Upon the ordering transaction, display the order details in the tabular format and save the order data to a text file for historical purposes. The order ID will be generated automatically using a sequential number and prefixed with the letter 'P'. Save the order date with the current date. You may use the Calendar class in Java to derive the current date. Apply 5% government sales tax to the gross total of the transaction. Order ID: P1000 No 1 2 Pizza Deluxe Cheese-L Aloha Chicken-R Date: 12/11/2022 Quantity 1 Tax (58) TOTAL (RM) Total 60.00 15.00 3.75 78.75 Figure 3: Pizza Order Page The user may select option 2 in the main menu to view back the previous order details by entering the Order ID. In this case, you should design a class called Order to capture all the information stated in Figure 3.0. Users can place a maximum of up to 8 pizzas per order. You may create another class called Order Line to present each ordered pizza details (e.g. Deluxe Cheese-L and quantity 2) in an order. Currently, all the pizza information is saved into a file called pizza.txt. Create a Pizza entity class to capture the pizza details. The Pizza class should have a name and a price for each different size. Certain pizzas might have different prices although with the same size. There are 4 different prices for each pizza separated by a comma in the file. The following record represents P-8.9, R-16.0, L-26.0 and XL-36.0. Blazing Seafood, 8.9,16.0, 26.0,36.0 However, certain pizza does not have the price for the personal pizza and the system will simply place a zero in the record. Hence, users are not allowed to select their personal pizza on the ordering page. While the program is launched, you are required to load and populate all the pizza details from the given pizza.txt file to the object array. For option 3, the user can add new pizza details including the prices for each pizza size and update the pizza.txt file. Validation should be done for each transaction to avoid logical errors (e.g. prevent negative value for the chocolate weight). Application of advanced OOP concepts such as Inheritance, composition & aggregation will gain higher marks in the overall assessment.
Upon the ordering transaction, display the order details in the tabular format and save the order data to a text file for historical purposes. The order ID will be generated automatically using a sequential number and prefixed with the letter 'P'. Save the order date with the current date. You may use the Calendar class in Java to derive the current date. Apply 5% government sales tax to the gross total of the transaction. Order ID: P1000 No 1 2 Pizza Deluxe Cheese-L Aloha Chicken-R Date: 12/11/2022 Quantity 1 Tax (58) TOTAL (RM) Total 60.00 15.00 3.75 78.75 Figure 3: Pizza Order Page The user may select option 2 in the main menu to view back the previous order details by entering the Order ID. In this case, you should design a class called Order to capture all the information stated in Figure 3.0. Users can place a maximum of up to 8 pizzas per order. You may create another class called Order Line to present each ordered pizza details (e.g. Deluxe Cheese-L and quantity 2) in an order. Currently, all the pizza information is saved into a file called pizza.txt. Create a Pizza entity class to capture the pizza details. The Pizza class should have a name and a price for each different size. Certain pizzas might have different prices although with the same size. There are 4 different prices for each pizza separated by a comma in the file. The following record represents P-8.9, R-16.0, L-26.0 and XL-36.0. Blazing Seafood, 8.9,16.0, 26.0,36.0 However, certain pizza does not have the price for the personal pizza and the system will simply place a zero in the record. Hence, users are not allowed to select their personal pizza on the ordering page. While the program is launched, you are required to load and populate all the pizza details from the given pizza.txt file to the object array. For option 3, the user can add new pizza details including the prices for each pizza size and update the pizza.txt file. Validation should be done for each transaction to avoid logical errors (e.g. prevent negative value for the chocolate weight). Application of advanced OOP concepts such as Inheritance, composition & aggregation will gain higher marks in the overall assessment.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
object-oreinted
need the full code together with the comments.
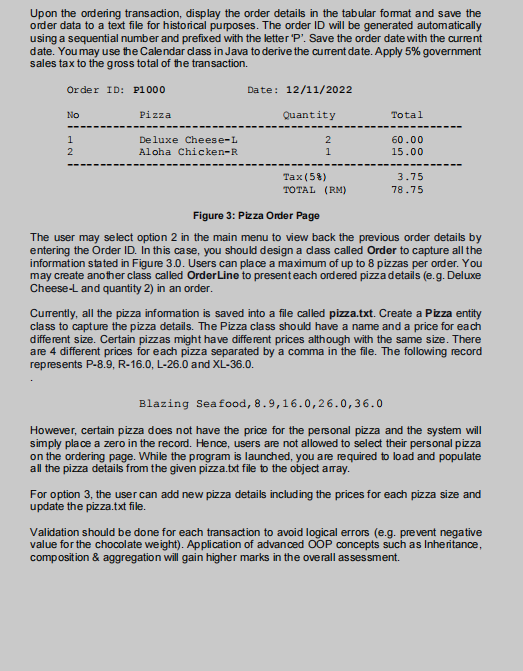
Transcribed Image Text:Upon the ordering transaction, display the order details in the tabular format and save the
order data to a text file for historical purposes. The order ID will be generated automatically
using a sequential number and prefixed with the letter 'P'. Save the order date with the current
date. You may use the Calendar class in Java to derive the current date. Apply 5% government
sales tax to the gross total of the transaction.
