Use C++ and solve the problem without a sorting algorithm. here is the code I have: #include #include using namespace std; struct Node { int data; Node* next; }; void insert(Node** root, int item) { Node* temp = new Node; Node* ptr; temp->data = item; temp->next = NULL; if (*root == NULL) *root = temp; else { ptr = *root; while (ptr->next != NULL) ptr = ptr->next; ptr->next = temp; } } void display(Node* root) { while (root != NULL) { cout << root->data << " "; root = root->next; } cout<
Use C++ and solve the problem without a sorting algorithm. here is the code I have: #include #include using namespace std; struct Node { int data; Node* next; }; void insert(Node** root, int item) { Node* temp = new Node; Node* ptr; temp->data = item; temp->next = NULL; if (*root == NULL) *root = temp; else { ptr = *root; while (ptr->next != NULL) ptr = ptr->next; ptr->next = temp; } } void display(Node* root) { while (root != NULL) { cout << root->data << " "; root = root->next; } cout<
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter8: Arrays And Strings
Section: Chapter Questions
Problem 19PE
Related questions
Question
100%
Use C++ and solve the problem without a sorting
here is the code I have:
#include <iostream>
#include <bits/stdc++.h>
using namespace std;
struct Node {
int data;
Node* next;
};
void insert(Node** root, int item)
{
Node* temp = new Node;
Node* ptr;
temp->data = item;
temp->next = NULL;
if (*root == NULL)
*root = temp;
else
{
ptr = *root;
while (ptr->next != NULL)
ptr = ptr->next;
ptr->next = temp;
}
}
void display(Node* root)
{
while (root != NULL)
{
cout << root->data << " ";
root = root->next;
}
cout<<endl;
}
Node *arrayToList(int arr[], int n)
{
Node *root = NULL;
for (int i = 0; i < n; i++)
insert(&root, arr[i]);
return root;
}
int main()
{
int arr[]={4,3,6,1,10,9,5,8,7,2};
int n = sizeof(arr) / sizeof(arr[0]);
#include <bits/stdc++.h>
using namespace std;
struct Node {
int data;
Node* next;
};
void insert(Node** root, int item)
{
Node* temp = new Node;
Node* ptr;
temp->data = item;
temp->next = NULL;
if (*root == NULL)
*root = temp;
else
{
ptr = *root;
while (ptr->next != NULL)
ptr = ptr->next;
ptr->next = temp;
}
}
void display(Node* root)
{
while (root != NULL)
{
cout << root->data << " ";
root = root->next;
}
cout<<endl;
}
Node *arrayToList(int arr[], int n)
{
Node *root = NULL;
for (int i = 0; i < n; i++)
insert(&root, arr[i]);
return root;
}
int main()
{
int arr[]={4,3,6,1,10,9,5,8,7,2};
int n = sizeof(arr) / sizeof(arr[0]);
for(int i = 1; i <= n; i++)
{
sort(arr, arr+i);
Node* root = arrayToList(arr, i);
display(root);}
return 0;
{
sort(arr, arr+i);
Node* root = arrayToList(arr, i);
display(root);}
return 0;
![◆ The array contains all 10 different integers. Store them in a linked list in
order and print them out.
4
34
346
1 346
1 3 4 6 10
1 3 4 6 9 10
1 3 4 5 6 9 10
1 3 4 5 6 8 9 10
1 3 4 5
6 7 8 9 10
1 2 3 4 5 6 7 8 9 10
int main()
{
}
int A[10] { 4, 3, 6, 1, 10, 9, 5, 8, 7, 2 };
=
return 0;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fcdbdf4c8-5459-45de-99f8-cd8d71031beb%2F6486d429-ad41-4e1d-a992-7bb8f380a74b%2Fl0j7y87_processed.png&w=3840&q=75)
Transcribed Image Text:◆ The array contains all 10 different integers. Store them in a linked list in
order and print them out.
4
34
346
1 346
1 3 4 6 10
1 3 4 6 9 10
1 3 4 5 6 9 10
1 3 4 5 6 8 9 10
1 3 4 5
6 7 8 9 10
1 2 3 4 5 6 7 8 9 10
int main()
{
}
int A[10] { 4, 3, 6, 1, 10, 9, 5, 8, 7, 2 };
=
return 0;
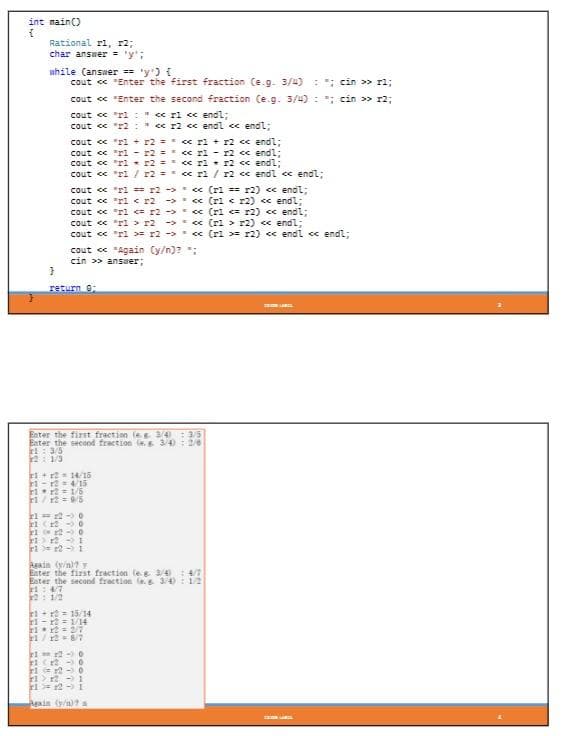
Transcribed Image Text:int main()
{
Rational r1, 2;
char answer = '';
while (answer == 'y') {
cout << "Enter the first fraction (e.g. 3/4); cin >> rl;
cout << "Enter the second fraction (e.g. 3/4) ; cin >> r2;
cout << "1" << r1 << endl;
cout << "r2: << r2 << endl << endl;
cout << "ri + r2 =
cout << "r1 r2 =
cout << "r1
cout << "ri / r2 ==
11-1²
cout << "ri == 12 ->
cout << "r1 r2 →>
cout << "r1 <= r2>
cout << "rl > r2 →>
cout << "ri >= r2>
}
return 0:
1+12=14/15
4/15
cout << "Again (y/n)? *;
cin >> answer;
Enter the first fraction (eg 3/4) : 3/5
Enter the second fraction (e.s 3/4): 2/6
ri: 3/5
21/3
112=1/5
1/2 = 9/5
21-12-0
21 (12
112-0
11 12 1
112-1
1+2= 15/14
1-12 1/14
21x2= 2/7
21/12 = 8/7
<< r1 + x2 << endl;
<< r1
r2 << endl;
r2 << endl;
<< rl/r2 << endl << endl;
r2 == << r1
21-12-0
21 (12
11 12
Again (y/n)? y
Enter the first fraction (eg. 3/4 4/7
Enter the second fraction (3/4): 1/2
24/7
₂ 1/2
0
0
<< (r1== r2) << endl;
<< (r1 < x2) << endl;
<< (r1 <= r2) << endl;
<< (r1 r2) << endl;
<< (r1 >= r2) << endl << endl;
> 12
12-1
Again (y/n)? a
COCON LARGE
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
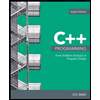
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
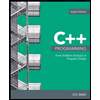
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage