ur team is designing a program to manage all of the cars that are in the inventory. You have been asked to design a class that represents a car. The data that should be kept as attributes in the class are as follows:
Write a code using abstract data types, classes, and inheritance methods as in the example below. Do not use the car class exemplified, but think of any similar use of such a class and adapt it to your idea. Make sure to follow all the requirements set below:
Creating the Car Class
Motorvehicles Solutions, Inc. is a business that sells cars and leasing services. You are a programmer in the company's IT department, and your team is designing a program to manage all of the cars that are in the inventory. You have been asked to design a class that represents a car. The data that should be kept as attributes in the class are as follows:
- The name of the car's manufacturer will be assigned to the __manufact attribute.
- The car's model number will be assigned to the __model attribute
- The car's retail price will be assigned to the __retail_price attribute
- The car's leasing price will be assigned to the __leasing_price attribute
The class will also have the following methods:
- An __init__ method that accepts arguments for the manufacturer, model number, and retail price.
- A set_manufact method that accepts an argument for the manufacturer. This method will allow us to change the value of the __manufact attribute after the object has been created, if necessary.
- A set_model method that accepts an argument for the model. This method will allow us to change the value of the __model attribute after the object has been created, if necessary.
- A set_retail_price method that accepts an argument for the retail price. This method will allow us to change the value of the __retail_price attribute after the object has been created, if necessary.
- A set_leasing_price method that accepts an argument for the leasing term in months. This method will allow us to change the value of the __leasing_price attribute after the object has been created, if necessary. You need to ask about the leasing term/time and calculate the price accordingly. It would be nice to save the loan term as well.
- A get_manufact method that returns the car's manufacturer
- A get_model method that returns the car's model number
- A get_retail_price method that returns the car's retail price
- A get leasing_price method that returns the car's leasing price
- A save_to_file method to save each user entry of manufacturer, model, and price to a text or spreadsheet file; this method should be called at the end of the class to save the final values for manufacturer, model, and price; use append so it will save each combination of those three values entered by the user; create a counter to save to each combination of values, so to distinguish one row/record of the next
The Car Class will be imported into several programs that your team is developing. To test the class, write a code that prompts the user for the car's manufacturer, model number, retail, and leasing price. Make sure you create an outer loop to ask the user if they want to continue entering cars into the '
The program output should look like this:
Enter the manufacturer: Tesla <enter>
Enter the model number: Model S <enter>
Enter the retail price: $104,990 <enter>
Enter the leasing/loan term in months: 24 <enter>
Here is the confirmation of what you need:
Manufacturer: Tesla
Model Number: Model S
Retail Price: $104,990
Leasing Price: $2,482.00 /mo
Do you want to continue adding cars to the database?
When the user quits, display all data saved in the file so far including the counter, which in fact will function as a row number or index.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 6 images

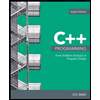
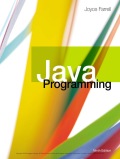
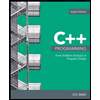
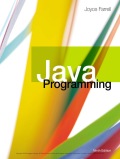