Order ID: P1000
No
1
2
Pizza
Deluxe Cheese-L
Aloha Chicken-R
Date: 12/11/2022
Quantity
2
1
Tax (58)
TOTAL (RM)
Total
60.00
15.00
3.75
78.75
Figure 3: Pizza Order Page
The user may select option 2 in the main menu to view back the previous order details by
entering the Order ID. In this case, you should design a class called Order to capture all the
information stated in Figure 3.0. Users can place a maximum of up to 8 pizzas per order. You
may create another class called OrderLine to present each ordered pizza details (e.g. Deluxe
Cheese-L and quantity 2) in an order.
Currently, all the pizza information is saved into a file called pizza.txt. Create a Pizza entity
class to capture the pizza details. The Pizza class should have a name and a price for each
different size. Certain pizzas might have different prices although with the same size. There
are 4 different prices for each pizza separated by a comma in the file. The following record
represents P-8.9, R-16.0, L-26.0 and XL-36.0.
Blazing Seafood, 8.9,16.0,26.0,36.0
However, certain pizza does not have the price for the personal pizza and the system will
simply place a zero in the record. Hence, users are not allowed to select their personal pizza
on the ordering page. While the program is launched, you are required to load and populate
all the pizza details from the given pizza.txt file to the object array.
For option 3, the user can add new pizza details including the prices for each pizza size and
update the pizza.txt file.
Validation should be done for each transaction to avoid logical errors (e.g. prevent negative
value for the chocolate weight). Application of advanced OOP concepts such as Inheritance,
composition & aggregation will gain higher marks in the overall assessment.
![You are about to create a prototype of a Pizza Ordering system using the Java programming
language. Create a simple user interface of the program (figure 1.0) in the console mode
environment. The initial interface is as follows:
Pizza Ordering System
1
1
1
1
1 [Order Pizza
2 [View Order
3 [Add New Pizza
4 [Exit
Enter Option: 1
Figure 1: Main Menu
Use a loop to allow the user to select the option repeatedly until the [Exit] option was selected.
When the user selects option 1, a new menu as shown in Figure 2.0 will be displayed to allow
the user to select the type pizza, size and quantity of the pizza.
<<Pizza Menu>>
1 [Blazing Seafood ]
2 [Chicken Supreme 1
3 [Hawaiian Supreme]
4 [Deluxe Cheese ]
5 [Veggie Lover 1
6 [Aloha Chicken ]
Select Pizza
4
Deluxe Cheese Price List
1 [Personal - RM 8.9 1
2 [Regular - RM 15.0 1
3 [Large - RM 30.0 1
4 [XLarge - RM 45.0 1
Select Pizza Size : 3
Enter Quantity : 2
Continue next pizza [Y/N]:
Figure 2: Pizza Order Page](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5d5dcd17-b353-4e6d-bb82-879436a4091f%2Fb2902bc4-d0f1-46c0-9c44-ea3e4c5a4473%2F52temm5_processed.png&w=3840&q=75)
Transcribed Image Text:You are about to create a prototype of a Pizza Ordering system using the Java programming
language. Create a simple user interface of the program (figure 1.0) in the console mode
environment. The initial interface is as follows:
Pizza Ordering System
1
1
1
1
1 [Order Pizza
2 [View Order
3 [Add New Pizza
4 [Exit
Enter Option: 1
Figure 1: Main Menu
Use a loop to allow the user to select the option repeatedly until the [Exit] option was selected.
When the user selects option 1, a new menu as shown in Figure 2.0 will be displayed to allow
the user to select the type pizza, size and quantity of the pizza.
<<Pizza Menu>>
1 [Blazing Seafood ]
2 [Chicken Supreme 1
3 [Hawaiian Supreme]
4 [Deluxe Cheese ]
5 [Veggie Lover 1
6 [Aloha Chicken ]
Select Pizza
4
Deluxe Cheese Price List
1 [Personal - RM 8.9 1
2 [Regular - RM 15.0 1
3 [Large - RM 30.0 1
4 [XLarge - RM 45.0 1
Select Pizza Size : 3
Enter Quantity : 2
Continue next pizza [Y/N]:
Figure 2: Pizza Order Page
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 6 steps with 2 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
i could not run the
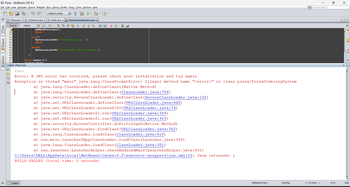
Transcribed Image Text:Pizza - NetBeans IDE 8.2
File Edit View Navigate Source Refactor Run Debug Profile Team Tools Window Help
TT ▷
Files
Projects
Services
2
Pizza.javax OrderLine.javax Order.javax
K
Source
P
36
History
Output - Pizza (run)
DD
|
Output
break;
addNewPiza(scanner);
case 4:
<default config>
break;
PizzaOrderingSystem.java x
27 284 ↑ & B
System.out.println("Exiting the program...):
default:
Jwhile (option !=4);
oranner cloco().
System.out.println("Invalid option. Please try again.");
run:
Error: A JNI error has occurred, please check your installation and try again
Exception in thread "main" java.lang.Class FormatError: Illegal method name "<error>" in class pizza/PizzaOrdering System
at java.lang.ClassLoader.defineClass1 (Native Method)
at java.lang.ClassLoader.defineClass
at java.security.SecureClassLoader.defineClass (SecureClassLoader.java:142)
at java.net. URLClassLoader.defineClass (URLClassLoader.java:468)
at java.net. URLClassLoader.access$100 (URLClassLoader.java:74)
at java.net. URLClassLoader$1.run (URLClassLoader.java:369)
at java.net. URLClassLoader$1.run (URLClassLoader.java:363)
at java.security.Access Controller.doPrivileged (Native Method)
at java.net. URLClassLoader.findClass (URLClassLoader.java:362)
java.lang.ClassLoader.loadClass (ClassLoader.java:418)
at
at sun.misc. Launcher $AppClassLoader.loadClass (Launcher.java:355)
at
java.lang.ClassLoader.loadClass (ClassLoader.java:351)
at sun.launcher. LauncherHelper.checkAndLoadMain (LauncherHelper.java:601)
C:\Users\DELL\AppData\Local\NetBeans\Cache\8.2\executor-snippets\run.xml:53: Java returned: 1
BUILD FAILED (total time: 0 seconds)
W
(ClassLoader.java:756)
litelunch3 (run)
running..
Search (Ctrl+l)
(2 more...)
61:5
X
■
||
INS
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
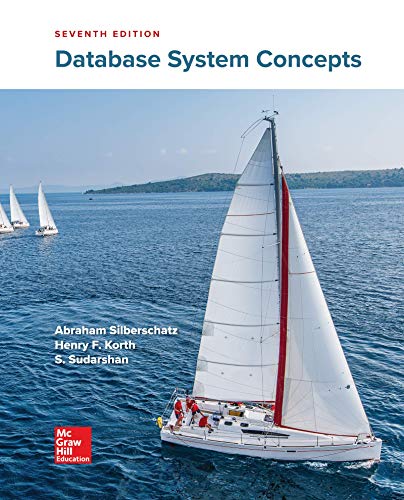
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
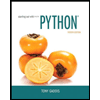
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
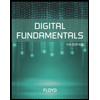
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
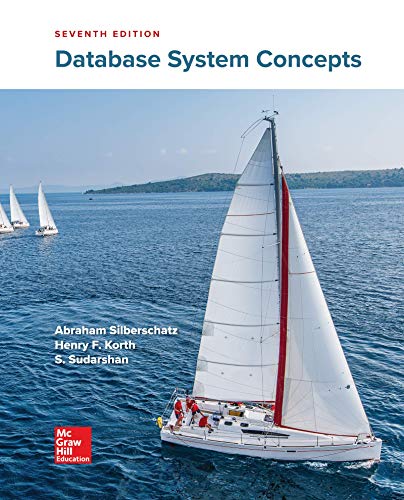
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
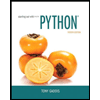
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
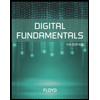
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
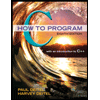
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
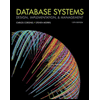
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
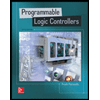
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education