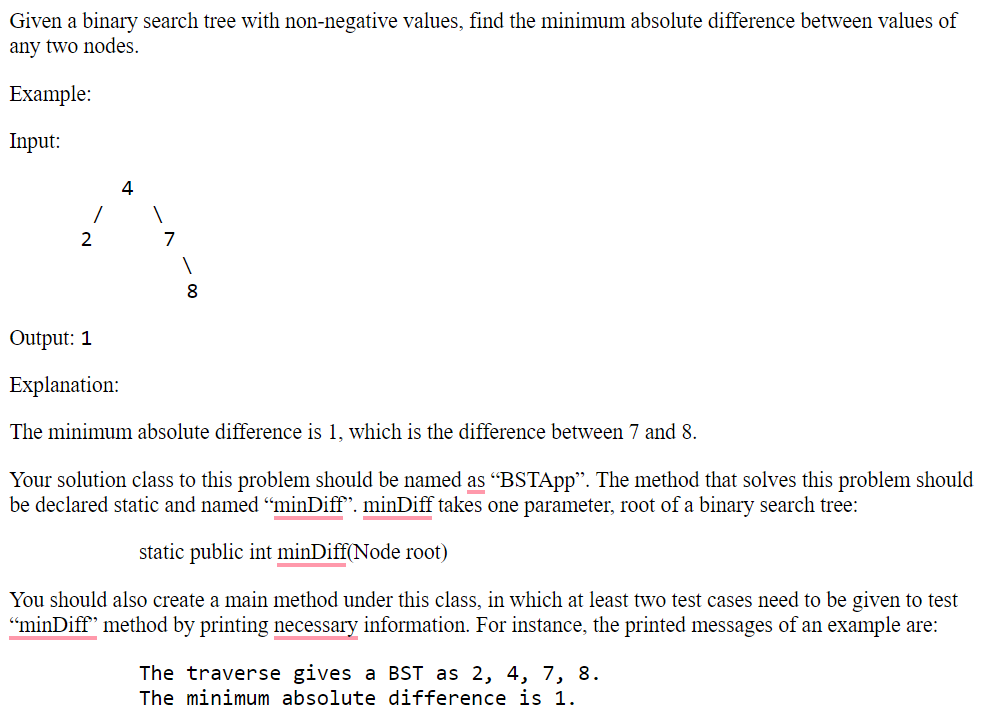

Step by step
Solved in 3 steps

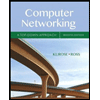
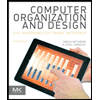
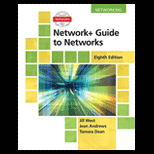
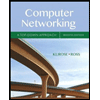
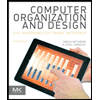
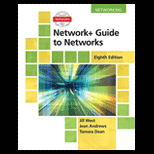
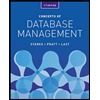
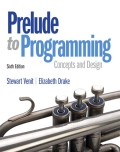
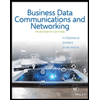